Answered step by step
Verified Expert Solution
Question
1 Approved Answer
P 2 0 . 2 Program 1 : List Methods Objectives Create a user - defined Java class that performs various operations with 1 -
P
Program : List Methods
Objectives
Create a userdefined Java class that performs various operations with dimensional arrays.
Use a static main method in a separate file to test the class methods.
Develop programs using an IDE.
Upload both the userdefined class and the driver class for grading.
Background Reading
ZyBooks: Arrays, Methods
Header comment section
Recall that each file you code should contain the following header information.
Program #
Line Description of classprogram
CSSection#
Date
@author Student Name
and the ID string method
The sole purpose of these methods is just as it says: to identify you as the author of the code. No matter what else is required in your program, the noarg" version of this method must also be included in all programs you write this semester.
getInfo should return a string in the form of the following where your name is substituted for "Student Name".
Program # Student Name
Instructions
You are to write an application application is used here to mean "program" called ListMethods.java that does some useful things with arrays. In addition to the getInfo method, your program should have the following methods.
public int findMin that accepts a onedimensional array as a parameter and returns the smallest value in the array. If the array is empty size it returns Integer.MINVALUE.
public int findMinIndex that accepts a onedimensional array as a parameter and returns the index of the smallest value in the array. If the array is empty size it returns
public void reverse that accepts a onedimensional array as a parameter and reverses order of the array values. Hint: there are two approaches to this: swap the array valuesbut stop half way or you'll swap them back to their original position, or create a new array and copy the values into it in the reverse order from the original array, then copy the new reversed array back into the original array.
public boolean shiftUp that accepts a onedimensional array as a parameter and moves all values in the array from their current index to current index minus Note that the value in position is overwritten. The highest index is not overwritten ie the value at arrayarraylength will be duplicated in arrayarraylength The method should return true unless the array size is zero.
public boolean shiftDown that accepts a onedimensional array as a parameter and moves all values in the array from their current index to current index plus Note that the value in arrayarraylength is overwritten. The lowest index is not overwritten ie the value at array will be duplicated in array The method should return true unless the array size is zero.
Making a main method to test your stuff
The array methods do not have the word "static" in the method header. The way to access nonstatic methods from main a static context is to create an instance of our ListMethods class and call the methods using the object reference. Like this:
public static void mainString args
ListMethods app new ListMethods;
int testData ;
int min app.findMintestData;
System.out.printlnSmallest value is min;
Add more calls to the other methods here, possibly using different arrays.
Program : List Methods
Objectives
Create a userdefined Java class that performs various operations with dimensional arrays.
Use a static main method in a separate file to test the class methods.
Develop programs using an IDE.
Upload both the userdefined class and the driver class for grading.
Background Reading
ZyBooks: Arrays, Methods
Header comment section
Recall that each file you code should contain the following header information.
Program #
Line Description of classprogram
CSSection#
Date
@author Student Name
and the ID string method
The sole purpose of these methods is just as it says: to identify you as the author of the code. No matter what else is required in your program, the noarg" version of this method must also be included in all programs you write this semester.
getInfo should return a string in the form of the following where your name is substituted for "Student Name".
Program # Student Name
Instructions
You are to write an application application is used here to mean "program" called ListMethods.java that does some useful things with arrays. In addition to the getInfo method, your program should have the following methods.
public int findMin that accepts a onedimensional array as a parameter and returns the smallest value in the array. If the array is empty size it returns Integer.MINVALUE.
public int findMinIndex that accepts a onedimensional array as a parameter and returns the index of the smallest value in the array. If the array is empty size it returns
public void reverse that accepts a onedimensional array as a parameter and reverses order of the array values. Hint: there are two approaches to this: swap the array valuesbut stop half way or you'll swap them back to their original position, or create a new array and copy the values into it in the reverse order from the original array, then copy the new reversed array back into the original array.
public boolean shiftUp that accepts a onedimensional array as a parameter and moves all values in the array from their current index to current index minus Note that the value in position is overwritten. The highest index is not overwritten ie the value at arrayarraylength will be duplicated in arrayarraylength The method should return true unless the array size is zero.
public boolean shiftDown that accepts a onedimensional array as a parameter and moves all values in the array from their current index to current index plus Note that the value in arrayarraylength is overwritten. The lowest index is not overwritten ie the value at array will be duplicated in array The method should return true unless the array size is zero.
Making a main method to test your stuff
The array methods do not have the word "static" in the method header. The way to access nonstatic methods from main a static context is to create an instance of our ListMethods class and call the methods using the object reference. Like this:
public static void mainString args
ListMethods app new ListMethods;
int testData ;
int min app.findMintestData;
System.out.printlnSmallest value is min;
Add more calls to the other methods here, possibly using different arrays.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
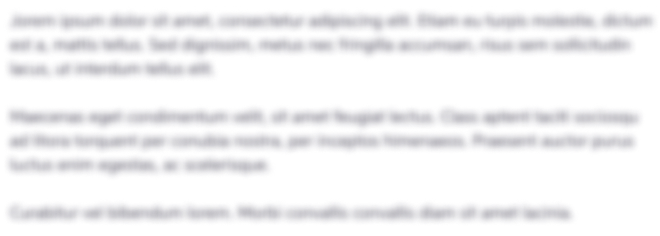
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started