Question
package algs24; import stdlib.StdIn; import stdlib.StdOut; /** * The PtrHeap class is the priorityQ class from Question 2.4.24. * It represents a priority queue of
package algs24;
import stdlib.StdIn;
import stdlib.StdOut;
/**
* The PtrHeap class is the priorityQ class from Question 2.4.24.
* It represents a priority queue of generic keys.
*
* It supports the usual insert and delete-the-maximum
* operations, along with methods for peeking at the maximum key,
* testing if the priority queue is empty, and iterating through
* the keys.
* For additional documentation, see Section 2.4 of
* Algorithms, 4th Edition by Robert Sedgewick and Kevin Wayne.
*/
public class PtrHeap
@SuppressWarnings("unchecked")
/** Create an empty priority queue */
public PtrHeap() {
}
/** Is the priority queue empty? */
public boolean isEmpty() { return true; }
/** Is the priority queue full? */
public boolean isFull() { return true; }
/** Return the number of items on the priority queue. */
public int size() { return 0; }
/**
* Return the largest key on the priority queue.
* Throw an exception if the priority queue is empty.
*/
public K max() {return null;
}
/** Add a new key to the priority queue. */
public void insert(K x) { return;
}
/**
* Delete and return the largest key on the priority queue.
* Throw an exception if the priority queue is empty.
*/
public K delMax() {return null;
}
private void showHeap() {
// a method to print out the heap
// useful for debugging
}
public static void main(String[] args) {
PtrHeap
StdIn.fromString("10 20 30 40 50 - - - 05 25 35 - - - 70 80 05 - - - - ");
while (!StdIn.isEmpty()) {
StdOut.print ("pq: "); pq.showHeap();
String item = StdIn.readString();
if (item.equals("-")) StdOut.println("max: " + pq.delMax());
else pq.insert(item);
}
StdOut.println("(" + pq.size() + " left on pq)");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
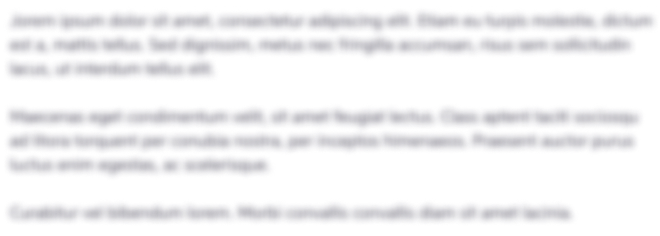
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started