Answered step by step
Verified Expert Solution
Question
1 Approved Answer
One of the benefits of a binary search tree is that ( if it is balanced ) , we can find any element without visiting
One of the benefits of a binary search tree is that if it is balanced we can find any element without visiting very many Nodes. To demonstrate this, update the findEntry method in BinarySearchTree to print how many Nodes were visited before the target item was found or was determined to not be in the tree Recall that findEntry is a private helper method called by contains, so in your test code youll have to call contains. Note: only one value should be printed, when the search is complete.
For example: Search complete. Nodes visited"
Hint: You can update this methods number of parameters!
package TreePackage;
public class BinarySearchTree extends BinaryTree implements SearchTreeInterface
public BinarySearchTree
public BinarySearchTreeT rootEntry
setRootNodenew BinaryNoderootEntry;
@Override
public boolean containsT anEntry
return findEntrygetRootNode anEntry null;
@Override
public T getEntryT anEntry
return findEntrygetRootNode anEntry;
private T findEntryBinaryNode rootNode, T anEntry
TODO update this method to print how many nodes were visited
T result null;
if rootNode null
We are not at a base case
T rootEntry rootNode.getData;
if anEntryequalsrootEntry
result rootEntry;
else if anEntrycompareTorootEntry
result findEntryrootNodegetLeftChild anEntry;
else
result findEntryrootNodegetRightChild anEntry;
return result;
@Override
public T addT anEntry
T result null;
if isEmpty
Tree is empty, add 'anEntry' to the root
setRootNodenew BinaryNodeanEntry;
else
Tree is not empty, recursively find the location of the new node
result addEntrygetRootNode anEntry;
return result;
private T addEntryBinaryNode rootNode, T anEntry
T result null;
compare anEntry and rootNode's data
int comparison anEntry.compareTorootNodegetData;
if comparison
result rootNode.getData;
rootNode.setDataanEntry;
else if comparison
anEntry is 'less than' rootNode.getData. Move left
if rootNodehasLeftChild
rootNode has a left child, so we can actually move left
result addEntryrootNodegetLeftChild anEntry;
else
rootNode has no left child but 'anEntry' belongs to rootNode's left
Create a new BinaryNode to be its left child
rootNode.setLeftChildnew BinaryNodeanEntry;
else
anEntry is 'greater than' rootNode.getData. Move right
if rootNodehasRightChild
rootNode has a right child, so we can actually move right
result addEntryrootNodegetRightChild anEntry;
else
rootNode.setRightChildnew BinaryNodeanEntry;
return result;
@Override
public T removeT anEntry
return null;
@Override
public void setTreeT rootData, BinaryTreeInterface leftTree, BinaryTreeInterface rightTree
throw new UnsupportedOperationExceptionBinary search trees do not support the 'setTree' method!";
package TreePackage;
import java.util.Iterator;
import java.util.NoSuchElementException;
public class BinaryTree implements BinaryTreeInterface
private BinaryNode root;
protected BinaryNode getRootNode
return root;
protected void setRootNodeBinaryNode newRoot
root newRoot;
public BinaryTree
root null;
public BinaryTreeT rootData
root new BinaryNoderootData;
public BinaryTreeT rootData, BinaryTree leftTree, BinaryTree rightTree
initializeTreerootData leftTree, rightTree;
@Override
public void setTreeT rootData
setTreerootData null, null;
@Override
public void setTreeT rootData, BinaryTreeInterface leftTree, BinaryTreeInterface rightTree
initializeTreerootDataB
Step by Step Solution
There are 3 Steps involved in it
Step: 1
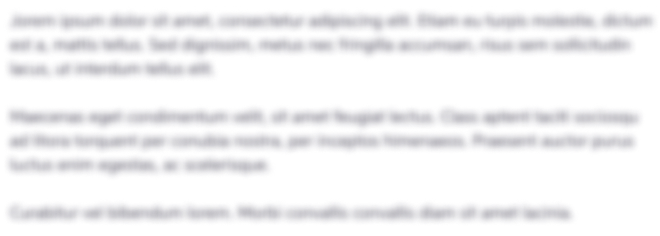
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started