Question
Overview In this assignment, the student will write a program that cleans a text file of target characters to prepare it for encryption with the
Overview
In this assignment, the student will write a program that cleans a text file of target characters to prepare it for encryption with the Affine Cipher.
When completing this assignment, the student should demonstrate mastery of the following concepts:
* String Processing
* Pointers
* File I/O
Assignment
Write a program that contains three functions used to read text data from a file into a buffer, write text data from a buffer into a file, and clean a string in a buffer for use with the Affine cipher given that the alphabet size is 26. A clean string for the Affine cipher contains only lower-case English letters (no spaces, no numbers, no other symbols). The input file can contain any message. The program should first make a function call to read the data file into a buffer. Another function call should be made to clean the buffer containing the files contents. Finally, you should make a function call to place the cleaned contents back into the text file. Minimally, the following functions should be implemented and should behave in the described manner:
int ReadStringFromFile(char* fileName, char* string) - Opens up a file called fileName with a relative file path. Read the raw contents of the file into the passed string. Return TRUE if the file was successfully opened and the contents were moved into string. Returns FALSE is an error occurs. In the event of an error, the function should display an informative message to the user indicating what went wrong.
int WriteStringToFile(char* filename, char* string) - Opens a new file called filename with a relative file path. Write the contents of string into the newly created file. If the function successfully creates and populates the new file, return a value of TRUE. If some sort of error occurs, return FALSE. In the event of an error the function should display an informative message to the user indicating what went wrong.
int CleanString(char* string) - Takes a dirty string in through the string argument. The function should clean the string (remove or convert all offensive characters), and rewrite the cleaned contents back into string. The function should return TRUE if the string was successfully cleaned. It should return FALSE if some sort of error occurred. In the event of an error, display an informative message to the user indicating what went wrong.
Use the following shell of code to begin your program. Take special note of all provided preprocessor directive statements containing entries for the filenames. Make sure to include a text file with some dirty data in the appropriate location on the file system to the compiler can see it.
After the variables have been created and initialized, write a printf() statement that shows the information in an intelligible sentence conveying that information. When you display your sentence, you must use the appropriate conversion characters within your printf() statement to make the variable contents show on the screen. You should end your single line display by moving the cursor to a new line.
// INCLUDES AND DEFINES
#include
#include
#ifndef __TRUE_FALSE__
#define __TRUE_FALSE__
#define TRUE 1
#define FALSE 0
#endif
#define BUFFER_SIZE 10000
#define INPUT_FILE "input.txt"
#define OUTPUT_FILE "output.txt"
#define ASCII_UPPER_LOWER_OFFSET 32
// PROTOTYPES
int ReadStringFromFile(char*, char*);
int WriteStringToFile(char*, char*);
int CleanString(char*);
// MAIN
int main() {
char buffer[BUFFER_SIZE] = "";
ReadStringFromFile(INPUT_FILE, buffer);
CleanString(buffer);
WriteStringToFile(OUTPUT_FILE, buffer);
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
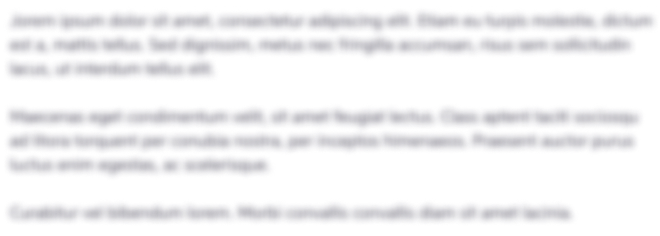
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started