Okay so this is what I have so far:
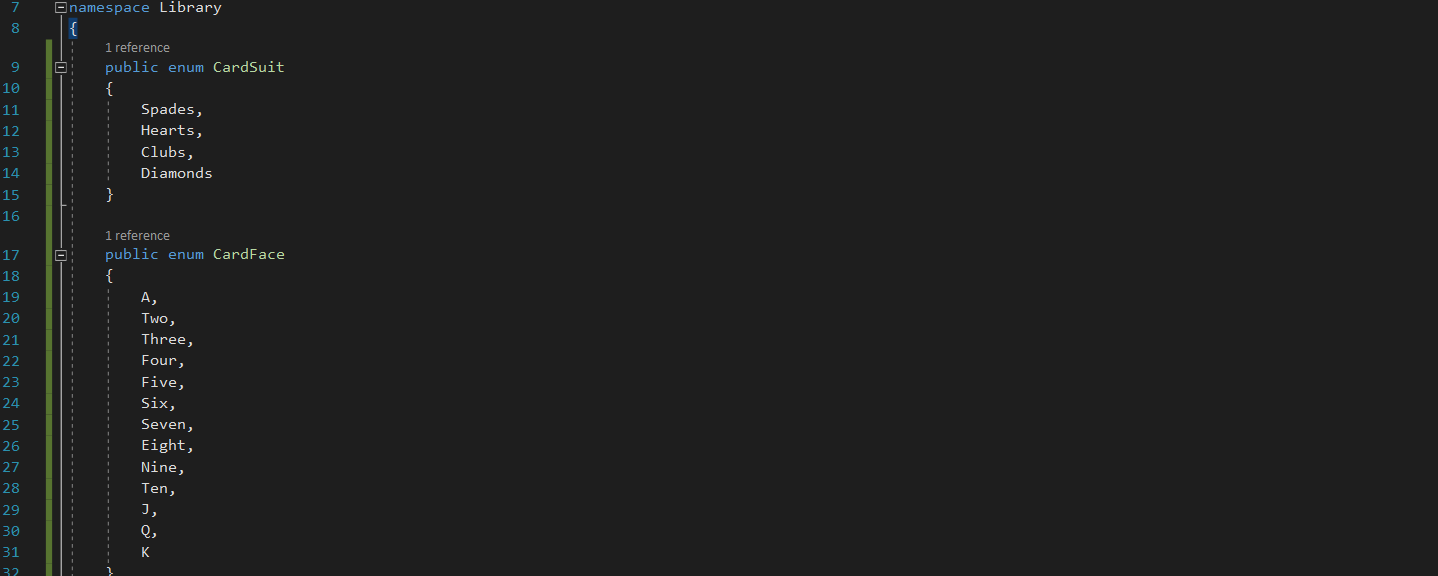
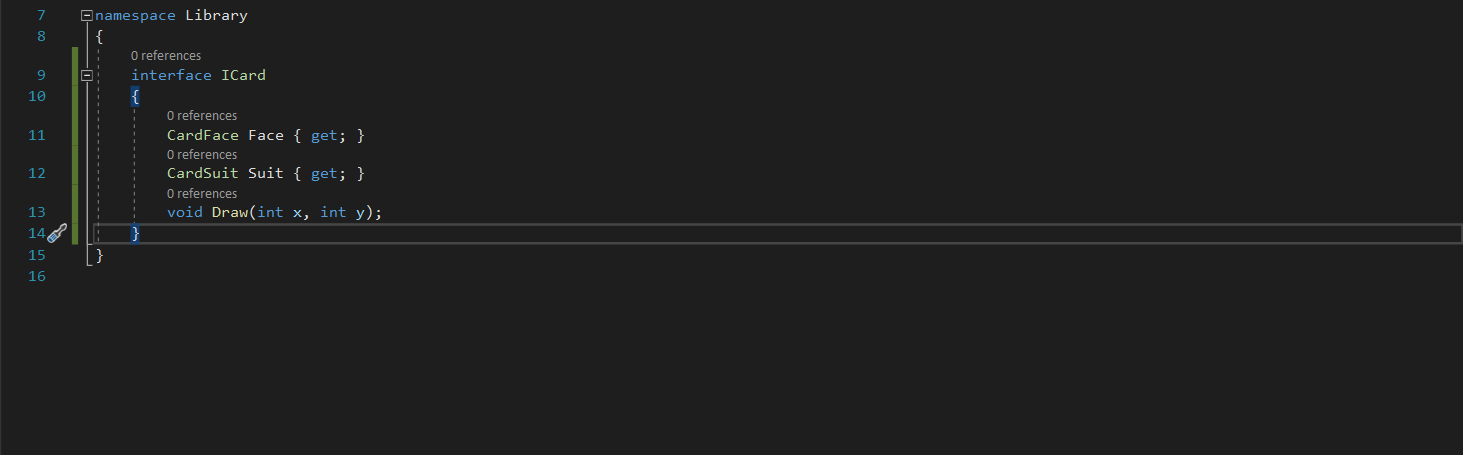
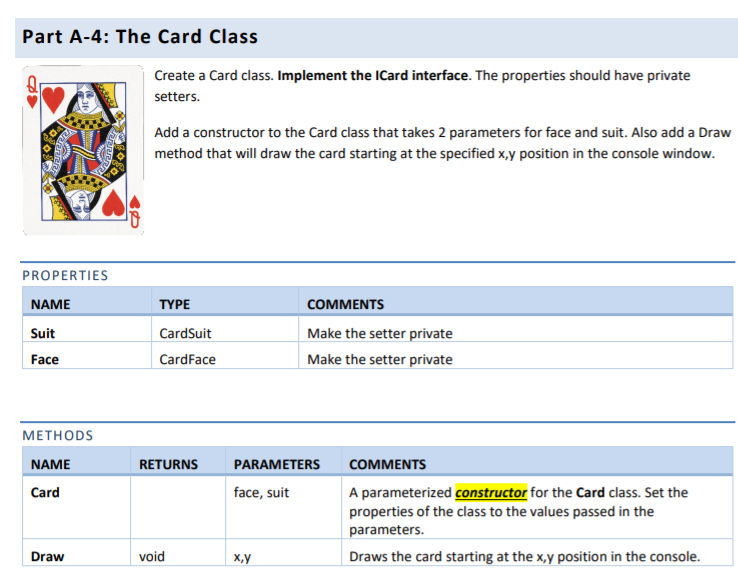
Part A-5: The Card Factory class:
Create a static factory class that will have a static method for creating cards.

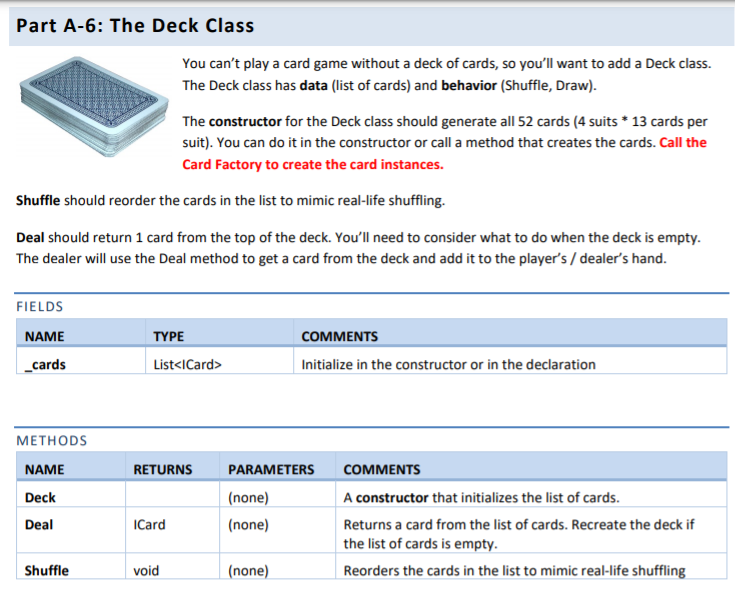
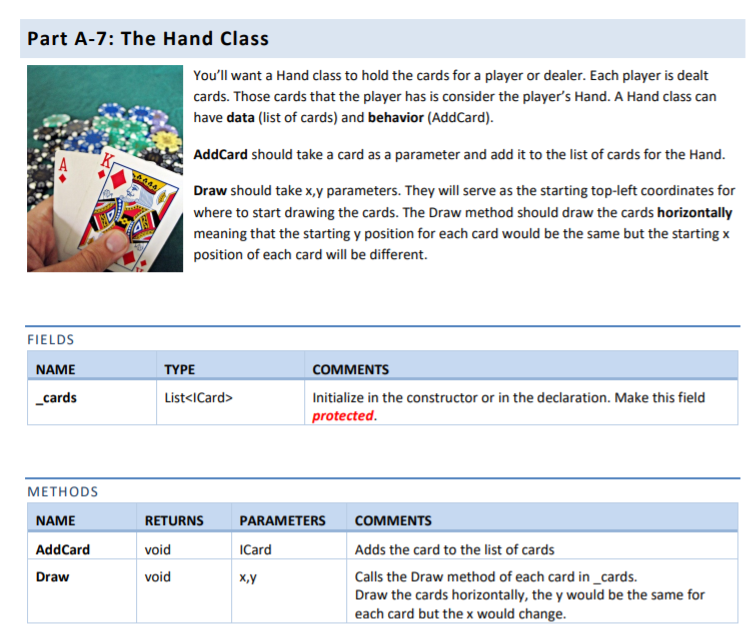
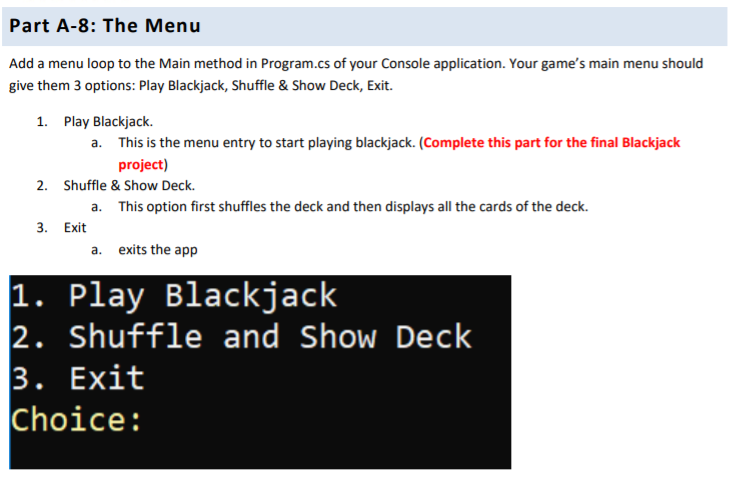
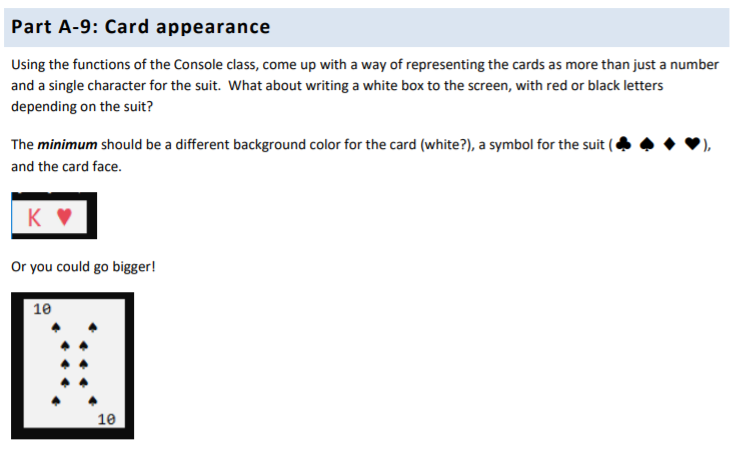
Onamespace Library 8 1 reference public enum CardSuit 9 10 11 12 13 14 15 16 Spades, Hearts, Clubs, Diamonds 17 18 19 20 21 22 23 24 25 26 27 28 29 30 1 reference public enum CardFace { A, Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, J, Q, K 31 32 7 8 namespace Library { O references interface ICard 9 10 11 O references CardFace Face { get; } O references CardSuit Suit { get; } O references void Draw(int x, int y); 12 13 14 15 } 16 Part A-4: The Card Class Create a Card class. Implement the ICard interface. The properties should have private setters. Add a constructor to the Card class that takes 2 parameters for face and suit. Also add a Draw method that will draw the card starting at the specified x,y position in the console window. PROPERTIES NAME TYPE COMMENTS Suit Face CardSuit CardFace Make the setter private Make the setter private METHODS RETURNS NAME Card PARAMETERS face, suit COMMENTS A parameterized constructor for the Card class. Set the properties of the class to the values passed in the parameters. Draws the card starting at the x,y position in the console. Draw void RETURNS NAME CreateCard PARAMETERS face, suit iCard COMMENTS A static method that creates a Card instance using the parameters and returns it. Part A-6: The Deck Class You can't play a card game without a deck of cards, so you'll want to add a Deck