Question
Overloading and Templates Overloading the operators < >, =, +, , ==, ||, and && for the class lineType BACKGROUND: -Equation of a line: ax+by=c
Overloading and Templates Overloading the operators <<, >>, =, +, , ==, ||, and && for the class lineType BACKGROUND: -Equation of a line: ax+by=c is the standard equation for a line, where a and b cannot both be zero, and where a, b, and c are real numbers. -Slope of a line: When b != 0 the slope of a line is a/b. When b=0, the line is vertical, and the slope is undefined. When a=0, the line is horizontal, and the slope is zero. -Parallel Lines: Two lines are parallel when they have the same slope, or are both vertical. -Perpendicular Lines: Two lines are perpendicular when one is horizontal and the other vertical, or when the product of their slopes is 1. PROVIDED CLASS lineType -Stores a line: * stores a, the coefficient of x * stores b, the coefficient of y * stores c -Performs operations: * Determines slope of line, if line is non-vertical. * Determines if two lines are equal. * Determines if two lines are parallel. * Determines if two lines are perpendicular. * Determines point of intersection of two lines, if they are not parallel. Tasks: DOWNLOAD zip files corresponding to the class lineType from Blackboard, review the code, and gain an understanding of how it works. MODIFY class lineType to use overloaded operators as follows: Overload the stream insertion operator, <<, for output. Overload the stream extraction operator, >>, for input. * The input should be (a,b,c) for line output should be ax+by=c. Overload the assignment operator, =, to copy a line into another line. Overload the unary operator + so that it returns true if a line is vertical and false if the line is not vertical. Overload unary operator - so that it returns true if a line is horizontal and false if the line is not horizontal. Overload the operator == so that it returns true if two lines are equivalent and false if the two lines are not equivalent. * Two lines are equivalent when a1 =ka2, b1 =kb2, and c1 =kc2 for any real number k. Overload the operator || so that it returns true if two lines are parallel and false if the two lines are not parallel. Overload the operator && so that it returns true if two lines are perpendicular and false if the two lines are not perpendicular. Submit a single cpp file. #include #include #include using namespace std; //----------------------DriverProgram----------------------- int main() { lineType line1(2, 3, 4); lineType line2(3, 5, 7); lineType line3(2, 3, -2); lineType line4(3, -2, 1); lineType line5(2, 0, 3); //vertical line lineType line6(0, -1, 2); //horizontal line lineType line7(4, 6, 8); lineType line8; cout << "line1: " << line1 << endl; if (!(+line1)) cout << "The slope of line1: " << line1.slope() << endl; else cout << "line1 is a vertial line. Its slope is undefined." << endl; cout << "line2: " << line2 << endl; cout << "line3: " << line3 << endl; cout << "line4: " << line4 << endl; cout << "line5: " << line5 << endl; cout << "line6: " << line6 << endl; cout << "line7: " << line7 << endl; if (line1 || line2) cout << "line1 and line2 are parallel." << endl; else cout << "line1 and line2 are not parallel." << endl; line1.pointOfIntersection(line2); if (line1 || line3) cout << "line1 and line3 are parallel." << endl; else cout << "line1 and line3 are not parallel." << endl; if (line1 && line4) cout << "line1 and line4 are perpendicular." << endl; else cout << "line1 and line4 are not perpendicular." << endl; if (+line5) cout << "line5 is a vertical line." << endl; else cout << "line5 is not a vertical line." << endl; if (-line6) cout << "line6 is a horizontal line." << endl; else cout << "line6 is not a horizontal line." << endl; line5.pointOfIntersection(line6); if (line1 == line7) cout << "line1 and line 7 are equal." << endl; else cout << "line1 and line 7 are not equal." << endl; line1.pointOfIntersection(line7); cout << "Input line8 in the form (a, b, c): "; cin >> line8; cout << line8 << endl; if (line1 || line8) cout << "line1 and line8 are parallel." << endl; else cout << "line1 and line8 are not parallel." << endl; return 0; } class lineType { public: void setLine(double a = 0, double b = 0, double c = 0); //Function to set the line. void equation() const; double getXCoefficient() const; double getYCoefficient() const; double getCOnstantTerm() const; void setXCoefficient(double coeff); void setYCoefficient(double coeff); void setConstantTerm(double c); double slope() const; //Return the slope. This function does not check if the //line is vartical. Because the slope of a vertical line //is undefined, before calling this function check if the //line is nonvertial. bool verticalLine() const; bool horizontalLine() const; bool equalLines(lineType otherLine) const; //Returns true of both lines are the same. bool parallel(lineType otherLine) const; //Function to determine if this line is