Question
Overview: file you have to complete is WordTree.h , WordTree.cpp, main.cpp Write a program in C++ that reads an input text file and counts the
Overview: file you have to complete is WordTree.h, WordTree.cpp, main.cpp
Write a program in C++ that reads an input text file and counts the occurrence of individual words in the file. You will see a binary tree to keep track of words and their counts.
Project description:
The program should open and read an input file (named input.txt) in turn, and build a binary search tree of the words and their counts. The words will be stored in alphabetical order in the tree. The program should ignore the case of the words, so that Branch and branch are considered the same. However, words that are actually spelled differently such as tree and trees are considered to be different, and should be stored in separate nodes. All words will be stored in the tree in their lowercase form. Help is provided on how to accomplish this.
Two forms of queries are to be supported by the tree class:
A query for an individual word should return the number of occurrences of that word in the input file, as retrieved from searching the tree, or should display a message stating that the word was not found in the tree.
A query for words that meet or exceed a threshold number of occurrences, should result in the output of the words (and their counts) that meet that criteria, using inorder tree traversal.
The nodes for the word tree should be a struct with the following members
A string containing the word itself.
One pointer each for the left and right subtrees.
An int containing the count of the number of occurrences of the word.
Requirements:
Your program must be split into 3 files. There will be a class (with the separate interface and implementation files), and a driver file. The requirements for these are specified below:
file must be named WordTree.h and WordTree.cpp
The WordTree class This class represents a word binary search tree
Class must be named WordTree
You should implement the following member functions in the class
A constructor creates an empty tree
A destructor recursive function that explicitly releases all nodes allocated during program execution. You may not rely no program termination to release memory.
insert Recursive function that adds a word to the tree, if it is not found, or increments its count if it is already in the tree.
findNode accepts a string argument (a word) and searches for the word in the tree. This function should be public, but should call a private function, so as to not expose the trees root, its implementation, etc. If found, outputs the word and its count. Otherwise displays a message stating that the word was not found.
printInOrder Recursive function that accepts a single integer argument (a threshold value), and traverses that tree in order, outputting the words (and their counts) that meet or exceed the threshold count. The function should also output the number of nodes meeting the criteria (see sample output).
2. A driver, or client, file that
Must be named proj6.cpp
Instantiates the word tree object
Opens and reads the text file named input.txt and builds the word tree from the file by invoking the insert function described above. The input files should contain a single line of alphabetic characters (no numbers or punctuation). It should be split using a single space character via the provided function or one you write. Care should be taken to ensure that multiple sequential spaces and trailing spaces are not present in the file/line to be read. Example file: This is the whole file and is stored and read as a single line into a string
IF YOU NEED A HELP USE THIS
-------------------- code to transform string to lowercase //transforms a string to lowercase - must include// text is a string std::transform(text.begin(), text.end(), text.begin(), ::tolower); -------------------- function to split a string with a delimiter and load individual tokens into a string vector // From book "C++ Cookbook" by Jeff Cogswell, Jonathan Turkanis, Christopher Diggins, D. Ryan Stephens // Splits a string s, using character c as a delimeter, and places individual words into a vector of strings void split(const std::string& s, char c, std::vector<:string>& v) { std::string::size_type i = 0; std::string::size_type j = s.find(c); while (j != std::string::npos) { v.push_back(s.substr(i, j-i)); i = ++j; j = s.find(c, j); if (j == std::string::npos) v.push_back(s.substr(i, s.length())); } }
Invokes queries for individual words, or for words whose counts meet or exceed a threshold number of occurrences, as shown in the sample output below.
TESTING FILE:
Project description The program should open and read input file named input.txt in turn building up a binary search tree of words and counts as it progresses The words will be stored in alphabetical order in the tree The program should ignore the case of the words so that Branch and branch are considered the same However words that are actually spelled differently such as tree and trees are considered to be different and should be stored in separate nodes All words will be stored in the tree in their lowercase form Two forms of queries are to be supported by the tree class A query for an individual word should return the number of occurrences of that word in the input file as retrieved from searching the tree or should display a message stating that the word was not found in the tree A query for words that meet or exceed a threshold number of occurrences should result in the output of the words and their counts that meet that criteria using inorder tree traversal The nodes for the word tree should be a struct with the following members One pointer each to the left and right subtrees An int containing the count of the number of occurrences of the word A string containing the word itself Requirements Your program must be split into files There will be a class with separate interface and implementation files and a driver file The requirements for these are specified below The WordTree class This class represents a Word Binary Tree Files must be named WordTree.h and WordTree.cpp Class must be named WordTree You should implement the following member functions in the class A constructor Creates an empty tree A destructor Recursive function that explicitly releases all nodes allocated during program execution You may not rely on program termination to release memory insert Recursive function that adds a word to the tree if it is not found or increments its count if it is already in the tree findNode accepts a string argument a word and searches for the word in the tree If found outputs the word and its count Otherwise displays a message stating that the word was not found printInOrder Recursive function that accepts a single integer argument a threshold value and traverses the tree in order outputting the words and their counts that meet or exceed the threshold count The function should also output the number of nodes meeting the criteria A driver or client file that Must be named proj6.cpp Declares the word tree object Opens and reads the text file named input.txt and builds the word tree from the file by invoking the insert function described above Invokes queries for individual words or for words whose counts meet or exceed a threshold number of occurrences
ord tree ilt an oade Finding all words with 4 or more occurrences: a(20 and 16 are in(13 meet 4> nust 4> named 5 nodes 4) ot occurrences(4) of that 13 the (45 threshold to 5 tree Searchin for occurrences of the word query' The word query occurs 2 time> in the text. Searching for occurrences of the word ' stackStep by Step Solution
There are 3 Steps involved in it
Step: 1
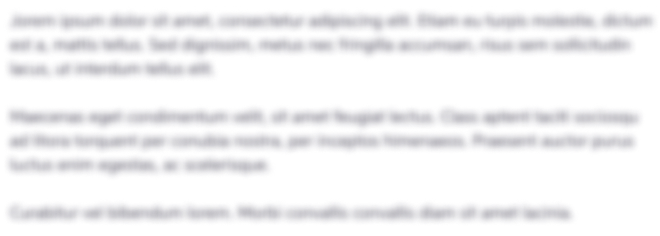
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started