Question
output is not sorting out my cards and asks for bid twice which I could not find where the error was at through code for
output is not sorting out my cards and asks for bid twice which I could not find where the error was at through code for a spades project. My code is showing up with cards in my hand but it is not sorting it by suit correctly. I am not sure what I have wrong. Screenshots will be below all the code. Need a response as soon as possible. I pasted all the code that is in the project. Please just help me asap as I need to turn this into class. Please any help
Instructions needed to follow:
Constants class | |
Update enum Face to include the following Two has the value of 2 Three has the value of 3 Four has the value of 4 Five has the value of 5 Six has the value of 6 Seven has the value of 7 Eight has the value of 8 Nine has the value of 9 Ten has the value of 10 Jack has the value of 11 Queen has the value of 12 King has the value of 13 Ace has the value of 14 Add field value of data type int Add a public getter to return the field value Add a private constructor for enum Face with one parameter of type int; set the field value to the parameter passed in Update enum Suit to include the following Clubs has the rank of 0 Diamonds has the rank of 1 Hearts has the rank of 2 Spades has the rank of 3 Add field rank of data type int Add a public getter to return the field rank Add a private constructor for enum Suit with one parameter of type int; set the field rank to the parameter passed in Example code: public enum Suit { CLUBS (0), DIAMONDS (1), HEARTS (2), SPADES (3);
private int rank;
public int getRank() { return rank; }
private Suit(int rank) { this.rank = rank; } } | |
core package | |
AiPlayer class | Update method placeBid() to do the following: Create a local variable of type int for the players bid Using an enhanced for loop, loop through the players hand (i.e. the ArrayList of class Card called hand) and increment the players bid based on the following logic for each card in the players hand:If, the face of the card is equal to an ACE or KING, increment the bid by one Else if, the suit of the card is equal to SPADES and the face value of the card is greater than TEN, increment the bid by one (1) If the bid counter is equal to zero(0), then by default set it equal to one (1) Call method .setBid() on the super class passing as an argument the local variable defined in step a. Return the bid by calling method .getBid() on the super class |
Game class | Update the class to do the following: Custom constructor Game()Comment out the call to method outputTeams() Add call to method play() Update method setTable() to do the following:Using an enhanced for loop output the names of each Player in the member variable table representing the setup of the players seated at the table Update method dealHand() to comment out any System.out.println() statements that show what card each player is being dealt if they exist Update method displayHands() to do the following:Call method .sortBySuit() for each player Only call method .displayHand() if the player is an instanceof class HumanPlayer Create method play() to do the following:Return type of void Empty parameter list Call method getBids() Create method getBids() to do the following:Return type of void Empty parameter list Create a local variable of data type int representing the number of bids placed initialized to the value of zero (0) Create a local variable of data type int to represent the index of the lead player, not initialized Using an if condition, check if the member value of the dealerIdx if less than 3If true then set the lead player index created in step d. equal to the dealerIdx plus one Else set the lead player index to zero(0) Set the member variable of class Player representing theleaderPlayerequal to method call .get() of the member variable tablepassing the lead player index as an argument Using an if condition, check if the leaderPlayer member variable is an instanceof class HumanPlayerIf true, create an instance of class HumanPlayer set equal to an explicit type cast of the member variable leadPlayer and call method .placeBid() on class HumanPlayer Else, create an instance of class AiPlayer set equal to an explicit type cast of the member variable leadPlayer and call method .placeBid() on class AiPlayer Increment the bids placed counter by one Create a local variable of data type int to represent the current player index, not initialized Using an if condition check if the lead player index is less than 3If true, set the player index equal to the lead player index plus one (1) Else, set the player index equal to the value of zero (0) Instantiate an instance of class Player set equal to the method call .get() on member variable table passing as an argument the player index created in step i. to get the nextPlayer While the number of bids submitted is less or equal to the number of players (i.e. 4)Write an if condition to determine if the local variable nextPlayer is an instanceof class HumanPlayerIf true, instantiate an instance of class HumanPlayer set equal to an explicit type cast of local variable nextPlayer and call method .placeBid() Else, instantiate an instance of class AiPlayer set equal to an explicit type cast of local variable AiPlayer set equal to an explicit type cast of local variable nextPlayer an call method .placeBid() Increment the bids placed counter Write an if condition to check if the value of the local variable for the player index is less than 3If true, increment the player index variable by one (1) Else, set the player index variable to zero (0) Set the local variable representing the nextPlayer equal to method call .get() on member variable table passing the player index as an argument |
