Question
Old Maid is a game that requires 51 cards, that is to say the traditional 52 cards minus the jack of clubs card. Each player
Old Maid is a game that requires 51 cards, that is to say the traditional 52 cards minus the jack of clubs card. Each player takes a card from his left neighbour,
without seeing the card. Then all pairs are discarded. A pair is two cards with the same value. You have to get rid of all your cards to win. The player who ends up with the Old Maid player, that is to say the jack of clubs, will loose.To simplify the game even more, we will have only two players: the computer (i.e., your program)
and a user (you). We will call the computer Robot and the user Human. Robot will give the cards for each game.Part of the game is already provided in the a3Part2.py file. You can study it and test it. Thenyou must add your code in the same file, in the places indicated by comments, in the functions
that have only "docstring" comments and partial code or no code.
It is not allowed to modify the functions that are already completed, nor the main program. Youcan only change functions that are not completed. You can add other functions if you wish. The functions make_deck, wait_for_player and shuffle_deck are already fully coded for you.
You must complete the other functions: deal_cards, remove_pairs, display_cards, get_valid_input, and play. These functions must respect the descriptions for the data type and the result type (the "docstring" comments). Do not modify their header (name and number of parameters).
Most of your code should go into the play function, which should call the other functions (i.e.,deal_cards, remove_pairs, display_cards, and get_valid_input).
For the get_valid_input function, we assume that Human will enter an integer value, but check in the function if it is a valid integer (between 1 and the number of cards). The function should ask Human which card he wants, among the Robot cards that Human cannot see. When Robot takes a Human card, the card must be chosen randomly (you can use the random function). If Human asks, for example the 3rd card, it means the position/index element 2 among Robot's cards (because it
is a list).
Suggestions:
• Study the parts of the code already completed to understand them.
• Think about the parts of the game that remain to be completed. For example, the game must show
to Human these cards, be able to ask Human which card he wants, remove the card pairs for Robot
and for Human, etc.
• Complete the functions one at a time, to avoid too many errors at the same time. You can test
each function before completing the others, in the Python interpreter. For example, you can test
the remove_pairs function with test cases like this: (the order of elements in the result could be
different):
>>> remove_pairs (['10♣', '2♣', '5♢', '6♣', '9♣', 'A♢', '10♢']):
['2♣', '5♢', '6♣', '9♣', 'A♢']
• The game takes turns between Robot and Human. Think about how you can represent each round
(who to play). One possibility is to use a variable that is zero when it is Robot's turn and 1 when it
is Human's turn. You will also need to identify when the game ends and who has won.
# Card game called "Old Maid"
# The computer is the card dealer.
# A card is a 2 character string.
# The first character represents a value and the second a color.
# Values are characters like '2','3','4','5','6','7','8','9','10','J','Q' ,'K', and 'A'.
# Colors are characters like: ♠, ♡, ♣, and ♢.
# We use 4 Unicode symbols to represent the 4 suits: spades, hearts, clubs and diamonds.
# For cards of 10 we use 3 characters, because the value '10' uses two characters.
a3Part2.py file
import random
def wait_for_player():
'''()->None
Pause the program until the user presses Enter
'''
try:
input("Press Enter to continue. ")
except SyntaxError:
pass
def make_deck():
'''()->list of str
Returns a list of strings representing all cards,
except the black jack.
'''
deck=[]
colors = ['u2660', 'u2661', 'u2662', 'u2663']
values = ['2','3','4','5','6','7','8','9','10','J','Q','K','A']
for val in values:
for color in colors:
deck.append(val+color)
deck.remove('Ju2663') # eliminates the black jack (the jack of clubs)
return deck
def shuffle_deck (p):
'''(list of str)->None
Shuffles the list of character strings representing the deck of cards
'''
random.shuffle(p)
def deal_cards(p):
'''(list of str)-> tuple of (list of str,list of str)
Return two lists that represent the two hands of the cards.
The dealer gives one card to the other player, one to himself,
and it continues until the end of packet p.
'''
dealer=[]
other=[]
# COMPLETE THIS FUNCTION IN ACCORDANCE WITH THE DESCRIPTION ABOVE
# ADD YOUR CODE HERE
return (dealer, other)
def remove_pairs(l):
'''
(list of str)->list of str
Returns a copy of list l with all pairs eliminated
and mix the remaining elements.
Test:
(Note that the order of the elements in the result could be different)
>>> remove_pairs(['9♠', '5♠', 'K♢', 'A♣', 'K♣', 'K♡', '2♠', 'Q♠', 'K♠', 'Q♢', 'J♠', 'A♡', '4♣', '5♣', '7♡', 'A♠', '10♣', 'Q♡', '8♡', '9♢', '10♢', 'J♡', '10♡', 'J♣', '3♡'])
['10♣', '2♠', '3♡', '4♣', '7♡', '8♡', 'A♣', 'J♣', 'Q♢']
>>> remove_pairs(['10♣', '2♣', '5♢', '6♣', '9♣', 'A♢', '10♢'])
['2♣', '5♢', '6♣', '9♣', 'A♢']
'''
result=[]
# COMPLETE THIS FUNCTION IN ACCORDANCE WITH THE DESCRIPTION ABOVE
# ADD YOUR CODE HERE
random.shuffle(result)
return result
def display_cards(p):
'''
(list)-None
Displays the elements of the list p separated by spaces
'''
# COMPLETE THIS FUNCTION IN ACCORDANCE WITH THE DESCRIPTION ABOVE
# ADD YOUR CODE HERE
def get_valid_input(n):
'''
(int)->int
Returns an integer from the keyboard, from 1 to n (1 and n inclusive).
Keep asking if the user enters an integer that is not in the range [1,n]
Précondition: n>=1
'''
# COMPLETE THIS FUNCTION IN ACCORDANCE WITH THE DESCRIPTION ABOVE
# ADD YOUR CODE HERE
def play():
'''()->None
This function plays the game'''
p=make_deck()
shuffle_deck(p)
tmp=deal_cards(p)
dealer=tmp[0]
human=tmp[1]
print("Hello. My name is Robot and I'm dealing the cards.")
print("Your hand is:")
display_cards(human)
print("Don't worry, I can't see your cards or their order.")
print("Now discard all pairs from your hand. I'll do it too.")
wait_for_player()
dealer=remove_pairs(dealer)
human=remove_pairs(human)
# COMPLETE THIS FUNCTION IN ACCORDANCE WITH THE DESCRIPTION ABOVE
# ADD YOUR CODE HERE
# Main program
play()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
import random def waitforplayer None Pause the program until the user presses Enter try inputPress Enter to continue except SyntaxError pass def maked...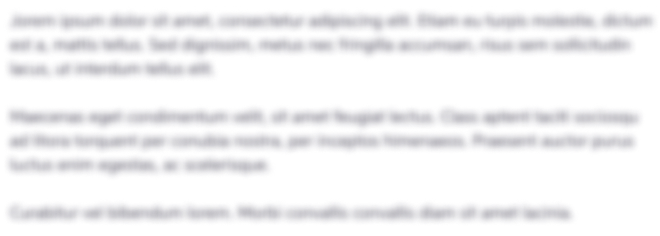
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started