Answered step by step
Verified Expert Solution
Question
1 Approved Answer
OVERVIEW In this assignment, you will implement a dictionary - like data structure called a hash table as a C abstract data type ( ADT
OVERVIEW In this assignment, you will implement a dictionarylike data structure called a hash table as a C abstract data type ADT After validating its correctness, you will implement a program that makes use of your hash table. GETTING STARTED For this assignment, you are asked to implement a hash table ADT in two modules: the ht module with the public interface and the htimpl module with private declarations and definitions. You are given a header file, hth which describes the public interface for the hash table abstract data type. You will implement those function signatures in a file called htc and declare any additional private datatypes and functions in another header, called htimpl.h Skeleton versions of these additional files are provided. You will first test your hash table ADT with a separate program, before using it to implement a postal code collator. The public interface must be complete, and its header file must not be changed. HASH TABLES IN ACTION: A POSTAL CODE COLLATOR You are asked to write a program, named pcode.c which uses your hash table implementation for a postal code collator. A skeleton file is provided. We have also provided several files containing the name of a city, town, or hamlet in Canada followed by a postal code in that location. Larger communities have of course more than one postal code. $ cat postalcodesin Quebec,GEC Forest Grove,VKM Gloucester,KBL Saintfelicien,GKR North York,MJZ Montreal,HYB Welland,LCT Montreal,HZP Laval,HAE Oshawa,LHB Kinburn,KAH Montreal,HGG Winnipeg,RLK Williamswood,BVC Calgary,TGM Unionville,LRY Leamington,NHW Markham,LRN Fort Erie,LAR Mississauga,LTE $ The path and name of the data file is passed as a command line argument to the program. The program reads the data from the file and inserts every postal code into the hash table, keyed by city. When a citypostal code pair is read, and the key city is already present in the table, the new postal code must be added to the existing value. For example, the file postalcodesin contains different postal codes for Montreal. When the program is finished processing each of the data lines, the value for the key Montreal must contain all postal codes. It is your design decision how you want to store multiple postal codes in a single hash table value. After the file has been processed and the hash table is created, the user can enter the name of a city on stdin The postal codes for that city are printed to standard output. If a city has more than one postal code, they should all be printed out, separated by commas, with a maximum of per line in the order that they appeared in the file. If the entered city does not exist, the program should print a message to the screen. The program should keep asking the user to enter a city until CtrlD is entered, and the program terminates. Example run the underlined text is the user input: $ pcode postalcodesin Enter a city name: Calgary Postal codes found: TGM Enter a city name: Montreal Postal codes found: HYB HZP HGG Enter a city name: Edmonton No postal codes found Enter a city name: $ Skeleton Files: hth: Picture attached htc: #include hth Import the public hashtable header. #include htimpl.h Also import the private header. struct hasht htimpl.h: #ifndef HTIMPLH #define HTIMPLH #include hth Import the public hashtable header. htimpl.c: #include htimpl.h Import the private header. htTest.c: #include hth Only import the public hashtable header. htTest.c is not allowed access to the htimpl.h private header int mainvoid return ; pcode.c: #include hth Only import the public hashtable header. pcode.c is not allowed access to the htimpl.h private header int mainint argc, char argv return ;
OVERVIEW
In this assignment, you will implement a dictionarylike data structure called a hash table as a C abstract data type ADT After validating its correctness, you will implement a program that makes use of your hash table.
GETTING STARTED
For this assignment, you are asked to implement a hash table ADT in two modules: the ht module with the public interface and the htimpl module with private declarations and definitions. You are given a header file, hth which describes the public interface for the hash table abstract data type. You will implement those function signatures in a file called htc and declare any additional private datatypes and functions in another header, called htimpl.h Skeleton versions of these additional files are provided. You will first test your hash table ADT with a separate program, before using it to implement a postal code collator. The public interface must be complete, and its header file must not be changed.
HASH TABLES IN ACTION: A POSTAL CODE COLLATOR
You are asked to write a program, named pcode.c which uses your hash table implementation for a postal code collator. A skeleton file is provided. We have also provided several files containing the name of a city, town, or hamlet in Canada followed by a postal code in that location. Larger communities have of course more than one postal code.
$ cat postalcodesin
Quebec,GEC
Forest Grove,VKM
Gloucester,KBL
Saintfelicien,GKR
North York,MJZ
Montreal,HYB
Welland,LCT
Montreal,HZP
Laval,HAE
Oshawa,LHB
Kinburn,KAH
Montreal,HGG
Winnipeg,RLK
Williamswood,BVC
Calgary,TGM
Unionville,LRY
Leamington,NHW
Markham,LRN
Fort Erie,LAR
Mississauga,LTE
$
The path and name of the data file is passed as a command line argument to the program. The program reads the data from the file and inserts every postal code into the hash table, keyed by city. When a citypostal code pair is read, and the key city is already present in the table, the new postal code must be added to the existing value. For example, the file postalcodesin contains different postal codes for Montreal. When the program is finished processing each of the data lines,
the value for the key Montreal must contain all postal codes. It is your design decision how you want to store multiple postal codes in a single hash table value.
After the file has been processed and the hash table is created, the user can enter the name of a city on stdin The postal codes for that city are printed to standard output. If a city has more than one postal code, they should all be printed out, separated by commas, with a maximum of per line in the order that they appeared in the file. If the entered city does not exist, the program should print a message to the screen. The program should keep asking the user to enter a city until CtrlD is entered, and the program terminates. Example run the underlined text is the user input:
$ pcode postalcodesin
Enter a city name: Calgary
Postal codes found:
TGM
Enter a city name: Montreal
Postal codes found:
HYB HZP HGG
Enter a city name: Edmonton
No postal codes found
Enter a city name:
$
Skeleton Files:
hth: Picture attached
htc:
#include hth Import the public hashtable header.
#include htimpl.h Also import the private header.
struct hasht
htimpl.h:
#ifndef HTIMPLH
#define HTIMPLH
#include hth Import the public hashtable header.
htimpl.c:
#include htimpl.h Import the private header.
htTest.c:
#include hth Only import the public hashtable header.
htTest.c is not allowed access to the htimpl.h private header
int mainvoid
return ;
pcode.c:
#include hth Only import the public hashtable header.
pcode.c is not allowed access to the htimpl.h private header
int mainint argc, char argv
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
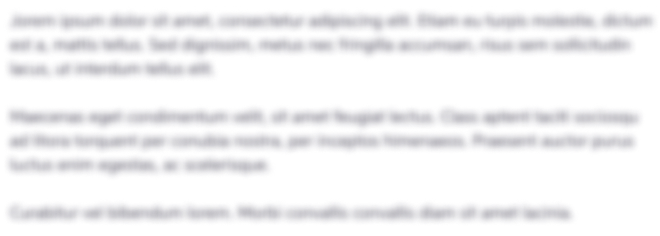
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started