class. The Deck class has data (list of cards) and behavior (Shuffle, Draw). The constructor for the Deck class should generate all 52 cards (4 suits * 13 cards per suit). You can do it in the constructor or call a method that creates the cards. Call the Card Factory to create the card instances. Shuffle should reorder the cards in the list to mimic real-life shuffling. Deal should return 1 card from the top of the deck. You'll need to consider what to do when the deck is empty. The dealer will use the Deal method to get a card from the deck and add it to the player's / dealer's hand. FIELDS NAME _cards TYPE List
COMMENTS Initialize in the constructor or in the declaration METHODS NAME RETURNS PARAMETERS Deck Deal (none) (none) ICard COMMENTS A constructor that initializes the list of cards. Returns a card from the list of cards. Recreate the deck if the list of cards is empty. Reorders the cards in the list to mimic real-life shuffling Shuffle void (none) Part A-7: The Hand Class You'll want a Hand class to hold the cards for a player or dealer. Each player is dealt cards. Those cards that the player has is consider the player's Hand. A Hand class can have data (list of cards) and behavior (AddCard). AddCard should take a card as a parameter and add it to the list of cards for the Hand. A444 Al Draw should take x,y parameters. They will serve as the starting top-left coordinates for where to start drawing the cards. The Draw method should draw the cards horizontally meaning that the starting y position for each card would be the same but the starting x position of each card will be different. FIELDS NAME _cards TYPE List COMMENTS Initialize in the constructor or in the declaration. Make this field protected. RETURNS PARAMETERS METHODS NAME AddCard Draw ICard void