Only need help creating the LibraryApp class!!!!!! Also need help with the AddBook method and this method which should also be in the BookCatinterface/BookCat: Sort this method has no parameter and returns nothing. It should sort all the books by the title.
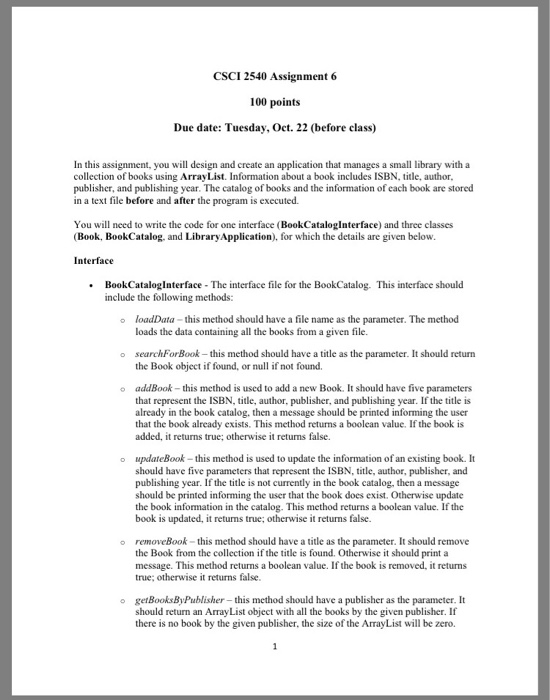
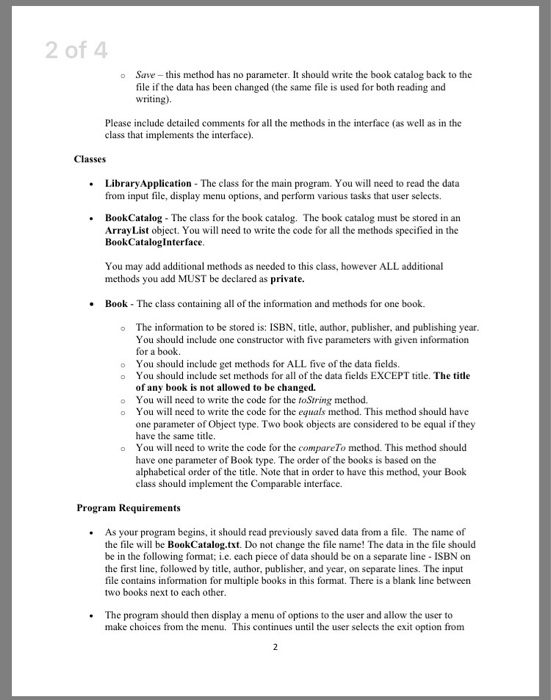
public class Book implements Comparable { private String ISBN; private String title; private String author; private String publisher; private int publishYear; public Book(String title) { this.title = title; ISBN = "0"; publishYear = 1111; } /** * constructor with given params. * @param iSBN given param * @param title given title * @param author given author * @param publisher given publisher * @param publishYear given published year. */ public Book(String iSBN, String title, String author, String publisher, int publishYear) { ISBN = iSBN; this.title = title; this.author = author; this.publisher = publisher; this.publishYear = publishYear; } public String getISBN() { return ISBN; } public String getTitle() { return title; } public String getAuthor() { return author; } public String getPublisher() { return publisher; } public int getPublishYear() { return publishYear; } public void setISBN(String iSBN) { ISBN = iSBN; } public void setAuthor(String author) { this.author = author; } public void setPublisher(String publisher) { this.publisher = publisher; } public void setPublishYear(int publishYear) { this.publishYear = publishYear; } @Override public String toString() { return "Title: " + title + " " + "Author: " + author + " " + "Publisher: " + publisher + " " + "Publish Year: " + publishYear + " " + "ISBN: " + ISBN; } @Override public int compareTo(Book other) { return this.title.compareToIgnoreCase(other.title); } } 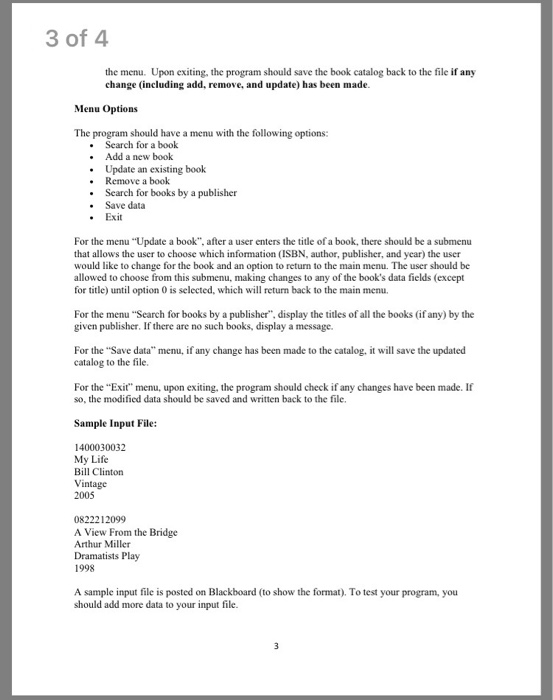
public class Book implements Comparable { private String ISBN; private String title; private String author; private String publisher; private int publishYear; public Book(String title) { this.title = title; ISBN = "0"; publishYear = 1111; } public Book(String iSBN, String title, String author, String publisher, int publishYear) { ISBN = iSBN; this.title = title; this.author = author; this.publisher = publisher; this.publishYear = publishYear; } public String getISBN() { return ISBN; } public String getTitle() { return title; } public String getAuthor() { return author; } public String getPublisher() { return publisher; } public int getPublishYear() { return publishYear; } public void setISBN(String iSBN) { ISBN = iSBN; } public void setAuthor(String author) { this.author = author; } public void setPublisher(String publisher) { this.publisher = publisher; } public void setPublishYear(int publishYear) { this.publishYear = publishYear; } @Override public String toString() { return "Title: " + title + " " + "Author: " + author + " " + "Publisher: " + publisher + " " + "Publish Year: " + publishYear + " " + "ISBN: " + ISBN; } @Override public int compareTo(Book other) { return this.title.compareToIgnoreCase(other.title); } }
CSCI 2540 Assignment 6 100 points Due date: Tuesday, Oct. 22 (before class) In this assignment, you will design and create an application that manages a small library with a collection of books using ArrayList. Information about a book includes ISBN, title, author, publisher, and publishing year. The catalog of books and the information of each book are stored in a text file before and after the program is executed. You will need to write the code for one interface (BookCatalogInterface) and three classes (Book, BookCatalog, and Library Application), for which the details are given below. Interface BookCatalogInterface - The interface file for the Book Catalog. This interface should include the following methods: loadData this method should have a file name as the parameter. The method loads the data containing all the books from a given file. search ForBook this method should have a title as the parameter. It should return the Book object if found, or null if not found. addBook - this method is used to add a new Book. It should have five parameters that represent the ISBN, title, author, publisher, and publishing year. If the title is already in the book catalog, then a message should be printed informing the user that the book already exists. This method returns a boolean value. If the book is added, it returns true; otherwise it returns false. updateBook - this method is used to update the information of an existing book. It should have five parameters that represent the ISBN, title, author, publisher, and publishing year. If the title is not currently in the book catalog, then a message should be printed informing the user that the book does exist. Otherwise update the book information in the catalog. This method returns a boolean value. If the book is updated, it returns true; otherwise it returns false. removeBook - this method should have a title as the parameter. It should remove the Book from the collection if the title is found. Otherwise it should print a message. This method returns a boolean value. If the book is removed, it returns true; otherwise it returns false. gerBooksBy Publisher - this method should have