Question
On the last part of the project, you'll add searching and sorting functionalities to the MovieApp. MovieApp.java contains all the methods you need to implement.
On the last part of the project, you'll add searching and sorting functionalities to the MovieApp. MovieApp.java contains all the methods you need to implement. Refer to the methods description on file for your implementation. Fill in the code in the following methods and submit MovieApp.java:
searchMovieByName: search a movie by name using the iterative binary search algorithm. Assume a sorted array.
searchMovieByName: search a movie by name recursively. Assume an unsorted array.
sortByYear: sort the array of movies by year using the insertion sort algorithm.
sortByName: sorts the array of movies by name using the selection sort algorithm.
We provide the files here:
IO.java: the IO class
Movie.java: the Movie class from the first part of the project
MovieApp.java: the application file with the methods from the second part of the project and the methods you are to update and submit
MovieDriver.java: a driver to test your MovieApp
Observe the following rules:
DO NOT add any import statements to MovieApp.java
DO NOT change the headers of ANY of the given methods
DO NOT change/remove any of the given class fields
DO NOT add any new class fields
DO NOT use System.exit()
DO NOT use the IO module in MovieApp.java
YOU MAY add new helper methods, but you must declare them private
If you wish to change MovieDriver, feel free. You are not submitting it.
Movie :
/*
* The Movie class
*
* @author runb-cs111
*/
public class Movie implements Comparable {
private String name; // the movie name
private String director; // the director's name
private int year; // movie release year
private String description; // movie short description
private int ratings; // rating from 1 (didn't like) to 5 (loved it)
/*
/*
* Returns this movie name
* @return String the movie name
*/
public String getName () {
return name;
}
/*
* Updates the director's name.
* @param director is the new director's name
*/
public void setDirector ( String director ) {
this.director = director;
}
* Constructor initializes the new Movie object.
* @param name This is the movie name
*/
public Movie ( String name ) {
setName ( name );
}
/*
* Updates the movie's name.
* @param name is the new name
*/
public void setName ( String name ) {
this.name = name;
}
/*
* Returns the director's name
* @return String the director's name
*/
public String getDirector () {
return director;
}
/*
* Updates the movie's description
* @param description is the new short description
*/
public void setDescription ( String description ) {
this.description = description;
}
/*
* Return the movie's description
* @return String the short movie's description
*/
public String getDescription () {
return description;
}
/*
* Updates the release year
* @param year is the new year
*/
public void setYear ( int year ) {
this.year = year;
}
/*
* Returns the year the movie was released
* @return int the year
*/
public int getYear () {
return year;
}
/*
* Updates the movie's ratings
* @param ratings is the new rating
*/
public void setRatings ( int ratings ) {
this.ratings = ratings;
}
/*
* Returns the movie rating
* @return int the rating
*/
public int getRatings () {
return ratings;
}
/*
* Returns the string representation of the movie.
* @return String the string representation
*/
public String toString () {
return String.format("%s - %d [Rate: %d] ", name, year, ratings);
}
/*
* Check is two movies have the same name
* @return boolean true if other'name is the same as this.name, or false if they don't
*/
public boolean equals ( Object other ) {
if ( other == null || !(other instanceof Movie) ) {
return false;
}
Movie o = (Movie) other;
return this.name.equals(o.name) && this.year == o.year && this.director.equals(o.director);
}
/*
* Compares this movie name against @other lexicographically.
