Question
Only C++ Please edit the below main.cpp by adding code to the areas that stated code added by student // Assignment #3 Timing Matrix Multiplication
Only C++
Please edit the below main.cpp by adding code to the areas that stated "code added by student"
// Assignment #3 Timing Matrix Multiplication (Part A) // CLIENT
#include
// --------------- main --------------- #define MIN_MAT_SIZE 1 #define MIN_PERCENT 1 #define MAX_PERCENT 10.0
// for matrix of fixed sizes #define MAT_SIZE 5 typedef float* DynMat[MAT_SIZE]; void matMultDyn(const DynMat & matA, const DynMat & matB, DynMat & matC, int size); void matShowDyn(const DynMat & matA, int start, int show_size);
// for Sparse Matrix of dynamically allocated sizes void matMultDynSp(float **matA, float **matB, float **matC, int size); void matShowDynSp(float **matA, int mat_size, int start, int show_size);
int main() { int r, c, mat_size; clock_t startTime, stopTime; double randFrac; int randRow, randCol, numElement; float smallPercent; // easy initialization, but only for fixed-size matrices float m[MAT_SIZE][MAT_SIZE] = {{1, 2, 3, 4, 5}, {-1, -2, -3, -4, -5}, {1, 3, 1, 3, 1}, {0, 1, 0, 1, 0}, {-1, -1, -1, -1, -1}};
float n[MAT_SIZE][MAT_SIZE] = {{2, 1, 5, 0, 2}, {1, 4, 3, 2, 7}, {4, 4, 4, 4, 4}, {7, 1, -1, -1, -1}, {0, 0, 8, -1, -6}};
// for non-sparse dynamic matrix of fixed size DynMat mDyn, nDyn, ansDyn;
// allocate and load for (r = 0; r
// move the static data into the dyn input matrices m, n for (c = 0; c
matMultDyn(mDyn, nDyn, ansDyn, MAT_SIZE);
cout
cout
cout
// clean up for (r = 0; r > mat_size >> smallPercent; //check the input values and reset to MIN or MAX if out of range // code provided by student // // // non fixed size dynamic matrix float *matDynSp[mat_size], *matDynSpAns[mat_size];
// allocate rows and initialize to 0 for (r = 0; r
// initialize random seed to a fixed start 0 // so it will generate the same random sequence checked by the Auto Grader srand (0); // generate small % (between .1 and 10.) non-default values (between 0 and 1.0) numElement = mat_size * mat_size * smallPercent / 100; for (r = 0; r
matShowDynSp(matDynSp, mat_size, mat_size - 10, 10);
startTime = clock(); // ------------------ start matMultDynSp(matDynSp, matDynSp, matDynSpAns, mat_size); stopTime = clock(); // ---------------------- stop
matShowDynSp(matDynSpAns, mat_size, mat_size - 10, 10);
cout
// clean up for (r = 0; r
void matMultDyn(const DynMat & matA, const DynMat & matB, DynMat & matC, int size) { // code provided by student // //
}
void matShowDyn(const DynMat & matA, int start, int show_size) { int r, c;
// code provided by student // // cout
void matShowDynSp(float **matA, int mat_size, int start, int show_size) { int r, c; // check the display range and make sure only data within the matrix will be shown // code provided by student // //
cout.width(6); cout.precision(2); cout
After writing main.cpp, please answer the following questions
- What was the smallest mat_size that gave you a non-zero time?
- What happened when you doubled mat_size? What if tripled it, quadrupled it, etc?
- Create a table that shows the relationship between mat_size values and their times. Also indicate if smallPercent values would affect the times.
- How large an mat_size can you use before the program refuses to run (exception or run-time error due to memory overload) or it takes so long you can't wait for the run?
- What is the time complexity O(n) of your implementation?
- How did the data agree or disagree with your original time complexity estimate?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
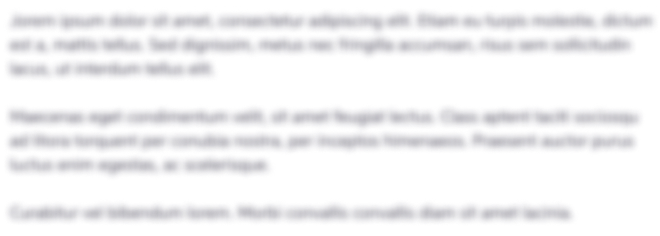
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started