Question
Only need help for part C In java Part A Note that in addition to the required methods below, you are free to add as
Only need help for part C
In java
Part A
Note that in addition to the required methods below, you are free to add as many other private methods as you want.
(a) Character Class Character.java represents a character in our battle game. The monster and the player will both
be instances of the Character class, each with their own attributes. The Character class should contain the following (private) attributes:
A String name A double attack value A double maximum health value A double current health value A int number of wins in the battle game
Here are the required public methods for this class. Note that you will also have to add getters and setters for the instance attributes as needed.
1) A constructor
The constructor for the Character class takes one String, two doubles, and one int as input. These parameters represent the name, attack value, maximum health, and number of wins in the battle game for the character, in that order. Note that the current health of a new character is the same as the maximum health.
2) The toString method
This method returns a String consisting of the characters name and current health. Format the String in any way you want. This method will be very handy for debugging your code, and will be used during the battle game to keep track of the health of each character.
3) calcAttack method
This method calculates how much attack damage one character does in a battle. The calculation is as follows: Take the characters attack value and multiply it by a random value between 0.3 (inclusive) and 0.7 (exclusive). Return this value as a double. Use the Random class to generate the random numbers, and do not use a seed.
4) takeDamage method In this method, we take the damage done to this character as a double parameter, and then we
subtract this value from the characters current health. This method does not return anything. 5) increaseWins method
This method will increase the number of wins by the character by one, and does not return anything. This method will be called when the character wins the battle game.
(b) FileIO FileIO.java must contain a static method readCharacter.
This method takes as input a filename as a String parameter, and returns a new Character, using the constructor defined in the Character class.
The readCharacter method must use a FileReader and a BufferedReader to open the file specified by filename. Make sure to have two catch blocks to catch both FileNotFoundException and IOException when reading from the file. In these cases, throw an IllegalArgumentException with an appropriate message that the file was not found, or that there was an IO exception. Note that the catch block for the FileNotFoundException must be directly above the catch block for the IOException.
If you prefer, you can also use the throws statement to throw the FileNotFoundException and IOException up to the calling playGame method. Then these Exceptions would be caught in a try/catch block there.
Example player.txt and monster.txt files are provided with the assignment. The format of these files is exactly this, one value per line:
Name of the character Attack value Maximum health Number of wins so far in the battle game
Use the readLine() method of the BufferedReader to get the lines from the file. Do not use the Scanner class. Then, use the Double.parseDouble() and Integer.parseInt() methods to parse the lines. Finally, use those values to create and return a new Character.
(c) BattleGame The code for this part will go in a file named BattleGame.java.
The BattleGame class only contains the playGame method, and the doAttack method, though you are allowed to create as many other private methods as you want.
1) playGame method must do the following things: Create the player and their enemy, using the FileIO.readCharacter method
After a character is created, print the characters name, current health, attack value, and number of wins.
We recommend you place this print statement in a public method inside the Character class
Use a Scanner to take input from the user Loop while both the player and the monster have health above zero
Ask the user for a command
For this question, the only options will be attack and quit
If the input is attack:
Call the doAttack method (in the BattleGame class) with the player as first parameter and the monster as second parameter
Call the doAttack method with the monster as first parameter and the player as second parameter
If the input is quit
Print a goodbye message and return from the method
If the input is anything else
Print a message that the input was not recognized and suggest the attack or quit commands
If the loop stops because one of the character's health is zero or below,then that character is knocked out. Print an appropriate message either congratulating the player, or saying how they lost. Also make sure to call the increaseWins method on either the player or the monster, depending who won.
2) The doAttack method takes two Characters as input, and returns nothing as output. This method must:
Get the damage from the first Character by calling the calcAttack method
Print out a statement with the first characters name and how much damage they do.
Apply the damage to the second Character with the takeDamage method
If the second characters current health is still above zero, print a message with their name and current health. If their current health is zero or below, print out a message saying that the second character was knocked out.
To make your output nicer, we suggest using a String formatting statement, though this is not necessary. For example, you could write this to only show two decimal places of the attack damage: String attackStr = String.format(%1$.2f, attack);
Here is some sample output produced by running the playGame method after Question 1 has been fin- ished. Output for the finished assignment is found at the bottom of this document. Note that for this assignment, your output doesnt need to match this exactly. You are free to change these statements as you wish, as long as the required information still appears
Part B:
(a) Spell Write a class Spell. A Spell has the following private attributes:
A String name
A double minimum damage
A double maximum damage
A double chance of success for the spell (from 0 to 1)
The Spell class also contains the following public methods:
1.A constructor that takes as input the name, minimum and maximum damage, and chance of
success for the spell
An IllegalArgumentException must be thrown if the minimum damage is less than 0 or greater than the maximum damage, or the chance of success is less than zero or greater than one.
