Answered step by step
Verified Expert Solution
Question
1 Approved Answer
One at a time, remove an entry from this.bagOfStrings . If the entry is a vowel, place it in this.bagOfVowels ; otherwise, discard the entry,
One at a time, remove an entry from this.bagOfStrings. If the entry is a vowel, place it in this.bagOfVowels; otherwise, discard the entry, report the number of vowels in the this.bagOfVowels and the number of times each vowel appears in the bag.
public class ResizableArrayBag implements BagInterface { private T[] bag; // Cannot be final due to doubling private int numberOfEntries; private boolean initialized = false; private static final int DEFAULT_CAPACITY = 25; // Initial capacity of bag private static final int MAX_CAPACITY = 10000; /** * Creates an empty bag whose initial capacity is 25. */ public ResizableArrayBag() { this(DEFAULT_CAPACITY); } // end default constructor /** * Throws an exception if the client requests a capacity that is too large. */ private void checkCapacity(int capacity) { if (capacity > MAX_CAPACITY) throw new IllegalStateException("Attempt to create a bag whose capacity exceeds " + "allowed maximum of " + MAX_CAPACITY); } // end checkCapacity /** * Creates an empty bag having a given initial capacity. * * @param initialCapacity The integer capacity desired. */ public ResizableArrayBag(int initialCapacity) { checkCapacity(initialCapacity); // The cast is safe because the new array contains null entries @SuppressWarnings("unchecked") T[] tempBag = (T[]) new Object[initialCapacity]; // Unchecked cast this.bag = tempBag; this.numberOfEntries = 0; this.initialized = true; } // end constructor /** * Creates a bag containing given entries. * * @param contents An array of objects. */ public ResizableArrayBag(T[] contents) { checkCapacity(contents.length); this.bag = Arrays.copyOf(contents, contents.length); this.numberOfEntries = contents.length; this.initialized = true; } // end constructor /** * Throws an exception if receiving object is not initialized. */ private void checkInitialization() { if (!this.initialized) throw new SecurityException("Uninitialized object used " + "to call an ArrayBag method."); } // end checkInitialization /** * Returns true if the array bag is full, or false if not. */ private boolean isArrayFull() { return this.numberOfEntries >= this.bag.length; } // end isArrayFull /** * Doubles the size of the array bag. * Precondition: checkInitialization has been called. */ private void doubleCapacity() { int newLength = 2 * this.bag.length; checkCapacity(newLength); this.bag = Arrays.copyOf(this.bag, newLength); } // end doubleCapacity /** * Adds a new entry to this bag. * * @param newEntry The object to be added as a new entry. * @return True. */ public boolean add(T newEntry) { checkInitialization(); if (isArrayFull()) { doubleCapacity(); } // end if this.bag[this.numberOfEntries] = newEntry; this.numberOfEntries++; return true; } // end add /** * Gets the current number of entries in this bag. * * @return The integer number of entries currently in this bag. */ public int getCurrentSize() { return this.numberOfEntries; } // end getCurrentSize /** * Sees whether this bag is empty. * * @return True if this bag is empty, or false if not. */ public boolean isEmpty() { return this.numberOfEntries == 0; } // end isEmpty /** * Removes and returns the entry at a given index within the array. * If no such entry exists, returns null. * Precondition: 0 = 0)) { result = this.bag[givenIndex]; // Entry to remove int lastIndex = this.numberOfEntries - 1; this.bag[givenIndex] = this.bag[lastIndex]; // Replace entry to remove with last entry this.bag[lastIndex] = null; // Remove reference to last entry this.numberOfEntries--; } // end if return result; } // end removeEntry /** * Removes one unspecified entry from this bag, if possible. * * @return Either the removed entry, if the removal * was successful, or null. */ public T remove() { checkInitialization(); T