* @return int positive number if s1 > s2, negative number if s1 < s2, zero if s1 == s2
*/
public int compareTo (Movie other) {
return this.name.compareTo(other.name);
}
}
MovieApp :
import java.util.Arrays;
/*
*
* This class implements a library of movies
*
* @author runb-cs111
*
*/
public class MovieApp {
private Movie[] items; // keep movies in an unsorted array
private int numberOfItems; // number of movies in the array
/*
* Default constructor allocates array with capacity for 20 movies
*/
MovieApp() {
/** COMPLETE THIS METHOD **/
items = new Movie[20];
numberOfItems = 0;
}
/*
* One argument constructor allocates array with user defined capacity
*
* @param capacity defines the capacity of the movie library
*/
MovieApp(int capacity) {
/** COMPLETE THIS METHOD **/
items = new Movie[capacity];
numberOfItems = 0;
}
/*
* Add a movie into the library (unsorted array)
*
* Inserts @m into the first empty spot in the array. If the array is full
* (there is no space to add another movie) - create a new array with double
* the size of the current array - copy all current movies into new array -
* add new movie
*
* @param m The movie to be added to the libary
*/
public void addMovie(Movie m) {
/** COMPLETE THIS METHOD **/
if (numberOfItems < items.length) {
items[numberOfItems++] = m;
} else {
items = Arrays.copyOf(items, items.length * 2);
items[numberOfItems++] = m;
}
}
/*
* Removes a movie from the library. Returns true if movie is removed, false
* otherwise. The array should NOT become sparse after a remove, that is,
* all movies should be in consecutive array indices.
*
* @param m The movie to be removed
*
* @return Returns true is movie is successfuly removed, false otherwise
*/
public boolean removeMovie(Movie m) {
/** COMPLETE THIS METHOD **/
// THE FOLLOWING LINE IS A PLACEHOLDER TO MAKE THIS METHOD COMPILE
// Change as needed for your implementation
for (int i = 0; i < numberOfItems; i++) {
if (items[i].equals(m)) {
if (i == numberOfItems - 1)
numberOfItems--;
else {
for (int j = i; j < numberOfItems - 1; j++) {
items[j] = items[j + 1];
}
numberOfItems--;
}
return true;
}
}
return false;
}
/*
* Returns the library of movies
*
* @return The array of movies
*/
public Movie[] getMovies() {
/** COMPLETE THIS METHOD **/
// THE FOLLOWING LINE IS A PLACEHOLDER TO MAKE THIS METHOD COMPILE
// Change as needed for your implementation
return items;
}
/*
* Returns the current number of movies in the library
*
* @return The number of items in the array
*/
public int getNumberOfItems() {
/** COMPLETE THIS METHOD **/
// THE FOLLOWING LINE IS A PLACEHOLDER TO MAKE THIS METHOD COMPILE
// Changed as needed for your implementation
return numberOfItems;
}
/*
* Update the rating of movie @m to @ratings
*
* @param @m The movie to have its ratings updated
*
* @param @ratings The new ratings of @m
*
* @return tue if update is successfull, false otherwise
*/
public boolean updateRating(Movie m, int ratings) {
/** COMPLETE THIS METHOD **/
// THE FOLLOWING LINE IS A PLACEHOLDER TO MAKE THIS METHOD COMPILE
// Changed as needed for your implementation
for (int i = 0; i < numberOfItems; i++) {
if (items[i].equals(m)) {
items[i].setRatings(ratings);
return true;
}
}
return false;
}
/*
* Prints all movies
*/
public void print() {
/** COMPLETE THIS METHOD **/
for (int i = 0; i < numberOfItems; i++)
System.out.println(items[i]);
}
/*
* Return all the movies by @director. The array size should be the number
* of movies by @director.
*
* @param director The director's name
*
* @return An array of all the movies by @director
*/
public Movie[] getMoviesByDirector(String director) {
/** COMPLETE THIS METHOD **/
// THE FOLLOWING LINE IS A PLACEHOLDER TO MAKE THIS METHOD COMPILE
// Changed as needed for your implementation
Movie[] list = new Movie[0];
int index = 0;
for (int i = 0; i < numberOfItems; i++) {
if (items[i].getDirector().equalsIgnoreCase(director)) {
list = Arrays.copyOf(list, index + 1);
list[index++] = items[i];
}
}
return list;
}
/*
* Returns all the movies made in @year. The array size should be the number
* of movies made in @year.
