Question
Objectives For this programming project, you will develop a library management program that maintains the library book list and the library client (i.e., customer) list,
Objectives
For this programming project, you will develop a library management program that maintains the library book list and the library client (i.e., customer) list, as well as the interactions between the two lists. The program allows the librarian to add copies of books, add clients, let a client check out (i.e., borrow) books, and let a client check in (i.e., return) books.
Problem Description
In this programming assignment, you will develop a project that implements an engineering library (or any other library; engineering library is mentioned because all the books I currently include are engineering textbooks). You will implement and test four classes in this assignment. They are the BookType, BookRecord, ClientType, and LibraryType classes. You are to write a implementation file: ClientType.cpp. In the following, we will discuss each class in more detail.
The ClientType Class
Each object of the ClientType class contains the related information of a library client. The ClientType.h file is given to you. Carefully read the class definition in ClientType.h. The ClientType class has five protected data members. They are: a string ID7 to store the 7-digit ID number of a client; a string lastName to store the clients last name; a string firstName to store the clients first name; an integer key to indicate the key member used for searching and sorting clients; a sorted linked list bookList of BookType objects to store the books borrowed by the client.
You are to implement and test the following operations for the ClientType class. Detailed description and precondition/postcondition for all the ClientTypes member functions are specified in the given header file.
A constructor.
Five set functions: setID7, setLastName, setFirstName, setKey, setBookList.
A setClientInfo function.
Five get functions: getID7, getLastName, getFirstName, getKey, getBookList.
A borrowBook function.
A returnBook function.
The six comparison operators.
The assignment operator=.
The operator << (as a friend function).
This is the .h file:
//**********************************************************
// SPECIFICATION FILE (ClientType.h)
// This class specifies the basic members to implement a
// library client.
//**********************************************************
#ifndef CLIENTTYPE_H
#define CLIENTTYPE_H
#include "SortedLinkedList.h"
#include "BookType.h"
#include
#include
using namespace std;
// Forward declarations
class ClientType;
ostream& operator <<(ostream& outs, const ClientType& client);
class ClientType
{
public:
// Constructor
ClientType(string s1 = "", string s2 = "", string s3 = "",
int k = 1);
// The member variables are set according to the incoming
// parameters. If no values are specified, the default values
// are assigned. (By default, we set key=1, i.e., we set the ID7
// as the key member since it is different for different clients;
// so it is also used in the role of primary key for a list of clients.)
// Post: ID7= s1; lastName = s2; firstName = s3; key = k; and bookList
// is initialized to be an empty linked list (by default).
// Action responsibilities
void setID7(string s);
// Function to set the ID7 of a client.
// Post: ID7 = s;
void setLastName(string s);
// Function to set the last name of a client.
// Post: lastName = s;
void setFirstName(string s);
// Function to set the first name of a client.
// Post: firstName = s;
void setKey(int k);
// Function to set the key of a client. (When key==1, set ID7 as
// the key member; when key==2, set lastName as the key; we do not use
// firstName as the key member.)
// Post: key = k;
void setBookList(const SortedLinkedList
// Function to set the list of currently borrowed books of a client.
// Post: bookList = books (by deep copy using the overloaded operator=
// in the LinkedListType class).
void setClientInfo(string s1, string s2, string s3,
const SortedLinkedList
// Function to set the member variables according to the incoming
// parameters. (By default, we set key=1, i.e., we set the ID7
// as the key member.)
// Post: ID7 = s1; lastName = s2; firstName = s3;
// bookList = books; key = k;
// Knowledge responsibilities
string getID7() const;
// Function to check the client's ID7.
// Post: The value of ID7 is returned.
string getLastName() const;
// Function to check the client's last name.
// Post: The value of lastName is returned.
string getFirstName() const;
// Function to check the client's first name.
// Post: The value of firstName is returned.
int getKey() const;
// Function to check the client's key.
// Post: The value of key is returned.
SortedLinkedList
// Function to check the client's list of currently borrowed
// books. This function requires the default and copy constructors
// for SortedLinkedList class to be written (they have already been
// added to the SortedLinkedList class declaration and definition).
// Post: A deep copy of the linked list of bookList is returned.
// Other functions
void borrowBook(const BookType& book);
// Function to check out a book. This function calls the insert function
// of the SortedLinkedList class. Hence, no duplicate copies of the book
// can be borrowed.
// Post: If book is already in client's bookList or the bookList is full, an
// an appropriate message is displayed. Otherwise, book is inserted
// to the bookList and the list items are in ascending order
// (according to ISBN13)
void returnBook(const BookType& book);
// Function to check in a book. This function calls the remove function
// of the SortedLinkedList class.
