Answered step by step
Verified Expert Solution
Question
1 Approved Answer
OS term project part 1: Where do i even start? How do i make a class to contain the data? How do I implement these
OS term project part 1: Where do i even start? How do i make a class to contain the data? How do I implement these functions? what are some actually good online resources?
Operating Systems CSCI 5806 Spring Semester 2020 CRN 21176 / 26762 Term Project Step 1 VDI File Access Target completion date: Friday, January 31, 2020 Goals Provide the five basic file 1/O functions to access disk space inside a VDI file. Create a structure or class to contain the data necessary to implement the five functions. Details You'll want a single entity a structure or a class, either works to represent a VDI file within your project. The intent is to collect the various data your project is going to need into one place for ease of use; if you're using C++ (you are using C or C++, right?) then a class can also contain the basic I/O functions as methods. What do you need to keep track of in a VDI file? To implement the five functions you'll probably want at least these four items to be contained in your structure: The file descriptor for the VDI file. This is an int. A VDI header structure. Discussion of the structure can be found at https://forums.virtualbox.org/viewtopic.php?t=8046; C structures for the header can be found in the VirtualBox source code. The VDI translation map. This is optional; include it if you are going to enable dynamic VDI files (they're easy!), ignore it otherwise. This is just an array of integers, although you don't know the size in advance. The size is given in the VDI header, so you'll need to allocate this dynamically. A cursor. This is just an integer (of type size_t) that holds the location of the next byte to be read or written. Once the structure / class is created, you'lIl need to implement a VDI version of each of the five basic I/O functions. struct VDIFile *vdi0pen (char *fn) Open the file whose name is given. The filename can be either a relative or absolute path. The function returns a pointer to a dynamically created VDI file structure (see above), or a null pointer if there was an error. The function should load the header and the translation map, set the cursor to 0 and set the file descriptor to whatever was returned from the open ( ) system call. If you're using a class, then this can return a boolean to indicate success or failure of the open. void vdiclose (struct VDIFile *f) Close the file whose pointer is given. Deallocate any dynamically created memory regions. VDI Access Operating Systems Spring 2020 CRN 21176 / 26762 ssize_t vdiRead (struct VDIFile *f,void *buf,size_t count) Reads the given number of bytes from the given VDI file's disk space, placing the bytes in the given buffer. The location of the first byte read is given by adding the cursor to the start of the VDI file's data space. The starting location is given in the VDI header. Advance the cursor by the number of bytes read. ssize_t vdiwrite(struct VDIFile *f,void *buf,size_t count) Writes the given number of bytes to the given file, starting at the cursor (plus the data start location). Bytes are written sequentially and the cursor is advanced to the end of the written block. Bytes to be written are located in the given buffer. off_t vdiseek (VDIFile *f,off_t offset,int anchor) Move the cursor of the given file to the given location, based on the offset and anchor values. If the resulting location is negative or larger than the disk size, do not change the value of the cursor. If you are using a class, then the VDIFile * parameter is omitted. You should also write a function that takes a pointer to a VDIFile as a parameter and displays its header fields in an easy-to-read manner. See example 1 below for a sample; your exact format may vary. Suggestions vdiSeek () should only set the cursor in the VDIFile structure; it should not call lseek( ). Reading and writing should be done one page at a time. While there are bytes left to be read or written, do the following tasks: 1. Determine where within the current page reading or writing should begin. 2. Determine how many bytes should be read or written in the current page. 3. Determine the physical location of the page. This may involve page translation and/or page allocation. Questions: What if the page is not allocated? What if the page is marked as "all zeroes"? 