Question
Objectives The objective of this project is to learn how create a class on your own and how to test code with the unit testing
Objectives
The objective of this project is to learn how create a class on your own and how to test code with the unit testing framework JUnit.
Background
The project contains one class Project3Test.
The project3Test class represents a test suite for a class RoachPopulation.java that you will be writing.
The Project3Test class is a test suite that is used to test some aspects of the RoachPopulation class. It does this by creating a RoachPopulation object and printing the results of calling some of their methods.
In class, we went over BankAccount class and a driver class BankAccountTest for testing our BankAccount class (also check Die class under Activity 7 - Classes). Using the main method to test your class design relies on a human to read the output and to compare it to the expected output to determine if the code is working correctly. The problem with this testing method is that the human has to carefully check the output, and needs to do so every time the code is changed, compiled and run. What we would like to have is a more automated way of testing our code.
Unit testing provides a way of automatically testing individual classes and methods in an automated and repeatable way. By writing tests that test the behavior of classes and methods and comparing them automatically to expected values, we can let the computer run through our suite of tests every time we make a change to the code. This way we can be sure that any changes we made did not break some code that was already working. In many large software projects the test suite is run automatically every time a developer pushes new code to repository.
We will be using JUnit, a unit testing framework written specifically for Java. JUnit can be run on its own, or as a plug-in to integrated development environments like Eclipse. JUnit is already built in to BlueJ.
Objectives
This project provides you with the opportunity to put together nearly all of the material you have learned so far to create a single program. Most of what you are asked to do is very similar to what you did in the labs and in-class work, so look back at those labs and class examples for ideas.
You will be writing code for one class - RoachPopulation.
Specifications
In this programming project, you will write a simple program to do the following.
Implement a class RoachPopulation that simulates the growth of a roach population. The class has the following methods:
- The constructor takes the size of the initial roach population (an int).
- The breed method simulates a period in which the roaches breed, which doubles their population. This method has no arguments and no return value.
- The spray method simulates spraying with insecticide, which reduces the population by the given percentage. This method takes a percentage as a double value and has no return value
- The getRoaches method returns the current number of roaches.
- The toString method returns a String. For example, if the current number of roaches in the population is 50, this method will return Number of roaches in the population: 50
- You need to write javadoc comments for all the methods in the RoachPopulation class. Please refer to your class examples, POGIL activity worksheets and Zybooks for more information and ask questions on Piazza.
Procedure
A program Project3Test is already provided for you. To use this code in your Bluej package, do the following:
- Create a folder Project3Code within the Project3 folder
- Create empty RoachPopulation class.
-
Create empty RoachPopulationTest class by doing the following steps.
- Right-click on the box for the RoachPopulation class you just created, and choose Create Test Class as shown here. A new box will appear (colored green) called RoachPopulationTest, and with the annotation <
> as shown here - Double-click the RoachPopulationTest class to view the source code.
- The RoachPopulationTest class imports some code from org.junit, and sets up three methods a constructor, a setUp method and a tearDown method. You do not need to understand this code to use the tests.
- Replace the entire RoachPopulationTest.java with the contents of Project3Test.java file. You do not need to understand this code to use the tests.
- Right-click on the box for the RoachPopulation class you just created, and choose Create Test Class as shown here. A new box will appear (colored green) called RoachPopulationTest, and with the annotation <
-
To run the tests, use the Run Tests button on the left panel. If it is not visible as shown here then click on the arrow to make it visible as shown here. Compile your code and run the tests by clicking Run Tests. This should pass because you have an empty class and an empty test class. You should get in the habit of writing and testing a single method before moving on to the next.
-
Write your constructor for the RoachPopulation class, and then test it by uncommenting the setup method in RoachPopulation, compiling both classes, and running the tests. If your test passes, move on to the next method. If your test fails, make corrections to your constructor until the test passes.
-
Continue this process for each of the remaining methods and their associated tests:
- getRoaches and test_getRoaches
- toString and test_toString()
- breed and test_breed
- spray and test_spray
- test_breedAndSpray
This is an Individual Assignment - No Partners
As this is a Project (and not a Lab) you will be working on your own, not with a partner. You should not be sharing your code with anyone else, other than the instructor.
You will need to fork your own private Project3 repository on GitLab for this project. The only person who should have any access to your repository is your instructor.
You can ask questions on Piazza about setting up your repository on GitLab, about using Git to send code to the instructor, and general questions about how to write your code. However you should not be posting sections of code and asking others to find your errors.
Deliverables
You need to submit your Project3 folder with the subfolder Project3Code with RoachPopulation.java and RoachPopulationTest.java files.
Be sure that you have your name and an explanation of what your program does in the Javadoc comments. Be sure that you have indented consistently.
The instructor will pull your Project3 from your GitLab repository to grade it. Make sure:
- You have pushed all changes to your shared repository. (We cant access local changes on your computer.)
- You have added your instructor as Master to your shared GitLab repository.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
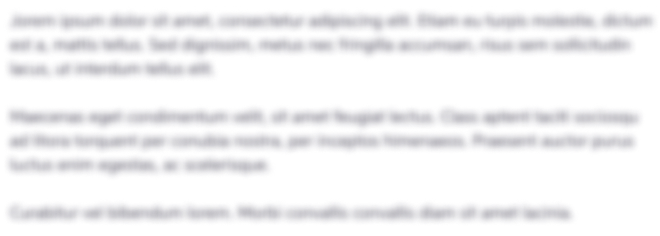
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started