void COMMENTS Adds the card to the list of cards Calls the Draw method of each card in _cards. Draw the cards horizontally, the y would be the same for each card but the x would change. X,Y Part A-8: The Menu Add a menu loop to the Main method in Program.cs of your Console application. Your game's main menu should give them 3 options: Play Blackjack, Shuffle & Show Deck, Exit. 1. Play Blackjack. a. This is the menu entry to start playing blackjack. (Complete this part for the final Blackjack project) 2. Shuffle & Show Deck. a. This option first shuffles the deck and then displays all the cards of the deck. 3. Exit a. exits the app 1. Play Blackjack 2. Shuffle and Show Deck 3. Exit Choice: Part A-9: Card appearance Using the functions of the Console class, come up with a way of representing the cards as more than just a number and a single character for the suit. What about writing a white box to the screen, with red or black letters depending on the suit? The minimum should be a different background color for the card (white?), a symbol for the suit and the card face. Or you could go bigger! 10 10 Onamespace Library 8 1 reference public enum CardSuit 9 10 11 12 13 14 15 16 Spades, Hearts, Clubs, Diamonds 17 18 19 20 21 22 23 24 25 26 27 28 29 30 1 reference public enum CardFace { A, Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, J, Q, K 31 32 7 8 namespace Library { O references interface ICard 9 10 11 O references CardFace Face { get; } O references CardSuit Suit { get; } O references void Draw(int x, int y); 12 13 14 15 } 16 Part A-4: The Card Class Create a Card class. Implement the ICard interface. The properties should have private setters. Add a constructor to the Card class that takes 2 parameters for face and suit. Also add a Draw method that will