// Post: If book is found in bookList, the node containing item is
// deleted from the bookList.
// Overloaded relational operators
bool operator==(const ClientType& otherClient) const;
// Overloaded operator==
// Post: Return 1 if and only the two clients' ID7 are the same;
// otherwise, return 0.
bool operator!=(const ClientType& otherClient) const;
// Overloaded operator!=
// Post: Return 1 if and only the two clients' ID7 are different;
// otherwise, return 0.
bool operator<(const ClientType& otherClient) const;
// Overloaded operator<
// Post: When key==1, return 1 if and only ID7 // otherwise, return 0. // When key!=1 (i.e., key==2), return 1 if, // (a) lastName of current client < // lastName of otherClient // OR // (b) lastName of current client == // lastName of otherClient // and firstName of current client < // firstName of otherClient; // OR // (c) lastName of current client == // lastName of otherClient // and firstName of current client == // firstName of otherClient // and ID7 // otherwise, return 0. bool operator<=(const ClientType& otherClient) const; // Overloaded operator<= // Post: When key==1, return 1 if and only ID7 // otherwise, return 0. // When key!=1 (i.e., key==2), return 1 if, // (a) lastName of current client < // lastName of otherClient // OR // (b) lastName of current client == // lastName of otherClient // and firstName of current client < // firstName of otherClient; // OR // (c) lastName of current client == // lastName of otherClient // and firstName of current client == // firstName of otherClient // and ID7<=otherBook.ID7; // otherwise, return 0. bool operator>(const ClientType& otherClient) const; // Overloaded operator> // Post: When key==1, return 1 if and only ID7>otherClient.ID7; // otherwise, return 0. // When key!=1 (i.e., key==2), return 1 if, // (a) lastName of current client > // lastName of otherClient // OR // (b) lastName of current client == // lastName of otherClient // and firstName of current client > // firstName of otherClient; // OR // (c) lastName of current client == // lastName of otherClient // and firstName of current client == // firstName of otherClient // and ID7>otherBook.ID7; // otherwise, return 0. bool operator>=(const ClientType& otherClient) const; // Overloaded operator>= // Post: When key==1, return 1 if and only ID7>otherClient.ID7; // otherwise, return 0. // When key!=1 (i.e., key==2), return 1 if, // (a) lastName of current client > // lastName of otherClient // OR // (b) lastName of current client == // lastName of otherClient // and firstName of current client > // firstName of otherClient; // OR // (c) lastName of current client == // lastName of otherClient // and firstName of current client == // firstName of otherClient // and ID7>=otherBook.ID7; // otherwise, return 0. // Overloaded operator = const ClientType& operator=(const ClientType& otherClient); // Function to overload the assignment operator for deep copy. // Friend function friend ostream& operator<<(ostream& outs, const ClientType& client); // Overloaded operator << for the ClientType class. (In order to overload // operator << for the ClientType class, we have added a templated version of // overloaded operator << for the LinkedListType class.) // Post: The client information is written to the input ostream instance. // The return value is the input ostream instance. The format of output // is as follows: // (First leave one empty line) // Client ID: (Display the client's ID7 in this line.) // Client Name: (Display the client's last name, followed by ", " and // first name in this line.) // Books borrowed by the client (ordered by ISBN-13): (Display in this line.) // (Now call outs << client.bookList to list the book.) // (Finally dispaly a line with "******************************") protected: string ID7; // variable to store a 7-digit ID, using string rather than // int allows for the leading 0's to show up in the 7 digits string lastName; // variable to store the client's last name string firstName; // variable to store the client's first name int key; // to indicate the key member used for searching and sorting. // When key==1, set ID7 as the key member; when key==2, set // lastName as the key. SortedLinkedList // the books currently borrowed by the client. We use BookType value // for the info of each node since we assume that for every book, a // client can check out at most one copy. }; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
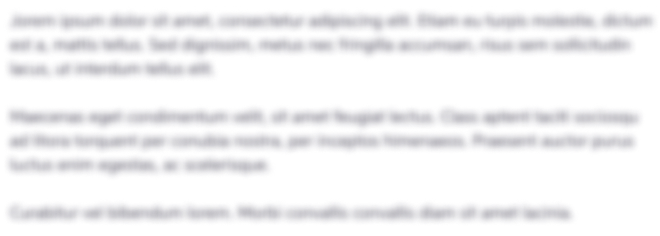
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started