HumanPlayer class | Update method placeBid() to do the following: Request the players bid using a System.out.println() method call Instantiate an instance of class Scanner passing System.in as an argument to the constructor Create a local variable of data type in set equal to the method call .nextInt() on the instance of class Scanner created in step b. Using a for condition, check if the inputted bid value is less than one (1), if true, output to the user they must bid at least one trick and set the local variable to the value of one (1) Call method .setBid() on the super class passing as an argument the local variable defined in step c. Return the bid by calling method .getBid() on the super class |
Player class | Update class to include the following: Add method sortBySuit() to do the following:Instantiate an instance of class ArrayList allowing only for instances of class Card to be elements that represents the sorted hand Loop while the size of member variable hand is greater than zero (0)Create a local variable of type int representing the current position in the ArrayList of member variable hand, initialize it to zero (0) Instantiate and instance of class Card (reference as firstCard) set equal to the first element in the ArrayList of member variable hand (hint: use the .get() method of class ArrayList) Using a for loop, starting at index 1, loop through the size of member variable ArrayList hand to do the following:Instantiate an instance of class Card (reference as nextCard) set equal to the next element in the ArrayList hand (i.e. index 1) Write an if statement to sort the cards based on suit and face value ranking with the following logic:If the suits rank of the nextCard in the hand is less than the suits rank of the firstCard in the hand ORThe suit of the nextCard is equal to the suit of the firstCard AND The face value of the nextCard in the hand is less than the face value of the firstCard in the handUpdate local variable position to equal the for loops looping variable Set the firstCard equal to the nextCard Remove from the member variable hand the card at the current position (hint: on member variable hand call method .remove() passing the argument defined in step i.) Add the instance represented as firstCard to the local ArrayList that represents the sorted hand created in step a. Set member variable hand equal to the local ArrayList that represents the sorted hand. |
Images of my Code are below:
Constants:
package constants;
/** * * @author Matth */
public class Constants { //Initiated constants with all caps and non changeable values public static final int AI_PLAYERS = 3; public static final int NUMBER_OF_CARDS = 52; public static final int CARDS_DEALT = 13; public static final int NUMBER_OF_ROUNDS = 13; public static final int BID_VALUE = 1; public static final int WINNING_SCORE = 200; public static final int ONE = 0; public static final int TWO = 1; public static final int NUMBER_OF_PLAYERS = 4; //Created enumerations for color, suit and face of each card. public enum Color { RED,BLACK } public enum Suit { CLUBS(0), DIAMONDS(1), HEARTS(2), SPADES(3); private int rank; public int getRank() { return rank; } private Suit(int rank) { this.rank = rank; } } public enum Face { TWO(2), THREE(3), FOUR(4), FIVE(5), SIX(6), SEVEN(7), EIGHT(8), NINE(9), TEN(10), JACK(11), QUEEN(12), KING(13), ACE(14); private int value; public int getValue() { return value; } private Face(int value) { this.value = value; } } }
AiPlayer Class:
package core;
import constants.Constants; import constants.Constants.Face; import constants.Constants.Suit;
/** * * @author Matth */
//Created a public class AiPlayer so it extends the abstract class player public class AiPlayer extends Player{ //implements methods inherited from class player. @Override public Card playCard(){ return null; } @Override public int placeBid() { System.out.println(super.getName() + " place your bid"); int bid = 0; //The AI will assume that if they have an Ace or Kind of any suit they will get the //The AI will assume that if they have the Jack or Queen of spades they will get the for(Card card : super.getHand()) { //Chooses the Ace and King of any suit if(card.getFace() == Face.ACE || card.getFace() == Face.KING) { bid++; } //Only chooses Jack and Queen of Spades //If player already received King or Ace It chooses Jack and Queen of Spades. else if(card.getSuit() == Suit.SPADES && card.getFace().getValue() > Face.TEN.getValue()) { bid++; } } if (bid == 0) bid = 1; super.setBid(bid); System.out.println("Player " + this.getName() + " bid " + bid); return super.getBid(); } }
Game Class:
package core;
/** * * @author Matth */
import constants.Constants; import constants.Constants.Suit; import java.util.ArrayList; import java.util.Iterator; import java.util.Random; import java.util.Scanner;
