Question
Open the clock-display project in BlueJ and save it as a separate project to work on for this lab, e.g., lab5-alarm . Modify the ClockDisplay
- Open the clock-display project in BlueJ and save it as a separate project to work on for this lab, e.g., lab5-alarm.
- Modify the ClockDisplay class to support a 12-hour clock as described in Exercise 3.38, including 'am' or 'pm' in the display. You will need to think about how to do this, as there is more than one way. The only restriction is that you NOT modify the NumberDisplay class, and NOT modify the signatures of existing methods & constructors. Read Exercise 3.39 to get some ideas.
- If you need help, watch the textbook video note on solving the 12-hour clock exercise(https://www.youtube.com/watch?v=1p5Uf7LDoO0). However, you should spend significant time on this yourself before watching the video - this is a tricky exercise and your problem-solving skills will be improved by giving it your full effort before watching the video.
- Now add an alarm clock feature to the clock (once again, update only the ClockDisplay class). You need to decide what additional fields are required. The clock should have the following public methods and functionality (names & parameters should be exactly as shown; consider this a spec from your client that needs to be met exactly).
- void turnAlarmOn(int hour, int minute) - turns the alarm on and sets it to the given time; hour may be an integer from 0 - 23 representing 12 am - 11 pm. (Note: This method does not ring the alarm, it just sets it to 'on' and the given time so that when the clock ticks to that time, it will ring.)
- void turnAlarmOff() - turns the alarm off. (When the alarm is off, it should not ring, even if the clock ticks to the alarm time.)
- When the clock is created, the alarm should start in the 'off' position.
- When the clock ticks to the set alarm time, if the alarm is turned on, an alarm should ring. You can simulate the ring with a print message.
- If you have questions about how the alarm should function, post in Lesson 5 or Student Q&A Discussion topic, and the instructor will answer. It is not uncommon in software development that a specification is unclear; asking clarifying questions is an important skill to develop.
- The signatures of the existing methods in ClockDisplay should also not be changed, but you are welcome to update the implementation of existing methods (and you will need to do so in order to add the alarm function).
- As usual, update the class header Javadoc comment block and include an up-to-date method header Javadoc comment.
- As usual, adhere to Java style guidelines as described in Appendix J.
- Test your code thoroughly! Make sure that each tick of the clock works as expected. This can be tricky, so spend some time thinking about test cases that cover all the relevant scenarios. Use the Inspector in BlueJ to examine the object's fields as you test.
- When you have completed the assignment, create a jar file of your project.
- While the project is open in BlueJ, choose Project->Create Jar File...
- Ensure that the "Include source" check box is checked
***********************************************************************
Clock Display project in BlueJ has two classes as below:
ClockDisplay:
/** * The ClockDisplay class implements a digital clock display for a * European-style 24 hour clock. The clock shows hours and minutes. The * range of the clock is 00:00 (midnight) to 23:59 (one minute before * midnight). * * The clock display receives "ticks" (via the timeTick method) every minute * and reacts by incrementing the display. This is done in the usual clock * fashion: the hour increments when the minutes roll over to zero. * * @author Michael Klling and David J. Barnes * @version 2016.02.29 */ public class ClockDisplay { private NumberDisplay hours; private NumberDisplay minutes; private String displayString; // simulates the actual display /** * Constructor for ClockDisplay objects. This constructor * creates a new clock set at 00:00. */ public ClockDisplay() { hours = new NumberDisplay(24); minutes = new NumberDisplay(60); updateDisplay(); }
/** * Constructor for ClockDisplay objects. This constructor * creates a new clock set at the time specified by the * parameters. */ public ClockDisplay(int hour, int minute) { hours = new NumberDisplay(24); minutes = new NumberDisplay(60); setTime(hour, minute); }
/** * This method should get called once every minute - it makes * the clock display go one minute forward. */ public void timeTick() { minutes.increment(); if(minutes.getValue() == 0) { // it just rolled over! hours.increment(); } updateDisplay(); }
/** * Set the time of the display to the specified hour and * minute. */ public void setTime(int hour, int minute) { hours.setValue(hour); minutes.setValue(minute); updateDisplay(); }
/** * Return the current time of this display in the format HH:MM. */ public String getTime() { return displayString; } /** * Update the internal string that represents the display. */ private void updateDisplay() { displayString = hours.getDisplayValue() + ":" + minutes.getDisplayValue(); } }
Number Display:
/** * The NumberDisplay class represents a digital number display that can hold * values from zero to a given limit. The limit can be specified when creating * the display. The values range from zero (inclusive) to limit-1. If used, * for example, for the seconds on a digital clock, the limit would be 60, * resulting in display values from 0 to 59. When incremented, the display * automatically rolls over to zero when reaching the limit. * * @author Michael Klling and David J. Barnes * @version 2016.02.29 */ public class NumberDisplay { private int limit; private int value;
/** * Constructor for objects of class NumberDisplay. * Set the limit at which the display rolls over. */ public NumberDisplay(int rollOverLimit) { limit = rollOverLimit; value = 0; }
/** * Return the current value. */ public int getValue() { return value; }
/** * Return the display value (that is, the current value as a two-digit * String. If the value is less than ten, it will be padded with a leading * zero). */ public String getDisplayValue() { if(value < 10) { return "0" + value; } else { return "" + value; } }
/** * Set the value of the display to the new specified value. If the new * value is less than zero or over the limit, do nothing. */ public void setValue(int replacementValue) { if((replacementValue >= 0) && (replacementValue < limit)) { value = replacementValue; } }
/** * Increment the display value by one, rolling over to zero if the * limit is reached. */ public void increment() { value = (value + 1) % limit; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
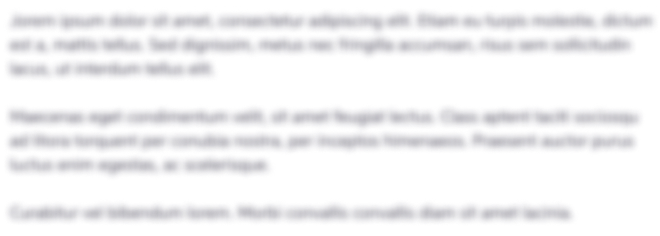
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started