a publisher as the parameter. It should return an ArrayList object with all the books by the given publisher. If there is no book by the given publisher, the size of the ArrayList will be zero. 1 2 of 4 Save this method has no parameter. It should write the book catalog back to the file if the data has been changed (the same file is used for both reading and writing). Please include detailed comments for all the methods in the interface (as well as in the class that implements the interface). Classes Library Application - The class for the main program. You will need to read the data from input file, display menu options, and perform various tasks that user selects. BookCatalog - The class for the book catalog, The book catalog must be stored in an ArrayList object. You will need to write the code for all the methods specified in the Book CatalogInterface You may add additional methods as needed to this class, however ALL additional methods you add MUST be declared as private. Book - The class containing all of the information and methods for one book. The information to be stored is: ISBN, title, author, publisher, and publishing year. You should include one constructor with five parameters with given information for a book . You should include get methods for ALL five of the data fields. You should include set methods for all of the data fields EXCEPT title. The title of any book is not allowed to be changed. You will need to write the code for the toString method. You will need to write the code for the equals method. This method should have one parameter of Object type. Two book objects are considered to be equal if they have the same title. You will need to write the code for the comparelo method. This method should have one parameter of Book type. The order of the books is based on the alphabetical order of the title. Note that in order to have this method, your Book class should implement the Comparable interface. Program Requirements As your program begins, it should read previously saved data from a file. The name of the file will be BookCatalog.txt. Do not change the file name! The data in the file should be in the following format; i.e. each piece of data should be on a separate line - ISBN on the first line, followed by title, author, publisher, and year, on separate lines. The input file contains information for multiple books in this format. There is a blank line between two books next to each other. The program should then display a menu of options to the user and allow the user to make choices from the menu. This continues until the user selects the exit option from 2 3 of 4 the menu. Upon exiting, the program should save the book catalog back to the file if any change (including add, remove, and update) has been made. Menu Options The program should have a menu with the following options: Search for a book Add a new book Update an existing book Remove a book Search for books by a publisher Save data Exit For the menu "Update a book", after a user enters the title of a book, there should be a submenu that allows the user to choose which information (ISBN, author, publisher, and year) the user would like to change for the book and an option to return to the main menu. The user should be allowed to choose from this submenu, making changes to any of the book's data fields (except for title) until option 0 is selected, which will return back to the main menu. For the menu "Search for books by a publisher", display the titles of all the books (if any) by the given publisher. If there are no such books, display a message. For the "Save data" menu, if any change has been made to the catalog, it will save the updated catalog to the file. For the "Exit" menu, upon exiting, the program should check if any changes have been made. If So, the modified data should be saved and written back to the file. Sample Input File: 1400030032 My Life Bill Clinton Vintage 2005 0822212099 A View From the Bridge Arthur Miller Dramatists Play 1998 A sample input file is posted on Blackboard (to show the format). To test your program, you should add more data to your input file. CSCI 2540 Assignment 6 100 points Due date: Tuesday, Oct. 22 (before class) In this assignment, you will design and create an application that manages a small library with a collection of books using ArrayList. Information about a book includes ISBN, title, author, publisher, and publishing year. The catalog of books and the information of each book are stored in a text file before and after the program is executed. You will need to write the code for one interface (BookCatalogInterface) and three classes (Book, BookCatalog, and Library Application), for which the details are given below. Interface BookCatalogInterface - The interface file for the Book Catalog. This interface should include the following methods: loadData this method should have a file name as the parameter. The method loads the data containing all the books from a given file. search ForBook this method should have a title as the parameter. It should return the Book object if found, or null if not found. addBook - this method is used to add a new Book. It should have five parameters that represent