result = removeEntry(this.numberOfEntries - 1); return result; } // end remove /** * Removes one occurrence of a given entry from this bag. * * @param anEntry The entry to be removed. * @return True if the removal was successful, or false if not. */ public boolean remove(T anEntry) { checkInitialization(); int index = getIndexOf(anEntry); T result = removeEntry(index); return anEntry.equals(result); } // end remove /** * Removes all entries from this bag. */ public void clear() { while (!isEmpty()) remove(); } // end clear /** * Counts the number of times a given entry appears in this bag. * * @param anEntry The entry to be counted. * @return The number of times anEntry appears in this ba. */ public int getFrequencyOf(T anEntry) { checkInitialization(); int counter = 0; for (int index = 0; index -1, anEntry is in the array bag, and it // equals bag[where]; otherwise, anEntry is not in the array. return where; } // end getIndexOf /** * Tests whether this bag contains a given entry. * * @param anEntry The entry to locate. * @return True if this bag contains anEntry, or false otherwise. */ public boolean contains(T anEntry) { checkInitialization(); return getIndexOf(anEntry) > -1; // or >= 0 } // end contains /** * Retrieves all entries that are in this bag. * * @return A newly allocated array of all the entries in this bag. */ public T[] toArray() { checkInitialization(); // The cast is safe because the new array contains null entries. @SuppressWarnings("unchecked") T[] result = (T[]) new Object[this.numberOfEntries]; // Unchecked cast for (int index = 0; indexpublic class WorkingWithBags { // instance variables should be created/initialized by the constructor private BagInterface bagOfStrings; private BagInterface bagOfVowels; // constants should be initialized at the definition level final BagInterface ALL_VOWELS = new ResizableArrayBag(new String[]{"a", "e", "i", "o", "u"}); final int MAX_NUMBER_OF_STRINGS_TO_GENERATE = 41; final int MIN_NUMBER_OF_STRINGS_TO_GENERATE = 35; final int MAX_STRING_LENGTH = 3; final int MIN_STRING_LENGTH = 1; /** * constructor creates this.bagOfStrings and this.bagOfVowels objects */ public WorkingWithBags() { // TODO Done this.bagOfStrings = new ResizableArrayBag(); this.bagOfVowels = new ResizableArrayBag(); } // default constructor /** * Adds the given entry to this.bagOfStrings if valid * * throws InvalidParameterException exception if the length of the string is outside the given bounds * throws InvalidParameterException exception if the string contains characters other than lowercase letters */ public void addToBagOfStrings(String newEntry) throws InvalidParameterException { // TODO Needs work } /** * Generates randomly string objects and adds them to this.bagOfStrings */ public void generateStrings() { System.out.println("*** Generating strings ***"); final int SEED = 41; final int ASCII_LOWERCASE_A = 97; final int ASCII_LOWERCASE_Z = 122; Random random = new Random(SEED); // using a seed to help with testing - the output should match exactly the sample run provided // TODO needs work // randomly generate an integer between MIN_NUMBER_OF_STRINGS_TO_GENERATE and MAX_NUMBER_OF_STRINGS_TO_GENERATE inclusive // representing the number of String objects to be generated // generate the String objects consisting of letters in lower case of randomly generated // length between MIN_STRING_LENGTH and MAX_STRING_LENGTH inclusive // call addToBagOfStrings to add the objects to the bag of strings }// end generateStrings /** * Removes the given entry from this.bagOfStrings and if successful creates and adds the replacement to the bag * The replacement is the given string in reverse * * @return returns String in reverse or null */ public String replaceGivenEntryWithItsReverse(String entryToReplace) { String reverse = null; // TODO // no need to call contains - call remove and check its returned value return reverse; } // replaceGivenEntryWithItsReverse /** * Removes all entries from this.bagOfStrings and if the removed entry is a vowel adds it to this.bagOfVowels */ public void findVowels() { // TODO } // findVowels /** * Counts and prints the frequency of each vowel in this.bagOfVowels */ public void countVowels() { // TODO // display the number of entries in the bag of vowels // create counters array // utilize toArray to get an array of vowels from ALL_VOWELS bag // utilize getFrequencyOf to get frequency of each vowel in the bag of vowels and display the count } // countVowels /** * Displays the number of entries and the content of the given bag * If the bag is empty displays appropriate message */ private void displayContentOfBag(BagInterface bag) { // TODO // If the bag is empty display appropriate message // Otherwise display the number of entries and the content of the given bag // Utilize Arrays.toString } // end displayContentOfBag public void displayContentOfBagOfStrings() { System.out.println("Displaying content of bag of strings:"); displayContentOfBag(this.bagOfStrings); } public void displayContentOfBagOfVowels() { System.out.println("Displaying content of bag of vowels:"); displayContentOfBag(this.bagOfVowels); } public static void main(String args[]) { long startTime = Calendar.getInstance().getTime().getTime(); // get current time in milliseconds System.out.println("--> calling constructor"); WorkingWithBags tester = new WorkingWithBags(); tester.displayContentOfBagOfStrings(); tester.displayContentOfBagOfVowels(); System.out.println(" --> calling addToBagOfStrings method to add valid and invalid strings"); String[] stringsToAdd = {"A", "e", "a1", "a c", "Ab", "CSUCI", "csuci", "cal", "if"}; for (int i = 0; i calling generateStrings method"); tester.generateStrings(); tester.displayContentOfBagOfStrings(); String entryToReplace = "ca"; System.out.println(" --> calling replaceGivenEntryWithItsReverse method to replace \"" + entryToReplace + "\""); String reverse = tester.replaceGivenEntryWithItsReverse(entryToReplace); if (reverse != null) System.out.println("\"" + entryToReplace + "\" was successfully replaced with \"" + reverse + "\""); else System.out.println("\"" + entryToReplace + "\" was not found"); entryToReplace = "cal"; System.out.println(" --> calling replaceGivenEntryWithItsReverse method to replace \"" + entryToReplace + "\""); reverse = tester.replaceGivenEntryWithItsReverse(entryToReplace); if (reverse != null) System.out.println("\"" + entryToReplace + "\" was successfully replaced with \"" + reverse + "\""); else System.out.println("\"" + entryToReplace + "\" was not found"); entryToReplace = "if"; System.out.println(" --> calling replaceGivenEntryWithItsReverse method to replace \"" + entryToReplace + "\""); reverse = tester.replaceGivenEntryWithItsReverse(entryToReplace); if (reverse != null) System.out.println("\"" + entryToReplace + "\" was successfully replaced with \"" + reverse + "\""); else System.out.println("\"" + entryToReplace + "\" was not found"); entryToReplace = "e"; System.out.println(" --> calling replaceGivenEntryWithItsReverse method to replace \"" + entryToReplace + "\""); reverse = tester.replaceGivenEntryWithItsReverse(entryToReplace); if (reverse != null) System.out.println("\"" + entryToReplace + "\" was successfully replaced with \"" + reverse + "\""); else System.out.println("\"" + entryToReplace + "\" was not found"); System.out.println(); tester.displayContentOfBagOfStrings(); System.out.println(" --> calling findVowels method"); tester.findVowels(); tester.displayContentOfBagOfStrings(); tester.displayContentOfBagOfVowels(); System.out.println(" --> calling countVowels method"); tester.countVowels(); long stopTime = Calendar.getInstance().getTime().getTime(); System.out.println(" The time required was " + (stopTime - startTime) + " milliseconds"); } // end main }--> calling constructor Displaying content of bag of strings: The bag is empty Displaying content