4. Use lseek ( ) to go to the proper location within the physical page. 5. Use read () or write() to read or write only the bytes within the current page. 6. Advance the cursor by the number of bytes read or written; subtract the number of bytes read or written from the number of bytes remaining. If you are planning to write into the filesystem, consider using the mmap ( ) and munmap ( ) func- tions to load the VDI file header and translation map. These act like a write-through cache; they read the areas from the file into memory set up by the OS, and writing to them automag- ically writes back to the file. Operating Systems CSCI 5806 Spring Semester 2020 CRN 21176 / 26762 Term Project Step 1 VDI File Access Target completion date: Friday, January 31, 2020 Goals Provide the five basic file 1/O functions to access disk space inside a VDI file. Create a structure or class to contain the data necessary to implement the five functions. Details You'll want a single entity a structure or a class, either works to represent a VDI file within your project. The intent is to collect the various data your project is going to need into one place for ease of use; if you're using C++ (you are using C or C++, right?) then a class can also contain the basic I/O functions as methods. What do you need to keep track of in a VDI file? To implement the five functions you'll probably want at least these four items to be contained in your structure: The file descriptor for the VDI file. This is an int. A VDI header structure. Discussion of the structure can be found at https://forums.virtualbox.org/viewtopic.php?t=8046; C structures for the header can be found in the VirtualBox source code. The VDI translation map. This is optional; include it if you are going to enable dynamic VDI files (they're easy!), ignore it otherwise. This is just an array of integers, although you don't know the size in advance. The size is given in the VDI header, so you'll need to allocate this dynamically. A cursor. This is just an integer (of type size_t) that holds the location of the next byte to be read or written. Once the structure / class is created, you'lIl need to implement a VDI version of each of the five basic I/O functions. struct VDIFile *vdi0pen (char *fn) Open the file whose name is given. The filename can be either a relative or absolute path. The function returns a pointer to a dynamically created VDI file structure (see above), or a null pointer if there was an error. The function should load the header and the translation map, set the cursor to 0 and set the file descriptor to whatever was returned from the open ( ) system call. If you're using a class, then this can return a boolean to indicate success or failure of the open. void vdiclose (struct VDIFile *f) Close the file whose pointer is given. Deallocate any dynamically created memory regions. VDI Access Operating Systems Spring 2020 CRN 21176 / 26762 ssize_t vdiRead (struct VDIFile *f,void *buf,size_t count) Reads the given number of bytes from the given VDI file's disk space, placing the bytes in the given buffer. The location of the first byte read is given by adding the cursor to the start of the VDI file's data space. The starting location is given in the VDI header. Advance the cursor by the number of bytes read. ssize_t vdiwrite(struct VDIFile *f,void *buf,size_t count) Writes the given number of bytes to the given file, starting at the cursor (plus the data start location). Bytes are written sequentially and the cursor is advanced to the end of the written block. Bytes to be written are located in the given buffer. off_t vdiseek (VDIFile *f,off_t offset,int anchor) Move the cursor of the given file to the given location, based on the offset and anchor values. If the resulting location is negative or larger than the disk size, do not change the value of the cursor. If you are using a class, then the VDIFile * parameter is omitted. You should also write a function that takes a pointer to a VDIFile as a parameter and displays its header fields in an easy-to-read manner. See example 1 below for a sample; your exact format may vary. Suggestions vdiSeek () should only set the cursor in the VDIFile structure; it should not call lseek( ). Reading and writing should be done one page at a time. While there are bytes left to be read or written, do the following tasks: 1. Determine where within the current page reading or writing should begin. 2. Determine how many bytes should be read or written in the current page. 3. Determine the physical location of the page. This may involve page translation and/or page allocation. Questions: What if the page is not allocated? What if the page is marked as "all zeroes"? 4. Use lseek ( ) to go to the proper location within the physical page. 5. Use read () or write() to read or write only the bytes within the current page. 6. Advance the cursor by the number of bytes read or written; subtract the number of bytes read or written from the number of bytes remaining. If you are planning to write into the filesystem, consider using the mmap ( ) and munmap ( ) func- tions to load the VDI file header and translation map. These act like a write-through cache; they read the areas from the file into memory set up by the OS, and writing to them automag- ically writes back to the file
Step by Step Solution
There are 3 Steps involved in it
Step: 1
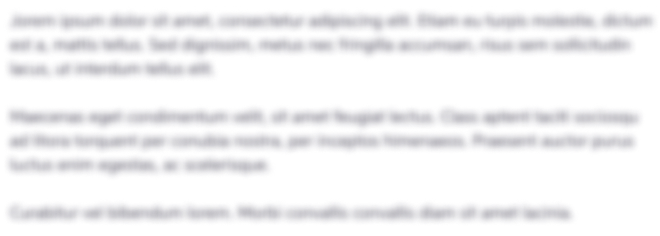
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started