draw the card starting at the specified x,y position in the console window. PROPERTIES NAME TYPE COMMENTS Suit Face CardSuit CardFace Make the setter private Make the setter private METHODS RETURNS NAME Card PARAMETERS face, suit COMMENTS A parameterized constructor for the Card class. Set the properties of the class to the values passed in the parameters. Draws the card starting at the x,y position in the console. Draw void RETURNS NAME CreateCard PARAMETERS face, suit iCard COMMENTS A static method that creates a Card instance using the parameters and returns it. Part A-6: The Deck Class You can't play a card game without a deck of cards, so you'll want to add a Deck class. The Deck class has data (list of cards) and behavior (Shuffle, Draw). The constructor for the Deck class should generate all 52 cards (4 suits * 13 cards per suit). You can do it in the constructor or call a method that creates the cards. Call the Card Factory to create the card instances. Shuffle should reorder the cards in the list to mimic real-life shuffling. Deal should return 1 card from the top of the deck. You'll need to consider what to do when the deck is empty. The dealer will use the Deal method to get a card from the deck and add it to the player's / dealer's hand. FIELDS NAME _cards TYPE List COMMENTS Initialize in the constructor or in the declaration METHODS NAME RETURNS PARAMETERS Deck Deal (none) (none) ICard COMMENTS A constructor that initializes the list of cards. Returns a card from the list of cards. Recreate the deck if the list of cards is empty. Reorders the cards in the list to mimic real-life shuffling Shuffle void (none) Part A-7: The Hand Class You'll want a Hand class to hold the cards for a player or dealer. Each player is dealt cards. Those cards that the