Question
Odd Jobs You have a friend who does odd jobs and wants you to write a program that will help her keep track of her
Odd Jobs
You have a friend who does odd jobs and wants you to write a program that will help her keep track of her customers and their orders.
NOTE: By now you should know that your constructors should not accept parameters that are invalid or out of range, and should have a way of handling that situation so that instance variables have valid values at all times.
Step One
Create a class called Job. Job has instance variables for
- Customer name (String)
- Job description (String)
- Job start date (Date)
- Job completion date (Date)
- Job price (double)
- Job amount paid (double)
Make a default constructor and a constructor that takes parameters for customer name, job description, job start date, and job price (in that order). The price should be non-negative; enforce this, but it is not generally advisable for a constructor to throw an exception. Job completion date is initially null and amount paid is initially 0.
NOTE: When modifying any of the Date instance variables, dont simply assign them, clone them.
The Job class should have the methods listed below. For those with parameters, the method should throw an appropriate exception, with an appropriate message, if its parameter cannot be accepted. Your exception messages should be meaningful and specific.
- completeJob(endDate) sets the job completion date. The completion date should be accepted (remember to clone) only if it is no earlier than the job start date, and no later than today.
- makePayment(amount) makes a payment on the job. The payment is accepted only if it is non-negative.
- addToPrice(amount) adds amount (which can be negative) to price. Not accepted if the new price ends up being negative.
Add other methods as needed. NOTE: it is not the job of this class to handle exceptions. Its job is to throw exceptions when necessary and pass them to the caller to be handled.
Step Two
Write a class called OddJobs that has a main program. Your main program has two modes. It can run in interactive mode or in data import mode. If the program is run with no command line arguments, it runs in interactive mode. To run the program in data import mode, it should be invoked from the command line like this java OddJobs di filename. If a user runs your program with command line arguments, your program must verify that the parameters are in the correct format; if not a usage message appears.
Begin by coding a main program that processes the command line arguments and then either calls a method called interactiveMode (that does nothing) or a method called importMode (that does nothing). Only after this basic framework is working should you proceed to the next step.
Step Three
Code the interactiveMode method. In this mode, your program begins by opening a file called oddjobs.txt. This file contains information about all of the Jobs, which should be read in from the file and stored in an ArrayList of Jobs. If the file named oddjobs.txt does not exist, your program creates it. This file is expected to have the following format and you can assume the format of this file is correct. For each job, there will be six lines of information, which contain the values for the 6 instance variables described above. If the job end date is null, there will be blank line for that value. For example, suppose there are 2 Job, then oddjobs.txt might have contents like this (the first job has a null completion date):
Wile E. Coyote Fake tunnel construction 2/20/2020
2999 1000 Bugs Bunny Enlargement of hole in the ground 1/3/2020 2/1/2020 700 700
|
After reading in the file (and closing it!) your program runs a menu that allow the user to do the following:
- Quit the program
- Add a new job (ask the user for customer name, job description, job start date, and price)
- Make a payment on a job (ask the user for the customer name and the amount of the payment)
- Complete a job (ask the user for the customer name and the completion date)
- Add to the price of a job (ask the user for the customer name and the amount of the change)
- Display to the screen all the information on all the jobs
Your main program catches and reports all exceptions without terminating. After the user chooses option 0, your program writes all the Jobs to the file named oddjobs.txt.
Step Four
Code the importMode method. In this mode, your program has received a parameter which is a filename. The filename must not be named oddjobs.txt. You can assume that the file has the same format as oddjobs.txt. In this mode, your program opens the indicated file and appends all the data in it to oddjobs.txt. If an error occurs, your program handles the error without crashing (reminder: you can assume input files are formatted correctly). If no error occurs, the program reports a count of how many records were successfully imported and then terminates.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
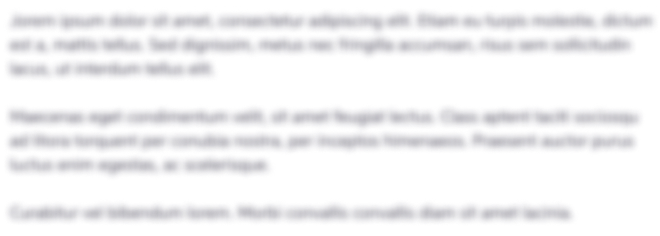
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started