public class Game { //Member Variables private Suit trump = Suit.SPADES; private Player leadPlayer; private Player dealer; private Player wonTrick; private int round; private ArrayList teams; private Deck deck; private Scanner scan; private ArrayList table; private int dealerIdx; //getters and setters for remainding member variables. /** * @return the deck */ public Deck getDeck() { return deck; }
/** * @param deck the deck to set */ public void setDeck(Deck deck) { this.deck = deck; }
/** * @return the leadPlayer */ public Player getLeadPlayer() { return leadPlayer; }
/** * @param leadPlayer the leadPlayer to set */ public void setLeadPlayer(Player leadPlayer) { this.leadPlayer = leadPlayer; }
/** * @return the dealer */ public Player getDealer() { return dealer; }
/** * @param dealer the dealer to set */ public void setDealer(Player dealer) { this.dealer = dealer; }
/** * @return the wonTrick */ public Player getWonTrick() { return wonTrick; }
/** * @param wonTrick the wonTrick to set */ public void setWonTrick(Player wonTrick) { this.wonTrick = wonTrick; }
/** * @return the round */ public int getRound() { return round; }
/** * @param round the round to set */ public void setRound(int round) { this.round = round; } //Custom constructor that calls method createTeams/outputTeams/generateDeck public Game() { createTeams(); //outputTeams(); createDeck(); setTable(); dealHand(); displayHands(); //Calls out play(); Method. play(); }
//Create Deck Method to initialize classes variable. private void createDeck() { //Initialize classes variable deck = new Deck(); } private void play() { System.out.println("**************"); System.out.println("Play the game!"); System.out.println("************** "); System.out.println("***************"); System.out.println("Get player bids"); System.out.println("***************"); //Starting with the player to the left of the dealer //Each player plays their best card. //Players must follow suit if they can, if not they trump if needed. getBids(); } private void getBids() { //Amount of bids placed int bidsPlaced = 0; //Setting up the lead player int leadIdx; if(dealerIdx
HumanPlayer Class:
package core;
import java.util.Scanner;
/** * * @author Matth */
//Created a public class HumanPlayer so it extends the abstract class player public class HumanPlayer extends Player{ @Override public Card playCard(){ throw new UnsupportedOperationException("Not supported yet."); } @Override public int placeBid() { System.out.println(super.getName() + "place your bid"); Scanner scanner = new Scanner(System.in); int bid = scanner.nextInt(); if(bid
Player Class:
package core;
import java.util.ArrayList;
/** * * @author Matth */ public abstract class Player implements IPlayer{
//member variables private String name; private int tricks; private int bid; private int score; private ArrayList hand; //Abstract methods. @Override public abstract Card playCard(); @Override public abstract int placeBid(); /** * @return the hand */ public ArrayList getHand() { return hand; }
/** * @param hand the hand to set */ public void setHand(ArrayList hand) { this.hand = hand; }
public Player () { hand = new ArrayList(); } /** * @return the name */ public String getName() { return name; }
/** * @param name the name to set */ public void setName(String name) { this.name = name; }
/** * @return the tricks */ public int getTricks() { return tricks; }
/** * @param tricks the tricks to set */ public void setTricks(int tricks) { this.tricks = tricks; }
/** * @return the bid */ public int getBid() { return bid; }
/** * @param bid the bid to set */ public void setBid(int bid) { this.bid = bid; }
/** * @return the score */ public int getScore() { return score; }
/** * @param score the score to set */ public void setScore(int score) { this.score = score; } public void displayHand() { System.out.println("**************************"); System.out.println("Player" + name + " hand is "); System.out.println("**************************"); for(Card card : hand) { System.out.println(card.getFace() + " of " + card.getSuit()); } } public void sortBySuit() { //Sets up the cards into increasing sorted value. //Cards with same value end up being sorted by suit. ArrayList sortedHand = new ArrayList(); while (hand.size() > 0) { int position = 0; Card firstCard = hand.get(0); for (int i = 1; i
Deck Class:
package core;
import java.util.Set; import constants.Constants; import constants.Constants.Color; import constants.Constants.Face; import constants.Constants.Suit; import java.util.ArrayList; import java.util.Collections; import java.util.HashSet; import java.util.List;
/** * * @author Matth */ public class Deck { //added to represent the deck of cards. private Set deck; //Constructor for Method declaration //and print line *s to seperate and label each method. public Deck(){ System.out.println("****************************"); generateDeck(); System.out.println("****************************"); System.out.println("Displaying the deck of cards"); System.out.println("****************************"); displayDeck(); System.out.println("***************************"); System.out.println("Shuffling the deck of cards"); System.out.println("***************************"); shuffleDeck(); System.out.println("*************************************"); System.out.println("Displaying the shuffled deck of cards"); System.out.println("*************************************"); displayDeck(); } //Adding getters and setters for private Set deck; /** * @return the deck */ public Set getDeck() { return deck; }
