Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Overview Search for #TODO tags to quickly find the functions you are required to implement. Descriptions and pseudocode for each function are provided in the
Overview
Search for #TODO tags to quickly find the functions you are required to implement. Descriptions and pseudocode for each function are provided in the notebook, along with links to external readings.
Complete the TravelingSalesmanProblem class by implementing the successors getsuccessor and getvalue methods
Complete the HillClimbingSolver class
Complete the LocalBeamSolver class
Complete the SimulatedAnnealingSolver class
Complete the LAHCSolver class
In :
import json
import math # contains sqrt exp, pow, etc.
import random
import time
from collections import deque
from helpers import
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
matplotlib inlineI. Hill Climbing
Next, complete the solve method for the HillClimbingSolver class below.
The hillclimbing search algorithm is the most basic local search technique. At each step the current node is replaced by the neighbor with the highest value. AIMA rd ed Chapter
Pseudocode for the hill climbing function from the AIMA textbook. Note that our Problem class is already a "node", so the MAKENODE line is not required.
In :
class HillClimbingSolver:
Parameters
epochs : int
The upper limit on the number of rounds to perform hill climbing; the
algorithm terminates and returns the best observed result when this
iteration limit is exceeded.
def initself epochs:
self.epochs epochs
def solveself problem:
Optimize the input problem by applying greedy hill climbing.
Parameters
problem : Problem
An initialized instance of an optimization problem. The Problem class
interface must implement a callable method "successors which returns
a iterable sequence ie a list or generator of the states in the
neighborhood of the current state, and a property "utility" which returns
a fitness score for the state. See the TravelingSalesmanProblem class
for more details.
Returns
Problem
The resulting approximate solution state of the optimization problem
Notes
DO NOT include the MAKENODE line from the AIMA pseudocode
# TODO: Implement this function!
for in :
neihbor maxproblemsuccessors
ifneihborsome problem.something:
raise NotImplementedError
Run the Hill Climbing Solver
In :
solver HillClimbingSolverepochs
starttime time.perfcounter
result solver.solvecapitalstsp
stoptime time.perfcounter
printsolutiontime: :f milliseconds".formatstoptime starttime
printInitial path length: :fformatcapitalstsputility
printFinal path length: :fformatresult.utility
printresultpath
showpathresultcoords, startingcity
Step by Step Solution
There are 3 Steps involved in it
Step: 1
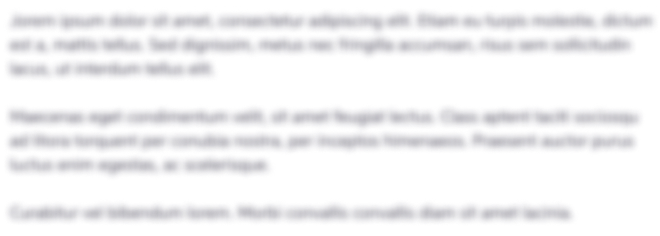
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started