the ISBN, title, author, publisher, and publishing year. If the title is already in the book catalog, then a message should be printed informing the user that the book already exists. This method returns a boolean value. If the book is added, it returns true; otherwise it returns false. updateBook - this method is used to update the information of an existing book. It should have five parameters that represent the ISBN, title, author, publisher, and publishing year. If the title is not currently in the book catalog, then a message should be printed informing the user that the book does exist. Otherwise update the book information in the catalog. This method returns a boolean value. If the book is updated, it returns true; otherwise it returns false. removeBook - this method should have a title as the parameter. It should remove the Book from the collection if the title is found. Otherwise it should print a message. This method returns a boolean value. If the book is removed, it returns true; otherwise it returns false. gerBooksBy Publisher - this method should have a publisher as the parameter. It should return an ArrayList object with all the books by the given publisher. If there is no book by the given publisher, the size of the ArrayList will be zero. 1 2 of 4 Save this method has no parameter. It should write the book catalog back to the file if the data has been changed (the same file is used for both reading and writing). Please include detailed comments for all the methods in the interface (as well as in the class that implements the interface). Classes Library Application - The class for the main program. You will need to read the data from input file, display menu options, and perform various tasks that user selects. BookCatalog - The class for the book catalog, The book catalog must be stored in an ArrayList object. You will need to write the code for all the methods specified in the Book CatalogInterface You may add additional methods as needed to this class, however ALL additional methods you add MUST be declared as private. Book - The class containing all of the information and methods for one book. The information to be stored is: ISBN, title, author, publisher, and publishing year. You should include one constructor with five parameters with given information for a book . You should include get methods for ALL five of the data fields. You should include set methods for all of the data fields EXCEPT title. The title of any book is not allowed to be changed. You will need to write the code for the toString method. You will need to write the code for the equals method. This method should have one parameter of Object type. Two book objects are considered to be equal if they have the same title. You will need to write the code for the comparelo method. This method should have one parameter of Book type. The order of the books is based on the alphabetical order of the title. Note that in order to have this method, your Book class should implement the Comparable interface. Program Requirements As your program begins, it should read previously saved data from a file. The name of the file will be BookCatalog.txt. Do not change the file name! The data in the file should be in the following format; i.e. each piece of data should be on a separate line - ISBN on the first line, followed by title, author, publisher, and year, on separate lines. The input file contains information for multiple books in this format. There is a blank line between two books next to each other. The program should then display a menu of options to the user and allow the user to make choices from the menu. This continues until the user selects the exit option from 2 3 of 4 the menu. Upon exiting, the program should save the book catalog back to the file if any change (including add, remove, and update) has been made. Menu Options The program should have a menu with the following options: Search for a book Add a new book Update an existing book Remove a book Search for books by a publisher Save data Exit For the menu "Update a book", after a user enters the title of a book, there should be a submenu that allows the user to choose which information (ISBN, author, publisher, and year) the user would like to change for the book and an option to return to the main menu. The user should be allowed to choose from this submenu, making changes to any of the book's data fields (except for title) until option 0 is selected, which will return back to the main menu. For the menu "Search for books by a publisher", display the titles of all the books (if any) by the given publisher. If there are no such books, display a message. For the "Save data" menu, if any change has been made to the catalog, it will save the updated catalog to the file. For the "Exit" menu, upon exiting, the program should check if any changes have been made. If So, the modified data should be saved and written back to the file. Sample Input File: 1400030032 My Life Bill Clinton Vintage 2005 0822212099 A View From the Bridge Arthur Miller Dramatists Play 1998 A sample input file is posted on Blackboard (to show the format). To test your program, you should add more data to your input file