*
* @param year The year the movies were made
*
* @return An array of all the movies made in @year
*/
public Movie[] getMoviesByYear(int year) {
/** COMPLETE THIS METHOD **/
// THE FOLLOWING LINE IS A PLACEHOLDER TO MAKE THIS METHOD COMPILE
// Changed as needed for your implementation
Movie[] list = new Movie[0];
int index = 0;
for (int i = 0; i < numberOfItems; i++) {
if (items[i].getYear() == year) {
list = Arrays.copyOf(list, index + 1);
list[index++] = items[i];
}
}
return list;
}
/*
* Returns all the movies with ratings greater then @ratings. The array size
* should match the number of movies with ratings greater than @ratings
*
* @param ratings
*
* @return An array of all movies with ratings greater than @ratings
*/
public Movie[] getMoviesWithRatingsGreaterThan(int ratings) {
/** COMPLETE THIS METHOD **/
// THE FOLLOWING LINE IS A PLACEHOLDER TO MAKE THIS METHOD COMPILE
// Changed as needed for your implementation
Movie[] list = new Movie[0];
int index = 0;
for (int i = 0; i < numberOfItems; i++) {
if (items[i].getRatings() > ratings) {
list = Arrays.copyOf(list, index + 1);
list[index++] = items[i];
}
}
return list;
}
}
MovieDriver :
/**
* Rutgers, The State University of New Jersey
* CS 111 Movie Driver
* Joseph A. Boyle (joseph.a.boyle@rutgers.edu)
* April 4, 2018
*/
class MovieDriver{
public static void main(String[] args){
MovieApp app = new MovieApp();
printSeparator();
System.out.println("Created a Movie app with the default constructor.");
System.out.println("Would you like to load in the first 20 movies? (Y for yes or n for no)");
if(IO.readBoolean()) loadAllMovies(app, getSampleMovies1());
System.out.println("Would you like to load in the last of the movies? (Y for yes or n for no)");
if(IO.readBoolean()) loadAllMovies(app, getSampleMovies2());
printSeparator();
printSeparator();
printSeparator();
System.out.println("You have loaded a total of: " + app.getNumberOfItems() + " movies.");
System.out.println("Now entering user interactivity mode.");
printSeparator();
printSeparator();
userActivityMode(app);
}
public static void userActivityMode(MovieApp app){
String operation = "";
while(!operation.equals("exit")){
printSeparator();
System.out.println("Possible Commands:");
System.out.println("\tprint:\t\tprint all of the movies.");
System.out.println("\tdirector:\tget all movies by a director.");
System.out.println("\tyear:\t\tget all movies by a year.");
System.out.println("\trating:\t\tget all movies about a certain rating.");
System.out.println("\tremove:\t\tRemoves a movie at a certain index.");
System.out.println("\tupdate:\t\tUpdate the ratings of a movie.");
System.out.println("\texit:\t\tstop the program.");
System.out.println("");
System.out.println("What would you like to do? (use the commands above. For example, type print to print all movies): ");
System.out.println("You may type exit or press Control C to stop this program.");
operation = IO.readString();
switch(operation){
case "exit":
return;
case "print":
appPrint(app);
break;
case "director":
appDirector(app);
break;
case "remove":
appRemove(app);
break;
case "update":
appUpdate(app);
break;
case "year":
appYear(app);
break;
case "rating":
appRating(app);
break;
default:
System.out.println("Unknown command: " + operation);
break;
}
System.out.println("Please press enter to continue.");
IO.readString(); // we ignore whatever is entered, we just was confirmation before continuing.
