Question
Open NetBeans or Eclipse and create a Java project with the following details. For Project Name include: DataAnalytics For the Main Class include: DataAnalytics In
Open NetBeans or Eclipse and create a Java project with the following details.
For Project Name include: DataAnalytics
For the Main Class include: DataAnalytics
In your Code window for this class, shown below, copy the program code shown in Figure 1 below, in the appropriate places, except substitute your own name in place of Sammy Student.
Figure 1 Source Code for the Data Analytics File Processing Program
import java.io.*; import java.util.ArrayList; import java.util.Scanner; // Sammy Student: Data Analysis with Java File Processing class DataAnalytics { public static void main(String args[]) { // declare an object to receive the data Scanner scan = new Scanner(System.in); // declare an array list to hold the data ArrayList list = new ArrayList int count = 0; int num = 0; int val = 0; String line = "";
try { // create or append to the file FileWriter fileOut = new FileWriter("outData.txt"); BufferedWriter fout = new BufferedWriter(fileOut);
System.out.println("how many data items?"); count = scan.nextInt(); for (int i = 1; i <= count; i++) { System.out.println("enter a data value"); val = scan.nextInt(); fout.write(val + " "); } System.out.println("thank you ... the data has been recorded!");
// close the output stream objects fout.close(); fileOut.close();
scan.close(); } catch(Exception e) { // catch an exception if any arises System.err.println("Error: " + e.getMessage()); } } } Test the Program and Write the Data
Once you have successfully compiled your program enter a valid list of data values as shown below. Your initial program code will request a count of data values to be recorded and then write the data values to a text file.
[ Program Output ]
how many data items? 5 enter a data value 20 enter a data value 50 enter a data value 10 enter a data value 80 enter a data value 90 thank you . . . the data has been recorded!
STEP 4 Open the Data File and Review the Contents of the File
Within your software development environment, click [ File ] and then [ Open ] to review the contents of the data file that was created by running the program. Locate the outData.txt text file and open the file to view its contents.
STEP 5 Read the Data File
Once you have successfully compiled and tested your starter program and opened the text file with the data, you will now supplement your program with some program code that will read the data in the outData.txt text file and individually place the data values into a Java ArrayList .
Place the statements that are shown in Figure 2 before the closing curly brace " } " of the try block.
PROJECT Java File Processing Application: Java Data Analytics
Figure 2 Additional Source Code for the Data Analytics File Processing Program
Save your program and run the program with the sample data that was given earlier. Observe the output of your program.
STEP 6 Supplement the Program Code Statements
Supplement again the program by including a method that displays the data that was recorded into the Java ArrayList .
Within your program, create a method named DisplayData() , which has this signature and definition. Place the method below the main() method and before the closing curly brace " } " of the class definition.
public static void DisplayData(ArrayList { for (int i = 0; i < num.size(); i++) System.out.println(num.get(i).toString()); }
Then, before the closing curly brace " } " of the try block, place these two statements.
System.out.println("display unsorted data"); DisplayData(list);
Save your program and run the program with the sample data that was given earlier.
Now include a method that will sort the data that was placed into the ArrayList . Place the method below the DisplayData() method and before the closing curly brace " } " of the class definition. Use the Bubble Sort routine that follows in Figure 3 .
In an appropriate place in the main() method include these lines of code to show that the data has been sorted.
System.out.println("display sorted data"); DisplayData(list); PROJECT Java File Processing Application: Java Data Analytics
Figure 3 Source Code for the Data Analytics File Processing Program
STEP 7 Modify the Program
After testing your program that it displays the original data in an unsorted order and a sorted order, modify again the program to include each of these variations:
Add a new method named MaxMin() that will find the smallest and largest value in the ArrayList after the data has been sorted.
Add a new method named Average() that will find the average value in the ArrayList after the data has been sorted.
Save your program and perform a trial run of it. Test your program with data similar to that shown below.
[ Program Output ]
how many data items? 5 enter a data value 20 enter a data value 50 enter a data value 10 enter a data value 60 enter a data value 40 thank you . . . the data has been recorded! |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
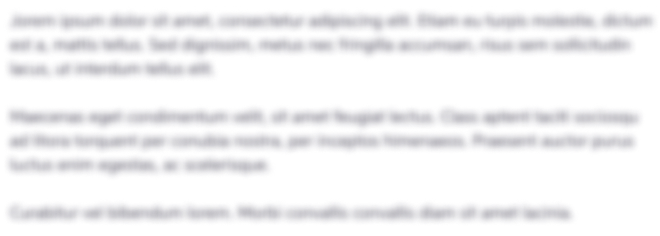
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started