Question
Objectives The objectives of this lab are to: Understand how numbers are stored in computers and the precision of those numbers Practice good documenting and
Objectives
The objectives of this lab are to:
Understand how numbers are stored in computers and the precision of those numbers
Practice good documenting and programming style
Practice writing a lab memo
Estimate Machine Epsilon and Digits of Precision
Estimate the single and double precision machine epsilons using the equation
EM = 2-(n)
In this equation, EM is machine epsilon, and n is the number of bits in the mantissa of the stored number (only
actual bits, not including the shadow bit).
Also, estimate the digits of precision based on each machine epsilon using the equation
Nd = -log10(EM)
Write Your C++ Program
Write a C++ program that will allow you to experimentally determine machine epsilon on your computer.
Initially declare your variables to be of type float. The program should prompt the user for a small value.
Store that value as dx. Then, add dx to 1.0 and store the result as x. Finally, print out the values of x and dx
using the following code:
// Set formatting for cout:
cout.setf(ios::fixed); // Fixed point (not scientific)
cout.setf(ios::showpoint); // Print trailing zeros
cout.precision(22); // Number of digits after decimal
cout << x << << dx << endl;
This section of code tells cout to print using fixed points rather than scientific notation, to print any trailing
zeros, and finally to always print 22 digits after the decimal. These commands make sure that when you print
out x and dx, youll be able to see exactly what is stored in each. Once your code is working, add code that
allows the user to run the program again without exiting and restarting the program. One way to do this is by
reading in the value of an integer flag. If the user enters 1 for the flag, your program should terminate. If the
flag is set to anything else, your program should read in a new value for dx, execute the addition again (and
print the result again), and read in the integer flag again. Your program should repeat this process until the
user enters a 1 for the integer flag.
Compile and Run Your Program to Find Machine Epsilon
Starting with a value of dx = 0.001, run your program with smaller and smaller values of dx (e.g. 1e-4, 1e-5,
etc.) until the precision of the computer is exceeded (i.e., x + dx = x because x + dx contains too
many digits of precision). Continue experimenting until you find machine epsilon (EM) to at least three
significant figures. Note that, because of rounding, EM will be twice as large as the largest value of dx which,
when added to 1, results in 1. Another way to tell when youve found EM is that, if dx is between EM and half
of EM, the result of 1 + dx will be equal to 1 + EM.
Modify and Re-Run Your Program
Change the declarations of x and dx to doubles. Find the double precision machine epsilon.
Report Your Findings
Write a memo, using the Suggested Format of Lab Memo Reports, which includes:
a) Your results, including your experimental machine epsilon, estimated machine epsilon, and digits of
precision for both floats and doubles.
b) A comparison of the experimental and estimated machine epsilons.
c) Answers to the following questions:
1. If single precision were used, what would the estimated round-off error be for a nominal value of
50.5? (You should not re-run your program to find this.)
2. What is machine epsilon? (Answer in words and describe machine epsilon; it is not the smallest
number the computer can store.)
3. Why does round-off error increase as the nominal value increases?
d) Your source code and your sample input/output that shows how you found machine epsilon.
C++ Program Format
Throughout the semester, use the following format for your C++ programs.
Following the header, the program statements are in the following order:
pre-processor directives (such as #include
main program body
variable declarations
program statements
other functions (Include a header which explains the function)
Your programs should also employ good style. This includes appropriate indentation, spacing, and
comments. Any user prompts should be simple and descriptive.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
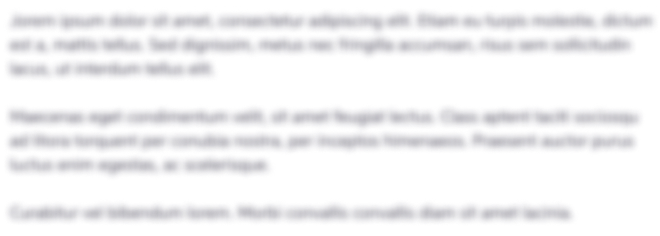
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started