Question
Operating Systems: Using Java, the project is to model the operation of the MMU (Memory Management Unit). Here are the specifics: -The virtual addresses are
Operating Systems:
Using Java, the project is to model the operation of the MMU (Memory Management Unit).
Here are the specifics:
-The virtual addresses are 16 bits.
-The memory uses a page size of 1K.
-The computer has 8 page frames.
Phase 1
For this phase, your MMU will have a function read, as follows:
// Int read(int virtualAddress);
This function is passed a 16-bit value, the virtual address coming in to the MMU. This function will return a 13-bit physical address, which is the output of the MMU unit. In addition, this function should print some messages showing what the MMU is doing. For example, one call to this function might print the following:
Output:
// Read is called with virtual address 0x43B7.
//..this becomes physical address 0x7B7.
Notice, by the way, that the addresses are printed in hex, which will make it easier for you to debug what is going on in the MMU. The function should also print some additional messages, for example, if there was a page fault:
Output:
// Read is called with virtual address 0x43B7.
//..page fault
//..loading data into page frame 1
//..this becomes physical address 0x7B7.
You will be implementing the First In First Out (FIFO) algorithm, which is is easy to code (the other phases use better algorithms). Your code should have a counter which gets incremented every time there was a page fault, when you have to load a new page into memory. In your page table, you will be storing these values:
- Page Frame Number
- Present/Absent Bit
- Time when loaded
The time when loaded field should be loaded from the counter. Suppose you are going to load Virtual Page 15 into Page Frame 2. In your Page Table, at index 15, you will store the value 2. You will set the Present bit for this entry as well. Finally, you will increment the counter, then store the new value into the LoadTime field for this entry.
When you get a page fault and need to load memory, first see if there are any unused Page Frames (they will all be unused at the start). If there is one, use it. But if all the frames are filled, you look through the page table for the page that is present, but has the smallest LoadTime value. This will be the page that was loaded the longest ago, so this is the page you will discard, reusing the page frame for the new page you are loading.
To complete this phase of the project, write some driver code that will make a series of calls to the read function. Design a sequence of calls that will demonstrate the correct operation of your implementation.
Phase 2
Augment your MMU to support memory writes as well, so your Page Table Entries will also need the M (modified) bit. Have a second function, write, which does the same translation code as the read function, but which will also set the M bit for the page. This will show that the page is dirty.
Change the page replacement algorithm to use the Not Recently Used algorithm, which gives much better performance than First In First Out. Note that this version does not have the R bit, it only has the M bit, so this is actually just a partial implementation of NRU(Not Recently Used Algorithm).
Now modify your driver code so the new sequence shows that the MMU is correctly implementing the Not Recently Used algorithm (this will involve making some write calls in addition to the read calls).
Phase 3
Make another change to the MMU so that it implements the LRU (Least Recently Used) algorithm. To do this, you will need to decide how to implement the algorithm and what changes you will need to make to the Page Table.
For reference, look at images attached,
Step by Step Solution
There are 3 Steps involved in it
Step: 1
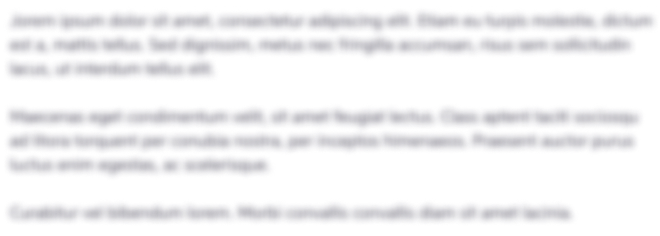
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started