parallel to otherLine. bool perpendicular(lineType otherLine) const; //Function to determine if this line is perperdicular to otherLine. void pointOfIntersection(lineType otherLine); //If lines intersect, then this function finds the point //of intersection. lineType(double a = 0, double b = 0, double c = 0); //Constructor private: double xCoeff; double yCoeff; double constTerm; bool hasSlope; }; //Main program #include #include "lineType.h" using namespace std; int main() { lineType line1(2, 3, 4); lineType line2(3, 5, 7); lineType line3(2, 3, -2); lineType line4(3, -2, 1); lineType line5(2, 0, 3); //vertical line lineType line6(0, -1, 2); //horizontal line lineType line7(4, 6, 8); cout << "line1: "; line1.equation(); cout << endl; if (!line1.verticalLine()) cout << "The slope of line1: " << line1.slope() << endl; else cout << "line1 is a vertial line. Its slope is undefined." << endl; cout << "line2: "; line2.equation(); cout << endl; cout << "line3: "; line3.equation(); cout << endl; cout << "line4: "; line4.equation(); cout << endl; cout << "line5: "; line5.equation(); cout << endl; cout << "line6: "; line6.equation(); cout << endl; cout << "line7: "; line7.equation(); cout << endl; if (line1.parallel(line2)) cout << "line1 and line2 are parallel." << endl; else cout << "line1 and line2 are not parallel." << endl; line1.pointOfIntersection(line2); if (line1.parallel(line3)) cout << "line1 and line3 are parallel." << endl; else cout << "line1 and line3 are not parallel." << endl; if (line1.perpendicular(line4)) cout << "line1 and line4 are perpendicular." << endl; else cout << "line1 and line4 are not perpendicular." << endl; if (line5.verticalLine()) cout << "line5 is a vertical line." << endl; else cout << "line5 is not a vertical line." << endl; if (line6.horizontalLine()) cout << "line6 is a horizontal line." << endl; else cout << "line6 is not a horizontal line." << endl; line5.pointOfIntersection(line6); if (line1.equalLines(line7)) cout << "line1 and line 7 are equal." << endl; else cout << "line1 and line 7 are not equal." << endl; line1.pointOfIntersection(line7); return 0; } #include #include #include #include "lineType.h" using namespace std; void lineType::setLine(double a, double b, double c) { xCoeff = a; yCoeff = b; if (a == 0 && b == 0) constTerm = 0; else constTerm = c; } void lineType::equation() const { cout << xCoeff << "x "; if (yCoeff < 0 ) cout << "- "; else cout << "+ "; cout << fabs(yCoeff) << "y = " << constTerm << endl; } double lineType::getXCoefficient() const { return xCoeff; } double lineType::getYCoefficient() const { return yCoeff; } double lineType::getCOnstantTerm() const { return constTerm; } void lineType::setXCoefficient(double coeff) { xCoeff = coeff; } void lineType::setYCoefficient(double coeff) { yCoeff = coeff; } void lineType::setConstantTerm(double c) { constTerm = c; } double lineType::slope() const { return - xCoeff / yCoeff; } bool lineType::verticalLine() const { if (yCoeff == 0) return true; else return false; } bool lineType::horizontalLine() const { if (xCoeff == 0) return true; else return false; } bool lineType::equalLines(lineType otherLine) const { //Note that lines such as 2x + 3y = 1 and 4x + 6y = 2 //are also equal. double factor = 1; if (xCoeff != 0) factor = otherLine.xCoeff / xCoeff; else factor = otherLine.yCoeff / yCoeff; if (xCoeff * factor == otherLine.xCoeff && yCoeff * factor == otherLine.yCoeff && constTerm * factor == otherLine.constTerm) return true; else return false; } bool lineType::parallel(lineType otherLine) const { if (yCoeff == 0 && otherLine.yCoeff == 0) return true; else if ((yCoeff != 0 && otherLine.yCoeff == 0) || (yCoeff == 0 && otherLine.yCoeff != 0)) return false; else if ((xCoeff / yCoeff) == (otherLine.xCoeff / otherLine.yCoeff)) return true; else return false; } bool lineType::perpendicular(lineType otherLine) const { if (yCoeff != 0 && otherLine.yCoeff != 0) { if ((xCoeff / yCoeff) * (otherLine.xCoeff / otherLine.yCoeff) == -1.0) return true; else return false; } else if ((xCoeff == 0 && otherLine.yCoeff == 0) || (yCoeff == 0 && otherLine.xCoeff == 0)) return true; else false; } void lineType::pointOfIntersection(lineType otherLine) { if (equalLines(otherLine)) cout << "Both lines are equal. They have infinite " << "points of intersections." << endl; else if (parallel(otherLine)) cout << "Lines do not intersect. No point intersection." << endl; else cout << "The point of intersection is: (" << (constTerm * otherLine.yCoeff - yCoeff * otherLine.constTerm) / (xCoeff * otherLine.yCoeff - yCoeff * otherLine.xCoeff) << ", " << (constTerm * otherLine.xCoeff - xCoeff * otherLine.constTerm) / (yCoeff * otherLine.xCoeff - xCoeff * otherLine.yCoeff) << ")" << endl; } lineType::lineType(double a, double b, double c) { setLine(a, b, c); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
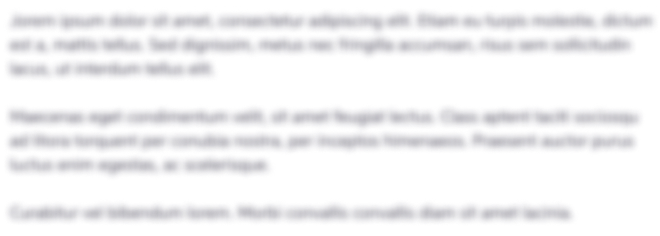
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started