Overview The objective of this assignment is to read in a list of (playing) cards from standard input and output a frequency histogram of the
Overview
The objective of this assignment is to read in a list of (playing) cards from standard input and output a frequency histogram of the playing cards read in.
Task
Recall some of the Week 03 Lecture Files, e.g., p7.cxx, r1.cxx, r2.cxx, and r3.cxx where:
- The p7 program was capable of reading in and writing out (playing) cards, i.e., struct card.
- r1 to r3 was capable constructing a frequency histogram.
This assignment's task is to read in card structs from standard input and place such in to a frequency histogram. Input is to occur until EOF (end-of-file) or an input error occurs. After reading in all input, for each entry in the frequency histogram's map, output the following (always on its own line):
occurs time(s)
where
Tips
- Do look at p7, r1, r2, and r3 to write the code for this assignment. Copy the relevant bits you need in to your code.
- NOTE: if you take p7.cxx and delete all lines starting at "struct cards" (i.e., line 85) to the end, then you will only need to write main() to do this assignment. :-)
- To output the histogram, use iterators to iterate through the map (or a range-for loop).
- NOTE: Use prefix ++ instead of postfix ++. Why? Prefix ++ and -- are more efficient --only use the postfix forms when they are absolutely needed.
- Each element in the map
is a std::pair and one can access the Key via .first or ->first and Value via .second or ->second. - Most of the code for this assignment is from the p7.cxx --but with the code handling cards removed and the code inside main() removed. Essentially this assignment is writing code in main() to compute a frequency histogram of cards since the rest of the code has been provided.
Sample Program Input
The program input will be a series of zero or more cards. (Read the input until EOF or an error.) For example, sample input might be placed in a file called input.dat, e.g.,
P7:
#include
using namespace std;
//------
struct card { enum class suit { club, spade, diamond, heart }; enum { ace=1, jack=10, queen=11, king=12 }; using number = int;
number num_; suit suit_; };
bool operator ==(card const& a, card const& b) { return a.num_ == b.num_ && a.suit_ == b.suit_; }
bool operator
istream& operator >>(istream& is, card& c) { // number followed by the suit (CSHD)... is >> c.num_;
char ch; if (is >> ch) { switch (ch) { case 'C': c.suit_ = card::suit::club; break; case 'S': c.suit_ = card::suit::spade; break; case 'H': c.suit_ = card::suit::heart; break; case 'D': c.suit_ = card::suit::diamond; break; default: is.setstate(ios::failbit); break; } } else is.setstate(ios::badbit); return is; }
ostream& operator
switch (c.suit_) { // card::suit allows us to access suit // suit::club allows us to access club // thus, card::suit::club lets us access club case card::suit::club: os
case card::suit::spade: os
case card::suit::diamond: os
case card::suit::heart: os
return os; }
//------
int main(){
}
R1:
#include
using namespace std;
int main() { // map of (number, num of times number occurs)... map
int i; while (cin >> i) ++freqhist[i];
cout
R2:
#include
using namespace std;
int main() { // map of (number, num of times number occurs)... map
string i; while (cin >> i) ++freqhist[i];
cout
R3:
#include
using namespace std;
int main() { // map of (number, num of times number occurs)... map
string i; while (cin >> i) { auto[pos,is_added] = freqhist.insert({i,1}); if (!is_added) ++pos->second; }
cout
**YOU ONLY HAVE TO CODE THE INT MAIN() IN P7 TO COMPLETE THIS**
1. 2. 3. $ cat input. dat 10D3S4H9C10DBH2D12510D6H2D4H Sample Program Run The program output for the above input.dat file is: 1. 2. 3. 4. 5. 6. $ g++-10.2.0 -Wall -Wextra -Werror a3.cxx $ ./a.outStep by Step Solution
There are 3 Steps involved in it
Step: 1
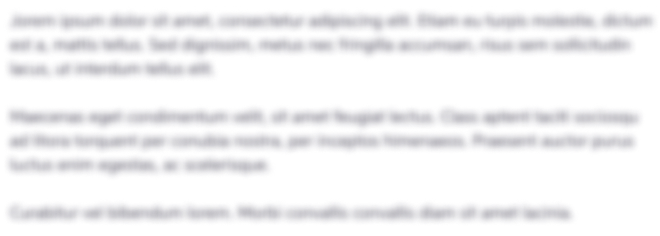
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started