printSeparator();
}
}
public static void appPrint(MovieApp app){
System.out.println("Printing all of the movies stored in the app.");
app.print();
}
public static void appDirector(MovieApp app){
System.out.println("Which director would you like to search by?");
String director = IO.readString();
Movie[] byDirector = app.getMoviesByDirector(director);
System.out.println("Found " + byDirector.length + " movies by director " + director + ":");
for(Movie m : byDirector){
System.out.println(m.toString());
}
}
public static void appYear(MovieApp app){
System.out.println("Which Year would you like to search by?");
int year = IO.readInt();
Movie[] byYear = app.getMoviesByYear(year);
System.out.println("Found " + byYear.length + " movies in year " + year + ":");
for(Movie m : byYear){
System.out.println(m.toString());
}
}
public static void appRating(MovieApp app){
System.out.println("What is the minimum rating would you like to search by?");
int rating = IO.readInt();
Movie[] byRating = app.getMoviesWithRatingsGreaterThan(rating);
System.out.println("Found " + byRating.length + " movies with a rating at least " + rating + ":");
for(Movie m : byRating){
System.out.println(m.toString());
}
}
public static void appUpdate(MovieApp app){
System.out.println("Here are all of the movies with their numbers:");
Movie[] movies = app.getMovies();
for(int i = 0; i < app.getNumberOfItems(); i ++){
System.out.println("[" + i + "]: " + movies[i].toString());
}
System.out.println("Please enter the number of the movie you'd like to update");
int i = IO.readInt();
if(i < 0 || i >= app.getNumberOfItems()){
System.out.println("i out of bounds.");
return;
}
System.out.println("Updating movie " + i + ": " + movies[i].toString());
System.out.println("Enter the new rating for the movie [1 through 5]");
int newRating = IO.readInt();
app.updateRating(movies[i], newRating);
}
public static void appRemove(MovieApp app){
System.out.println("Here are all of the movies with their numbers:");
Movie[] movies = app.getMovies();
for(int i = 0; i < app.getNumberOfItems(); i ++){
System.out.println("[" + i + "]: " + movies[i].toString());
}
System.out.println("Please enter the number of the movie you'd like to remove");
int i = IO.readInt();
if(i < 0 || i >= app.getNumberOfItems()){
System.out.println("i out of bounds.");
return;
}
System.out.println("Removing movie " + i + ": " + movies[i].toString());
app.removeMovie(movies[i]);
}
public static void printSeparator(){
System.out.println("====================================================");
}
public static void loadAllMovies(MovieApp app, Movie[] movies){
for(int i = 0; i < movies.length; i ++){
System.out.println("Loading Movie #" + (i + 1) + ": " + movies[i].toString());
app.addMovie(movies[i]);
}
}
public static Movie[] getSampleMovies2(){
int numMovies = 6;
String[] names = {"Patton", "Braveheart", "Jurassic Park", "The Exorcist", "The Grapes of Wrath", "The Green Mile"};
String[] directors = {"Franklin J. Schaffner", "Mel Gibson", "Steven Spielberg", "William Friedkin", "John Ford", "Frank Darabont"};
int[] years = {1970, 1995, 1993, 1973, 1940, 1999};
int[] ratings = {1, 4, 5, 3, 1, 5};
String[] descriptions = {"It's all about WWII", "Freeeeeedoooooom", "Dinosaurs make a Comeback", "Quite Scary", "Some awfuly angry Grapes", "I'd be Wrong to not include a Steven King book"};
Movie[] movies = new Movie[numMovies];
for(int i = 0; i < numMovies; i ++){
Movie m = new Movie(names[i]);
m.setDirector(directors[i]);
m.setYear(years[i]);
m.setRatings(ratings[i]);
m.setDescription(descriptions[i]);
movies[i] = m;
}
return movies;
}
// A bunch of movies, 20 in total.
// These should be loaded first.
public static Movie[] getSampleMovies1(){
int numMovies = 20;
String[] names = {"The Shawshank Redemption","Schindler's List", "The Wizard of Oz", "One Flew Over the Cuckoo's Nest", "Forrest Gump", "The Sound of Music", "West Side Story", "Star Wars IV", "2001: A Space Odyssey", "E.T", "The Silence of the Lambs", "It's a Wonderful Life", "The Lord of The Rings: Return of the Kind", "Gladiator", "Titanic", "Saving Private Ryan", "Raiders of the Lost Arc", "Rocky I", "To Kill a Mockingbird", "Jaws"};
