Question
Overview Design, implement, and test a class that returns various statistics on a given sequence of values. Please read this entire document before you get
Overview
Design, implement, and test a class that returns various statistics on a given sequence of values.
Please read this entire document before you get started.
Please read the Emacs/UNIX Commands for this Assignment section at the end of this document and practice using these tools during this assignment. These are programming tools that you should use constantly everyday during this course.
Discussion
Here is a description of a class of object that you must design:
-
The class must have a method that allows a single floating-point value to be entered into the data set that various statistics will be genereated from. This data-entry method (i.e. function) must take a single argument of type double, which represents one value in the sequence, and add this value to the data stored in the class.
This method can then be called on an object of this type to add a single data point to that particular object.
Since the user may enter any number of values into the sequence of values that statistics will be generated over, this method can be called any number (i.e. an unknowable number) of times. (That is, this method can and should be called from within a loop.)
After the class has received a sequence of values (using repeated calls to the method above), the class must supply methods that will generate the following statistics over the values in that data sequence:
-
Find N: the number of values in the sequence
-
Find the value of the last number in the sequence
-
Find the sum of all of the values in the sequence
-
Find the arithmetic mean of all of the values in the sequence. The arithmetic mean is given by:
-
Find the minimum (smallest) value in the sequence
-
Find the maximum (largest) value in the sequence
-
Clear the sequence so that statistics can be calculated on a new sequence of values
Each of these methods must be implemented in the class as a separate method that will return (supply) that statistic to the client of this class (a classs client is the code that uses that class, in this case, a main() function).
As you specify this class, be sure that you:
-
Give the class and the classs methods appropriate names (notice that you have not been told what to call anything)
-
Give the class a default constructor that initializes all data members to appropriate values
-
Give the classs methods appropriate operation contracts (more on this below)
Note
The method that returns the length of the sequence can be called on an object that has no values in the sequence. The length of an empty sequence is zero.
The same holds for the sum of the values in the sequencethe sum of an empty sequence is zero.
Also, an empty sequence can be cleared of all of its valuesnothing changes.
Note
However, none of the other methods of the class can be called on empty sequences. These operations should document that they cannot be called on empty sequences in their operation contracts, and they should protect against being executed if they are called on an empty sequence. Think about how you might do this, but you are not required to implement this behavior. We will discuss techniques for handling this type of situation later in the course.
The operation contracts (specifications) must be written in your class definition (in the header file) using JavaDoc-style comments. Please read Appendix I in the text on pages 809810 for details. You should also read the operation contract in the JavaDoc-style comment found in the BagInterface class template found on pages 2425 as a guide.
Implementation Requirements
Here are further requirements of the class you are to create:
-
You must write a class definition in a header file that has proper preprocessor guards. All methods must be declared in this class definition (do not implement any of them hereinline methods cannot be used).
-
You must write a separate implementation file where all methods declared in the class definition are implemented.
-
Your testing code must be found in a separate implementation (driver) file.
-
Your classs data members must all be declared private to the class.
Implementation Hint
Since you have no way of knowing how many values a user might supply in a sequence, you should not try to independently store all of the values in the sequence (i.e. do NOT use an array or any other container to store all of the data values). Rather, as new values are entered into the sequence, store only those values that are required to generate correct output for all the required operations given. Use the descriptions of what is required of this class to determine the data requirements, i.e. what or how the data is stored.
Note
This requires some thought. What data must an object of this type store in order to meet the functional requirements of its methods, without storing all of the data individually?
Look at each required method individually and think about what it requires (in terms of data storage) in order for it to execute its task.
Testing
To test your class, you must write a driver (i.e. a main() function that tests all aspects of your code). Part of your final grade will be based on how well you test your implementation. You should use these guidelines as you develop your testing routine:
-
Write tests that cause every line of code in your class to be executed. This means that every branch in every if or switch statement is executed and every loop is entered.
-
When code may not execute, then be sure that a test is written in such a way that that code does not execute. This means that a loop that may never execute its body should have a test written so that its body does not execute (i.e. that loop does not iterateeven once).
-
Tests should be written to test all functionality on varying data sequences:
-
An empty sequence of values
-
A single value sequence
-
An increasing sequence of values (e.g. {-2.1, 0.4, 3.8})
-
A decreasing sequence of values (e.g. {1.1, -0.5, -2.0})
-
A random sequence of values (e.g. {4.8, -2.6, 3.9})
-
A constant sequence of values (e.g. {1.4, 1.4, 1.4})
-
Other sequences of interest (e.g. a sequence that sums to zero)
Please note that these sequences should have varying lengths. The 3-value sequences given above are only simple examples.
-
Step by Step Solution
There are 3 Steps involved in it
Step: 1
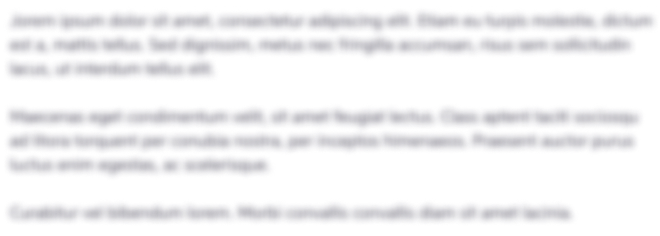
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started