Answered step by step
Verified Expert Solution
Question
1 Approved Answer
ok I went through many help and got to the point that I can interact with the game but is there a way to interact
ok I went through many help and got to the point that I can interact with the game but is there a way to interact with the grid and then code checks and gives solution if its right or wrong?
import java.util.Scanner;
public class Sudoku
public static void mainString args
Create a Sudoku object
Sudoku obj new Sudoku;
Initial Sudoku puzzle represents empty cells
int b
;
Display the initial Sudoku grid
int size blength;
System.out.printlnThe grid is:;
obj.displayb size;
Allow the player to input numbers into the Sudoku grid
obj.playSudokub size;
Attempt to solve the Sudoku puzzle and display the solution
if objsolveSudokub size
System.out.printlnSudoku solved:";
obj.displayb size;
else
System.out.printlnNo solution exists";
Method to allow the player to play Sudoku
public void playSudokuint b int num
Scanner scanner new ScannerSystemin;
while true
System.out.printlnEnter row and column separated by space to place a number to num or enter to finish:";
int row scanner.nextInt;
int col scanner.nextInt;
Check for the termination condition
if row col
break; Player wants to finish
Validate the input for row and column
if row row num col col num
System.out.printlnInvalid input. Row and column must be between and num ;
continue;
Get the number to be placed
System.out.printlnEnter a number to num :;
int number scanner.nextInt;
Validate the input number
if number number num
System.out.printlnInvalid number. Enter a number between and num ;
continue;
Check if the move is safe
if isSafeb row, col, number
System.out.printlnInvalid move. The number cannot be placed at this position.";
continue;
Place the number and display the updated grid
browcol number;
System.out.printlnNumber placed successfully!";
displayb num;
Method to check if placing a number at a particular position is safe
public boolean isSafeint b int row, int col, int number
Check row and column
for int i ; i blength; i
if browi number bicol number
return false;
Check x subgrid
int sqrt int Math.sqrtblength;
int boxRowStart row row sqrt;
int boxColStart col col sqrt;
for int r boxRowStart; r boxRowStart sqrt; r
for int d boxColStart; d boxColStart sqrt; d
if brd number
return false;
return true;
Method to solve the Sudoku puzzle using backtracking
public boolean solveSudokuint b int num
int row ;
int col ;
boolean isEmpty true;
Find the first empty cell
for int i ; i num; i
for int j ; j num; j
if bij
row i;
col j;
isEmpty false;
break;
if isEmpty
break;
If there is no empty cell, then the puzzle is solved
if isEmpty
return true;
Try placing numbers in the empty cell
for int number ; number num; number
if isSafeb row, col, number
browcol number;
Recursively solve the rest of the grid
if solveSudokub num
return true;
else
browcol; Backtrack
return false; No solution found
Method to display the Sudoku grid
Step by Step Solution
There are 3 Steps involved in it
Step: 1
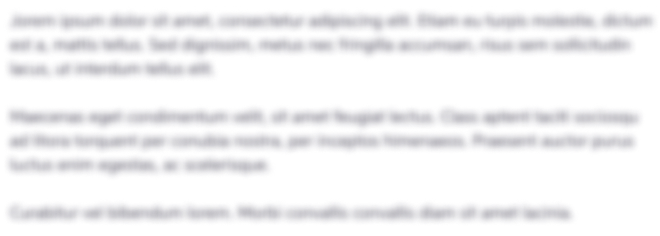
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started