String[] directors = {"Frank Darabont", "Steven Spielberg", "Victor Flemming", "Milos Forman", "Robert Zemeckis", "Robert Wise", "Robert Wise", "George Lucas", "Stanley Kubrik", "Steven Spielberg", "Jonathan Demme", "Frank Capra", "Peter Jackson", "Ridley Scott", "James Cameron", "Steven Spielberg", "Steven Spielberg", "John G. Avildsen", "Robert Mulligan", "Steven Spielberg"};
int[] years = {1994, 1993, 1939, 1975, 1994, 1965, 1961, 1977, 1968, 1982, 1992, 1946, 2003, 2000, 1997, 1998, 1981, 1976, 1962, 1975};
int[] ratings = {5, 5, 4, 5, 4, 5, 3, 4, 3, 2, 3, 1, 1, 5, 3, 4, 2, 5, 2, 4};
String[] descriptions = {"Prison Break", "It's a classic", "Follow the Road", "Don't mess with Big Nurse", "Bubba Gump Shrimp", "Do re me fa so la te do", "Not to be confused with the musical", "Use the Force, Luke", "Ground Control to Major Tom...", "E.T. Phone Home", "Surprisingly not about butterflies", "What a Wonderful World", "You're chosen, Bilbo Baggins", "A Film about Vengence", "Talk about Sinking Ships", "No man left behind", "Don't forget your hat, Indiana", "Win, Rocky!", "Two birds, one stone", "Sharks, in New Jersey"};
Movie[] movies = new Movie[numMovies];
for(int i = 0; i < numMovies; i ++){
Movie m = new Movie(names[i]);
m.setDirector(directors[i]);
m.setYear(years[i]);
m.setRatings(ratings[i]);
m.setDescription(descriptions[i]);
movies[i] = m;
}
return movies;
}
}
IO class :
import java.io.*;
public class IO
{
private static BufferedReader kb =
new BufferedReader(new InputStreamReader(System.in));
private static BufferedReader fio = null;
public static boolean openFile(String filename){
try{
fio = new BufferedReader(new FileReader(filename));
return true;
}catch (IOException e){ return false;}
}
public static String readLine(){
if (fio == null)
return null;
try{
return fio.readLine();
}catch(IOException e){ return null;}
}
public static String readString()
{
while (true) {
try {
return kb.readLine();
} catch (IOException e) {
// should never happen
}
}
}
public static int readInt()
{
while (true) {
try {
String s = kb.readLine();
return Integer.parseInt(s);
} catch (NumberFormatException e) {
System.out.print("That is not an integer. Enter again: ");
} catch (IOException e) {
// should never happen
}
}
}
public static double readDouble()
{
while (true) {
try {
String s = kb.readLine();
return Double.parseDouble(s);
} catch (NumberFormatException e) {
System.out.print("That is not a number. Enter again: ");
} catch (IOException e) {
// should never happen
}
}
}
public static char readChar()
{
String s = null;
try {
s = kb.readLine();
} catch (IOException e) {
// should never happen
}
while (s.length() != 1) {
System.out.print("That is not a single character. Enter again: ");
try {
s = kb.readLine();
} catch (IOException e) {
// should never happen
}
}
return s.charAt(0);
}
public static boolean readBoolean()
{
String s = null;
while (true) {
try {
s = kb.readLine();
} catch (IOException e) {
// should never happen
}
if (s.equalsIgnoreCase("yes") ||
s.equalsIgnoreCase("y") ||
s.equalsIgnoreCase("true") ||
s.equalsIgnoreCase("t")) {
return true;
} else if (s.equalsIgnoreCase("no") ||
s.equalsIgnoreCase("n") ||
s.equalsIgnoreCase("false") ||
s.equalsIgnoreCase("f")) {
return false;
} else {
System.out.print("Enter \"yes\" or \"no\": ");
}
}
}
public static void outputStringAnswer(String s)
{
if (s != null) {
System.out.println("RESULT: \"" + s + "\"");
} else {
System.out.println("RESULT: null");
}
}
public static void outputIntAnswer(int i)
{
System.out.println("RESULT: " + i);
}
public static void outputDoubleAnswer(double d)
{
System.out.println("RESULT: " + d);
}
public static void outputCharAnswer(char c)
{
System.out.println("RESULT: '" + c + "'");
}
public static void outputBooleanAnswer(boolean b)
{
System.out.println("RESULT: " + b);
}
public static void reportBadInput()
{
System.out.println("User entered bad input.");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
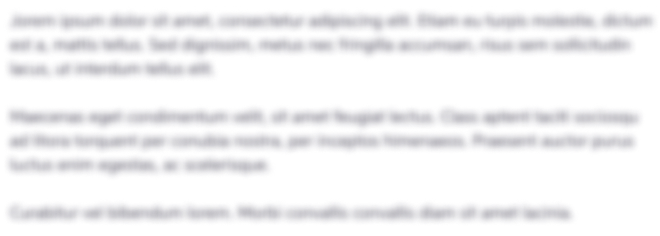
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started