player has is consider the player's Hand. A Hand class can have data (list of cards) and behavior (AddCard). AddCard should take a card as a parameter and add it to the list of cards for the Hand. A444 Al Draw should take x,y parameters. They will serve as the starting top-left coordinates for where to start drawing the cards. The Draw method should draw the cards horizontally meaning that the starting y position for each card would be the same but the starting x position of each card will be different. FIELDS NAME _cards TYPE List COMMENTS Initialize in the constructor or in the declaration. Make this field protected. RETURNS PARAMETERS METHODS NAME AddCard Draw ICard void void COMMENTS Adds the card to the list of cards Calls the Draw method of each card in _cards. Draw the cards horizontally, the y would be the same for each card but the x would change. X,Y Part A-8: The Menu Add a menu loop to the Main method in Program.cs of your Console application. Your game's main menu should give them 3 options: Play Blackjack, Shuffle & Show Deck, Exit. 1. Play Blackjack. a. This is the menu entry to start playing blackjack. (Complete this part for the final Blackjack project) 2. Shuffle & Show Deck. a. This option first shuffles the deck and then displays all the cards of the deck. 3. Exit a. exits the app 1. Play Blackjack 2. Shuffle and Show Deck 3. Exit Choice: Part A-9: Card appearance Using the functions of the Console class, come up with a way of representing the cards as more than just a number and a single character for the suit. What about writing a white box to the screen, with red or black letters depending on the suit? The minimum should be a different background color for the card (white?), a symbol for the suit and the card face. Or you could go bigger! 10 10