Question
Objectives Write methods and constructors Use parameters to provide methods with input Return values from methods Query / Modify object state with methods Specifications Getting
Objectives
Write methods and constructors
Use parameters to provide methods with input
Return values from methods
Query / Modify object state with methods
Specifications
Getting started
Download the HW3Starter.zip starter project from Moodle and import it into Eclipse.
Overview
In this homework you will write constructors and methods for a SnackMachine class that emulates a device where you can put some money in, select multiple items (gum, candy, and/or chips, in any quantity), and complete your order, receiving change if appropriate. The SnackMachine class in the starter project has the following instance variables and their getter methods:
gumPrice: the cost of one pack of gum
candyPrice: the cost of one bag of candy
chipsPrice: the cost of one bag of chips
gumInOrder: the total number of packs of gum currently in your order
candyInOrder: the total number of bags of candy currently in your order
chipsInOrder: the total number of bags of chips currently in your order
totalSales: the total sales (in dollars) sold by the machine (not just for the current order, but cumulative over all orders)
paymentTendered: the amount of money the user has put into the machine
Write the constructor
The constructor should have three parameters for the gum, candy, and chip prices (in that order).
The body of the constructor should set ALL of the instance variables. If an instance variable does not have an associated parameter then it should be set to zero.
Test by uncommenting all the code in the TestConstructor class and running it as a JUnit test. Fix any errors you find.
Refactor the constructor
Refactor a public changePrices() method from the constructor. This method will change the prices of the gum, candy, and chips, in that order.
Test the changePrices() method by uncommenting the code in the TestChangePrices class and running it as a JUnit test. Fix any errors that that you find.
Refactor a private helper method to reset the gumInOrder, candyInOrder, chipsInOrder, and paymentTendered instance variables to zero. Call this method resetOrder.
Refactor a private helper to reset totalSales to zero. Call this method resetMachine.
Re-run all tests and make sure you haven't introduced any new bugs.
Adding money
Write a putInMoney method that adds money to the current order (i.e., the paymentTendered) variable.
Uncomment the code in TestPutInMoney and run the JUnit tests. Fix any errors. If you have syntax errors, you have probably named your method incorrectly, or do not have the right number or type(s) of parameters. Rethink the needs of your method.
Adding items to the order
Write an addGumToOrder method that adds one or more packs of gum to the order.
Uncomment the code in TestAddGumToOrder and run the JUnit tests. Fix any errors.
Repeat steps 1 and 2 for addCandyToOrder and addChipsToOrder.
Calculating the total for the order
Write a getOrderTotal method that returns the total cost of the order, based on the price and quantity of each item.
Uncomment the code in TestGetOrderTotal and run the JUnit tests. Fix any errors.
Completing an order
Write a completeOrder method that "completes the order", i.e.,
figures out how much change (if any) is needed
increases the total sales by the order total
resets the order
returns the change
Uncomment the code in TestCompleteOrder and run the JUnit tests. Fix any errors.
Export the project
Export the project to a ZIP file FirstnameLastnameHW3.zip.
Upload the ZIP file to your Moodle account.
END OF EXERCISE
Submission notes
When uploading your file to Moodle, verify that you are uploading the correct one and verify the zip file is named FirstnameLastnameHW3 and submit the zip file in Moodle by the due date.
If you do not follow the specified naming convention, three points will be deducted from the score you would have received.
Grading breakdown
10 pts. Exercise completed successfully with at most only a minor error.
7 pts. Exercise is missing some functionality.
4 pts. Exercise is missing most of the major functionality.
0 pts. Exercise not submitted, does not compile, or barely started.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
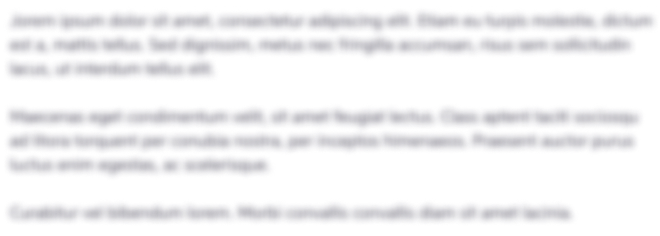
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started