Question
One of the most important skills in our craft is interpreting error messages. Remember the ones you receive when you attempt to compile and run
One of the most important skills in our craft is interpreting error messages. Remember the ones you receive when you attempt to compile and run the unmodified code.
The key abstractions employed in this program are Item, ItemStack, and Inventory. Complete ADT implementations have been provided for Item and Inventory.
A partial implementation has been provided for the ItemStack. Your task is to finish the update ItemStack ADT.
This assignment is smaller than the previous two (in terms of code and number of new concepts). Most of your time will be spent reviewing the basics of pointers. Spend the time reviewing. Practice with pointers. You will need to use pointers (in one form or another) for the reminder of the semester.
You must implement the:
1. Copy Constructor
2. Destructor
3. Assignment Operator
Note this is already provided and complete. Refer to our discussions of the copy-and-swap method.
Once you have completed the Copy Constructor, Destructor, and swap you are done with the Big-3.
4. Logical Equivalence (i.e., operator==).
5. Less-Than (i.e., operator<).
6. swap
Refer to the comments in each function in the code for additional detail.
Employ your Head-to-Head Testing Skills from CS 250.
This is the code, on top of the instructions provided ,some of the comments in the code have instructions, please follow them as well.
#include
#include "ItemStack.h"
const Item ItemStack::DEFAULT_ITEM(0, "Air");
//------------------------------------------------------------------------------
ItemStack::ItemStack()
:ItemStack(DEFAULT_ITEM, 0)
{
}
//------------------------------------------------------------------------------
ItemStack::ItemStack(const Item& inputItem, int s)
:quantity(s)
{
// Create a copy (clone) of inputItem and reference it with the this->item
// pointer
this->item = inputItem.clone();
}
//------------------------------------------------------------------------------
ItemStack::ItemStack(const ItemStack& src)
{
// 1 . Create a copy (clone) of src.item and reference it with the this->item pointer.
//
//
// 2. Do not forget to copy src.quantity.
//
}
//------------------------------------------------------------------------------
ItemStack::~ItemStack()
{
// Every pointer must be deleted to prevent memory leaks (item is a pointer).
}
//------------------------------------------------------------------------------
ItemStack& ItemStack::operator=(ItemStack rhs)
{
swap(*this, rhs);
return *this;
}
//------------------------------------------------------------------------------
Item ItemStack::getItem() const
{
return *(this->item);
}
//------------------------------------------------------------------------------
int ItemStack::size() const
{
return this->quantity;
}
//------------------------------------------------------------------------------
void ItemStack::addItems(int a)
{
this->quantity += a;
}
//------------------------------------------------------------------------------
void ItemStack::addItemsFrom(const ItemStack& other)
{
this->quantity += other.quantity;
}
//------------------------------------------------------------------------------
bool ItemStack::operator==(const ItemStack& rhs) const
{
// Compare this and rhs for equivalence based on the ids of this->item and rhs.item.
//
return false; // replace this line
}
//------------------------------------------------------------------------------
bool ItemStack::operator<(const ItemStack& rhs) const
{
// Order (sort) this and rhs based on the ids of this->item and rhs.item.
return false; // replace this line
}
//------------------------------------------------------------------------------
void ItemStack::display(std::ostream& outs) const
{
outs << std::right << "(" << std::setw(2) << this->size() << ") "
<< (this->getItem()).getName();
}
//------------------------------------------------------------------------------
void swap(ItemStack& lhs, ItemStack& rhs)
{
// Swap the item data members and quantity data members for lhs and rhs.
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
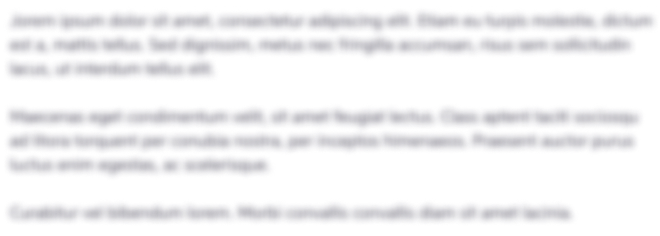
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started