of bag of vowels: The bag is empty --> calling addToBagOfStrings method to add valid and invalid strings "A" is invalid - the string can only contain lowercase letters "a1" is invalid - the string can only contain lowercase letters "ac" is invalid - the string can only contain lowercase letters "Ab" is invalid - the string can only contain lowercase letters "CSUCI" is invalid - the length of the string must be between 1 and 3 "csuci" is invalid - the length of the string must be between 1 and 3 Displaying content of bag of strings: The bag has 3 entries: [e, cal, if] --> calling generateStrings method *** Generating strings *** Displaying content of bag of strings: The bag has 38 entries: [e, cal, if, e, v, yam, nzn, e, ns, kze, yex, c, b, lu, u, v, tlt, nye, j, szy, ik, z, mb, d, cru, f, oe, h, v, cn, g, td, ky, s, con, ig, pe, a] --> calling replaceGivenEntryWithItsReverse method to replace "ca" "ca" was not found --> calling replaceGivenEntryWithItsReverse method to replace "cal" "cal" was successfully replaced with "lac" --> calling replaceGivenEntryWithItsReverse method to replace "if" "if" was successfully replaced with "fi" --> calling replaceGivenEntryWithItsReverse method to replace "e" "e" was successfully replaced with "e" Displaying content of bag of strings: The bag has 38 entries: [fi, a, lac, e, v, yqm, nzn, e, ns, kze, ycx, C, b, lu, u, v, tlt, nye, j, szy, ik, z, mb, d, cru, f, oe, h, v, cn, g, td, ky, s, con, ig, pe, e] --> calling findVowels method Displaying content of bag of strings: The bag is empty Displaying content of bag of vowels: The bag has 5 entries: [e, u, e, e, a] --> calling countVowels method There are 5 vowels in the bag of vowels: a occurs 1 times e occurs 3 times i occurs o times O occurs o times u occurs 1 times The time required was 22 milliseconds Process finished with exit code 0 --> calling constructor Displaying content of bag of strings: The bag is empty Displaying content of bag of vowels: The bag is empty --> calling addToBagOfStrings method to add valid and invalid strings "A" is invalid - the string can only contain lowercase letters "a1" is invalid - the string can only contain lowercase letters "ac" is invalid - the string can only contain lowercase letters "Ab" is invalid - the string can only contain lowercase letters "CSUCI" is invalid - the length of the string must be between 1 and 3 "csuci" is invalid - the length of the string must be between 1 and 3 Displaying content of bag of strings: The bag has 3 entries: [e, cal, if] --> calling generateStrings method *** Generating strings *** Displaying content of bag of strings: The bag has 38 entries: [e, cal, if, e, v, yam, nzn, e, ns, kze, yex, c, b, lu, u, v, tlt, nye, j, szy, ik, z, mb, d, cru, f, oe, h, v, cn, g, td, ky, s, con, ig, pe, a] --> calling replaceGivenEntryWithItsReverse method to replace "ca" "ca" was not found --> calling replaceGivenEntryWithItsReverse method to replace "cal" "cal" was successfully replaced with "lac" --> calling replaceGivenEntryWithItsReverse method to replace "if" "if" was successfully replaced with "fi" --> calling replaceGivenEntryWithItsReverse method to replace "e" "e" was successfully replaced with "e" Displaying content of bag of strings: The bag has 38 entries: [fi, a, lac, e, v, yqm, nzn, e, ns, kze, ycx, C, b, lu, u, v, tlt, nye, j, szy, ik, z, mb, d, cru, f, oe, h, v, cn, g, td, ky, s, con, ig, pe, e] --> calling findVowels method Displaying content of bag of strings: The bag is empty Displaying content of bag of vowels: The bag has 5 entries: [e, u, e, e, a] --> calling countVowels method There are 5 vowels in the bag of vowels: a occurs 1 times e occurs 3 times i occurs o times O occurs o times u occurs 1 times The time required was 22 milliseconds Process finished with exit code 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
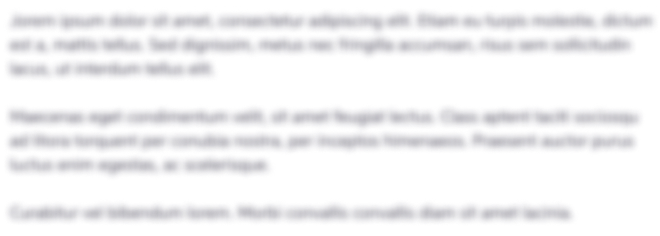
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started