2.getName which returns the name of the spell
3.A getDamage method that returns the damage for a spell casting. First, a random number from 0 to 1 is generated. If the random number is above the chance of success, the spell fails, and 0 damage is returned. Otherwise, a random number from the minimum damage to the maximum damage is returned. Use the Random class to generate the random numbers, and do not use a seed.
4.A toString method. The String which is returned must contain the name, minimum and maximum damage, and the success chance as a percentage from 0 to 100 (so a chance of 0.5 is reported as 50.0%)
(b) Character Modify the Character class.
1) Add a private static attribute spells. This variable will be an ArrayList which contains Spells that the character can cast. Note that this list of spells will be available to all Characters.
2) Add a setter method setSpells for the spells attribute. Recall that a setter method allows other classes to set values for private attributes. This method will take one parameter, and will copy the Spells contained in the input parameter into a new ArrayList stored in the spells attribute. This method does not return a value.
3) Add a new method castSpell, which takes the name of a spell to cast as a parameter spellName. This method will return the damage done by the spell as a double.
This method will iterate through the list of spells, searching for the spell with the name matching spellName. Note that you should ignore the capitalization of the spell name when matching.
If the spell cannot be found, print a message which says that the character tried to cast an unknown spell. Also return zero damage.
If the spell is found, the damage done by the spell is given by calling the getDamage method on the spell. If the damage returned is zero, then the spell failed to cast. Print a message that the character failed to cast the spell. If the damage was above zero, print a message that the spell was cast. Return the damage from the castSpell method.
In all messages printed in this castSpell method, the message should have the characters name, as well as the name of the spell. See the example output at the end of this document.
(c) FileIO Add a new static method, the readSpells method. This method takes a filename of spells to read,
and returns an ArrayList of Spells.
An example of a file containing spells is found in the provided files as spells.txt.
Read the file indicated by the method parameter filename, by using a BufferedReader. Catch the IOException and FileNotFoundException exceptions in two separate catch blocks, and throw an IllegalArgumentException with an appropriate error message if these occur. Note that the catch block for the FileNotFoundException must be above the catch block for the IOException.
As before, if you prefer you can also use the throws statement to throw the FileNotFoundException and IOException up to the calling playGame method. Then these Exceptions would be caught in a try/catch block there.
Each spell is on one line, consisting of the spell name, the min damage, the max damage, and the chance for success, separated by spaces. Use the split() method to split each line of the file by the space delimiter.
Use the four values on each line of the file to create new Spell instances, and then add these Spell instances to the ArrayList which will be returned from this method. Do not use the Scanner class to parse the file.
Part C:
(a) playGame Change the playGame method in the BattleGame class to allow the player to cast spells.
1) At the beginning of the playGame method, call the readSpells method in the FileIO class with the filename of the file containing spells. This method returns an ArrayList of spells.
Print out each spell (using the toString method of the Spell class), so that the player knows which spells they may cast.
Then call the setSpells method in the Character class with this list of spells.
2) In addition to the attack and quit commands, we will allow the user to cast spells. If the input is not attack or quit, then the input must be passed to the castSpell method on the players character, as we assume it is the name of a spell to cast.
The damage returned from the castSpell method should then be applied to the monster, using the takeDamage method.
b) FileIO In the FileIO class add a public static method named writeCharacter. This method takes as
input a Character to write, and a String which is the filename to write to.
Within this writeCharacter method, use the FileWriter and BufferedWriter objects to write the characters information back to a file. Make sure to catch the IOException when writing a file, and throw an IllegalArgumentException with an appropriate error message.
If you prefer, you can also use the throws statement to throw the IOException up to the calling playGame method. Then this Exceptions would be caught in a try/catch block there.
The format of the file that is written must match the format that is expected when a character is read. That is, you should be able to write a character to a file, and then read a character from that same file.
Recall that the format of a character in a file is each value on a separate line: Name of the character Attack value Maximum health
Number of wins so far in the battle game Note that this means you will have to write getter methods for the attributes in the Character
class.
(c) BattleGame
In the playGame method, after you have increased the wins for either the monster or the player, print how many wins each character has.
As well, you must write the characters to the files you loaded them from. For example, write the player character to player.txt or the monster character to monster.txt.
Call the writeCharacter method in the FileIO class, and pass the character who won and the file which stores that character as parameters. This will save the number of wins for that character, so that after playing the battle game multiple times, you will know which character has won more often.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
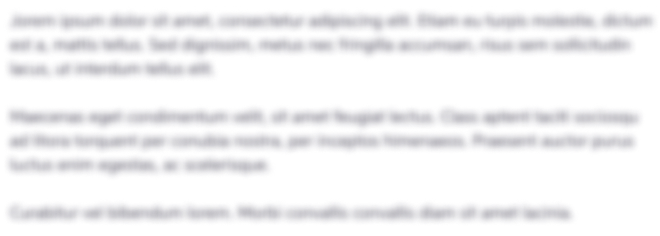
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started