Question
Opengl/C++ windows ** I need to fix the cannon code *** I need to move the cannon and the ball read the instructions below Develop
Opengl/C++ windows ** I need to fix the cannon code *** I need to move the cannon and the ball read the instructions below
Develop a simple 3D shooting game using OpenGL.
The program should meet the following requirements:
Create and add a 3D cannon such that it aims forward from the viewer towards the far plane (Fig. 1). It is okay to show only the tip (firing end) of the cannon.
The cannon can turn left and right, and up and down (yaw and pitch movements) like a real cannon. The
user controls left-right rotation with right-left arrow keys, and up-down rotation with up-down arrow keys. The rotation in either direction should be clamped to a certain range (say, 120 degrees) so the cannonball would stay within the view volume.
The cannon should fire a cannonball (sphere) every time the user presses the space bar. The ball should fly in the direction of the current orientation of the cannon. Once the ball goes through any wall and thus no longer visible, delete it from the scene to avoid clogging the memory. Therefore, the system will store no more than a few (fired) balls at any given time.
During the game, display text at the top of the screen that shows how many teapots are currently left
(Fig. 1).
When the cannonball hits one of the teapots (collision detection needed here), the hit teapot must immedi-ately disappear from the scene (and so must this cannonball). The text must also be updated accordingly with a new number (one less teapots left).
When the last teapot is hit, the game is over and the text should be replaced with Continue? (Y/N). If the user presses y, start the game over. If n, exit program.
The background walls (all 5 of them) must be texture mapped, although Fig. 1 doesnt show it. You can use any tileable texture image of your choice, but make sure the image file size is less then 1MB (with no bigger than 512512 pixel resolution). Do not use multiple texture images.
If the user presses q at any time (even during the game), exit program.
If the animation is too fast on your computer, slow it down to a level where each teapots movement and the cannonballs movement are clearly recognizable.
Make sure there is no sudden jump or discontinuity in the animation. The whole sequence of transfor-mations must be smooth and continuous.
#include
#include // standard definitions
#include // math definitions
#include // standard I/
#include
using namespace std;
// Camera position
float x = 0.0, y = -25.0, z = -5.0; // initialyaxis 5 units south of origin
float movespeed = 0.0; // initialyaxis camera doesn't move
float xaxis = 0.0, yaxis = 1.0; // camera points initialyaxis along y-axis
float angle = 0.0; // angle of rotation for the camera direction
float green, red, blue;
struct Sphere {
GLfloat x, y, z;
GLfloat a, b, c;
};
Sphere mySpheres[1];
GLfloat coordX[9], coordY[9], coordZ[9], ratio[9];
void changeSize(int w, int h)
{
float ratio = ((float)w) / ((float)h); // window aspect ratio
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(60, ratio, 1, 200.0);
gluLookAt(0, 0, 2, 0, 0, 0, 0, 1, 0);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
// Update with each idle event
void update(void)
{
for (int i = 0; i
mySpheres[i].z -= .01;
// ratio[i] = mySpheres[i].z / coordZ[i];
// mySpheres[i].x = coordX[i] * ratio[i];
// mySpheres[i].y = coordY[i] * ratio[i];
}
glutPostRedisplay();
}
void drawcylinder()
{
glPushMatrix();
glTranslatef(0, -2, -1);
GLUquadric* qobj;
qobj = gluNewQuadric();
gluQuadricDrawStyle(qobj, GL_EYE_LINEAR);
gluCylinder(qobj, 0.6, 0.6, 0.6, 50, 50);
glPopMatrix();
}
void drawsqaure()
{
for (int i = 0; i
{
glColor3d(0, 0, 1);
glPushMatrix();
glTranslatef(mySpheres[i].x, mySpheres[i].y, mySpheres[i].z);
glTranslatef(0, -2, -1);
glutSolidSphere(0.25, 20, 20);
glPopMatrix();
}
}
void newSphere(int i) {
mySpheres[i].x = 0;
mySpheres[i].y = 0;
mySpheres[i].z = -1;
coordX[i] = mySpheres[i].x;
coordY[i] = mySpheres[i].y;
coordZ[i] = mySpheres[i].z;
}
void firstSpheres() {
for (int i = 0; i
mySpheres[i].x = 0;
mySpheres[i].y = 0;
mySpheres[i].z = -1;
coordX[i] = mySpheres[i].x;
coordY[i] = mySpheres[i].y;
coordZ[i] = mySpheres[i].z;
}
}
// Draw the entire scene
void renderScene(void)
{
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
drawsqaure();
for (int i = 0; i
if (mySpheres[i].z > 0)
newSphere(i);
}
drawsqaure();
drawcylinder();
glutSwapBuffers(); // Make it all visible
}
void init()
{
glClearColor(1, 1, 1, 1);
GLfloat mat_shininess[] = { 50.0 };
GLfloat light_position[] = { 1.0, 1.0, 1.0, 0.0 };
GLfloat lightInt[] = { .9, .9, .9, 1 };
glShadeModel(GL_SMOOTH);
glEnable(GL_COLOR_MATERIAL);
glEnable(GL_LIGHTING);
glEnable(GL_LIGHT0);
glEnable(GL_DEPTH_TEST);
glLightfv(GL_LIGHT0, GL_POSITION, light_position);
glLightfv(GL_LIGHT0, GL_DIFFUSE, lightInt);
}
void myKeyboard(unsigned char key, int mouseX, int mouseY)
{
if (key == 81 || key == 113)
{
exit(-1);
}
if (key == 32)
{
update();
firstSpheres();
renderScene();
}
}
int main(int argc, char *argv [])
{
// general initializations
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DEPTH | GLUT_DOUBLE | GLUT_RGBA);
glutInitWindowPosition(100, 100);
glutInitWindowSize(800, 400);
glutCreateWindow("Flying Sphere");
// register callbacks
glutReshapeFunc(changeSize); // window reshape callback
// glutDisplayFunc(drawcylinder);
// glutDisplayFunc(drawsqaure);
glutDisplayFunc(renderScene); // (re)display callback
firstSpheres();
glutIdleFunc(update); // incremental update
glutKeyboardFunc(myKeyboard);
init();
glEnable(GL_DEPTH_TEST);
// enter GLUT event processing cycle
glutMainLoop();
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
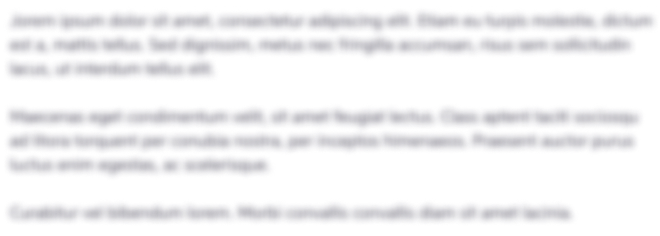
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started