Answered step by step
Verified Expert Solution
Question
1 Approved Answer
One use for removing random items is a word game like Scrabble in which players select seven tiles for their hand. The file WordGame.java contains
One use for removing random items is a word game like Scrabble in which players select seven tiles for their hand. The file WordGame.java contains a method for adding the Scrabble letter tiles to a bag. Implement a method that randomly removes seven letters from the bag and adds them to a players hand:
public static void selectLetters(Bag let, Bag player) Each time the program is run, it should pick seven random letters:
Your letters are: [A, N, T, G, I, H, R] Your letters are: [N, D, C, J, T, A, A] Your letters are: [E, I, E, D, T, S, R]
/*
* WordGame
*
* beginnings of a Scrabble-like word game
*
* adds the letter tiles from Scrabble to a bag and randomly selects
* seven for the user's hand
*/
public class WordGame
{
// number of letters in player's hand in Scrabble
public static final int LETTERS_PER_TURN = 7;
// distribution of Scrabble tiles
public static final char [][] ENGLISH_LETTERS =
{{'1', 'K', 'J', 'X', 'Q', 'Z'},
{'2', 'B', 'C', 'M', 'P', 'F', 'H', 'V', 'W', 'Y'},
{'3', 'G'},
{'4', 'L', 'S', 'U', 'D'},
{'6', 'N', 'R', 'T'},
{'8', 'O'},
{'9', 'A', 'I'},
{'0', 'E'}};
/*
* adds letter tiles to bag according to their distribution
*/
public static void addLetters(Bag b, char [] [] let)
{
// cycle through set of letters
for (int i = 0; i < let.length; i++)
{
// get count for letter group
int count = let [i][0] - '0';
if (count == 0) count = 12;
// for each letter in group
for (int j = 1; j < let[i].length; j++)
{
// add it to the bag the specified number of times
for (int k = 0; k < count; k++)
{
b.add(let[i][j]);
}
}
}
}
/*
* removes seven letters from bag and adds them to user's hand
*/
public static void selectLetters(Bag let, Bag player)
{
}
public static void main (String [] args)
{
// add letters to bag
Bag letters = new ArrayBag();
addLetters(letters, ENGLISH_LETTERS);
// give player seven random letters
Bag playerLetters = new LinkedBag();
selectLetters(letters, playerLetters);
System.out.println("Your letters are: " + playerLetters);
}
}
public interface Bag{ void add (T item); T remove(); T get(); boolean contains (T item); int size(); public String toString(); boolean remove (T item); T removeRandom(); T getRandom(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
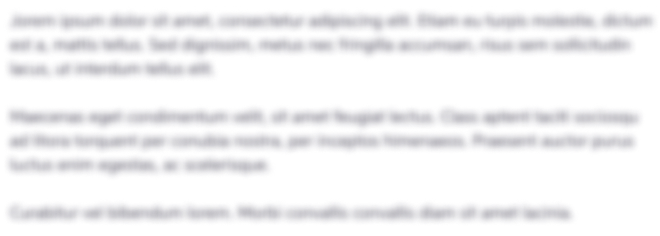
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started