/** * @param deck the deck to set */ public void setDeck(Set deck) { this.deck = deck; }
//Created to loop through values of enum face and states the color and value //else it doesn't exist and gets converted back. private void generateDeck() { deck = new HashSet(Constants.NUMBER_OF_CARDS); for(Face face : Face.values()) { for(Suit suit : Suit.values()) { Card card = new Card(); card.setFace(face); card.setSuit(suit); if(suit == Suit.DIAMONDS || suit == Suit.HEARTS) card.setColor(Color.RED); else card.setColor(Color.BLACK); if(!deck.contains(card)) deck.add(card); }//inner for loop }//outter for loop } //Iterates through collection of cards and outputs to console private void displayDeck() { System.out.println("Deck size:" + deck.size() + " cards"); System.out.println("Deck includes:"); for(Card card : deck) { System.out.println("Card: " + card.getFace() + " of " + card.getSuit() + " is color " + card.getColor()); } } private void shuffleDeck() { //Converts an ArrayList out of the HashSet... List cardList = new ArrayList(deck); //Shuffles the deck of cards using cardList ArrayList Collections.shuffle(cardList); deck = new HashSet(cardList); } }
Card Class:
package core;
/** * * @author Matth */ //Explicitly imports each enumeration from Constants. import constants.Constants.Color; import constants.Constants.Face; import constants.Constants.Suit;
public class Card { //added getters and setters for the member variables below. /** * @return the face */
public Face getFace() { return face; }
/** * @param face the face to set */ public void setFace(Face face) { this.face = face; }
/** * @return the suit */ public Suit getSuit() { return suit; }
/** * @param suit the suit to set */ public void setSuit(Suit suit) { this.suit = suit; }
/** * @return the color */ public Color getColor() { return color; }
/** * @param color the color to set */ public void setColor(Color color) { this.color = color; } private Face face; private Suit suit; private Color color; //Created Class HashCode to use Deck classes import Hashset. @Override public int hashCode() { int hashCode = 0; return hashCode; } //Created public boolean to receive one parameter of Object data type. @Override public boolean equals(Object obj) { //While checking if the parameter to an instance of class Card. //and returning the result of comparing if the face,suit and card match. if (obj instanceof Card) { Card card = (Card) obj; return (card.face.equals(this.face) && card.color.equals(this.color)&& card.suit.equals(this.suit)); } else //Else nothing happens and returns false. { return false; } } }
Team Class:
package core;
import java.util.ArrayList; import java.util.Scanner; import constants.Constants;
/** * * @author MatthewS */ public class Team { //Member variables private final ArrayList team; private int teamBid; private int teamScore; private int teamTricks; private int teamBags; private Scanner scan; private String teamName; //custom constructor public Team() { team = new ArrayList(); } //Getters and setters for member variables /** * @return the team */ public ArrayList getTeam() { return team; }
/** * @return the teamBid */ public int getTeamBid() { return teamBid; }
/** * @param teamBid the teamBid to set */ public void setTeamBid(int teamBid) { this.teamBid = teamBid; }
/** * @return the teamScore */ public int getTeamScore() { return teamScore; }
/** * @param teamScore the teamScore to set */ public void setTeamScore(int teamScore) { this.teamScore = teamScore; }
/** * @return the teamTricks */ public int getTeamTricks() { return teamTricks; }
/** * @param teamTricks the teamTricks to set */ public void setTeamTricks(int teamTricks) { this.teamTricks = teamTricks; }
/** * @return the teamBags */ public int getTeamBags() { return teamBags; }
/** * @param teamBags the teamBags to set */ public void setTeamBags(int teamBags) { this.teamBags = teamBags; }
/** * @return the teamName */ public String getTeamName() { return teamName; }
/** * @param teamName the teamName to set */ public void setTeamName(String teamName) { this.teamName = teamName; }
}
Spades Class:
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package spades;
import core.Deck; import core.Game; import javax.swing.JOptionPane; /** * * @author MatthewS */ public class Spades {
/** * @param args the command line arguments */ public static void main(String[] args) { // TODO code application logic here //Created a println that outputs Welcome to Spades! //While outputting a dialog box that states Let's Play Spade! System.out.println("Welcome to Spades!"); JOptionPane.showMessageDialog(null,"Lets Play Spades!"); //instantiate instance of class game Game Game = new Game(); } }
My output is not sorting out my cards and asks for bid twice which I could not find where the error was at through code. Screenshots for what I am talking about will be below Here:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
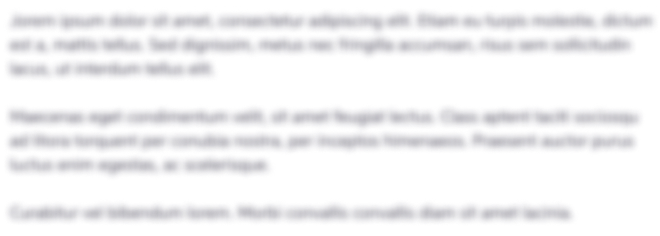
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started