Question
package college; import java.util.Scanner; public class CollegeDriver { private static Player player = new Player(Chris); private static Scanner sc = new Scanner(System.in); public static void
package college;
import java.util.Scanner;
public class CollegeDriver {
private static Player player = new Player("Chris"); private static Scanner sc = new Scanner(System.in);
public static void main(String[] args) { System.out.println("Type T to test, or any other character to play."); String answer = sc.nextLine(); try { if (answer.toUpperCase().equals("T")) test(); else play(); } catch (InterruptedException e) { e.printStackTrace(); } }
private static void test() throws InterruptedException { while (player.getLocation()
private static void play() throws InterruptedException { while (player.getLocation()
import java.util.HashMap; import java.util.function.Consumer;
public class Game { public static final int NUMBER_OF_CLUBS = 0; public static final int NUMBER_OF_FRIENDS = 1; public static final int MONEY = 225; public static final int HAPPINESS = 20; public static final double GPA = 3.0; public static final int LAST_SQUARE = 26; public static HashMap
public static int roll() { return (int)(Math.random()*6+1); }
}
import java.text.DecimalFormat;
public class Player {
String name; int numberOfClubs; int numberOfFriends; int money; int happiness; double gpa; int location; private static DecimalFormat df = new DecimalFormat("0.00"); public Player(String name) { this.name = name; this.numberOfClubs = Game.NUMBER_OF_CLUBS; this.numberOfFriends = Game.NUMBER_OF_FRIENDS; this.money = Game.MONEY; this.happiness = Game.HAPPINESS; this.gpa = Game.GPA; this.location = 0; } public void move(int squares) { this.location += squares; if (this.location >= Game.LAST_SQUARE) this.location = Game.LAST_SQUARE; // Use System.err output stream to get red text! No error here. System.err.println(" " + Game.squareText.get(this.location)); if (this.location == Game.LAST_SQUARE) getStats(); } public void moveTo(int square) { this.location = square; if (this.location >= Game.LAST_SQUARE) this.location = Game.LAST_SQUARE; // Use System.err output stream to get red text! No error here. System.err.println(" " + Game.squareText.get(this.location)); if (this.location == Game.LAST_SQUARE) getStats(); }
private void getStats() { Double delta; System.out.println(" FINAL RESULTS"); delta = (double) (this.numberOfFriends - Game.NUMBER_OF_FRIENDS); outputStat("Number of Friends", delta); delta = (double) (this.money - Game.MONEY ); outputStat("Money", delta); delta = (double) (this.happiness - Game.HAPPINESS ); outputStat("Happiness", delta); delta = (double) (this.gpa - Game.GPA); outputStat("GPA", delta); } private void outputStat(String property, double delta) { if (delta > 0) { System.out.println("\t" + property + " improved!"); } else if (delta
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getNumberOfClubs() { return numberOfClubs; }
public void setNumberOfClubs(int numberOfClubs) { this.numberOfClubs = numberOfClubs; }
public int getNumberOfFriends() { return numberOfFriends; }
public void setNumberOfFriends(int numberOfFriends) { this.numberOfFriends = numberOfFriends; if (this.numberOfFriends
public int getMoney() { return money; }
public void setMoney(int money) { this.money = money; }
public int getHappiness() { return happiness; }
public void setHappiness(int happiness) { this.happiness = happiness; }
public double getGpa() { return gpa; }
public void setGpa(double gpa) { this.gpa = gpa; if (this.gpa 4.0) this.gpa = 4.0; }
public int getLocation() { return location; }
public void setLocation(int location) { this.location = location; }
@Override public String toString() { return name + ": [" + "numberOfClubs=" + numberOfClubs + ", numberOfFriends=" + numberOfFriends + ", money=$" + money + ", happiness=" + happiness + ", gpa=" + df.format(gpa) + ", location=" + location + "]"; } }
The Game of College We will be building a board game, where a player rolls a dice and progresses through the board from Orientation to Graduation. Orientation Fall asleep in class. Lose 10% of GPA Roommate comforts you. Happiness +5 Roommate neads a loan. Lose $200 Skip class to travel to New York. Happiness +10. Lose 0.2 GPA. Pull an all nighter. Happiness - 3. Increase GPA by 0.1 Move ahead two spaces Lose a friend Roommate pays you back - with interest! Gain $220 Get caught buying paper from web. Lose $100 and 0.5 GPA Throw major party. Gain 2 friends. Lose $100. Kick ass on midterms. Improve GPA by 20%. Help classmate study Gain a friend Join a Club Join a Club Turn research entrepreneurial Gain 0.8 GPA and $2000 Fail DSA Go back 3 spaces; Lose 0.5 GPA Dean discovers your illegal party. Happiness -12 Win eSports tournament. Happiness +10; Gain $1000 Join Honors Club Gain 1.0 GPA Gain 2 friends Senioritis! Lose 20% GPA and $400 Study hard for Calculus III. Happiness -7; Gain 0.3 GPA Borrow from friend; forget to repay. Gain $150; Lose a friend Robbed! Lose $300 Graduation Get graduation gift from Aunt June Gain $750; Happiness +15 Playing the game might look like this: Roommate comforts you. Chris: [numberOfClubs-e, numberOfFriends-1, money-$225, happiness-25, gpa-3.00, location-2] Roommate needs a loan. Chris: [numberOfClubs-o, numberOfFriends-1, money-$25, happiness-25, gpa-3.00, location=3] Move ahead two spaces. Help classmate study. Chris: (numberOfClubs=e, numberOfFriends=2, money-$25, happiness=25, gpa=3.00, location=8] Lose a friend. Chris: [numberOfClubs-o, number of Friends-1, money-$25, happiness-25, gpa-3.00, location-13] Fail DSA Chris: [numberOfClubs-e, numberOfFriends-1, money-$25, happiness-25, gpa-2.50, location=16] Lose a friend. Chris: [numberOfClubs=e, numberOfFriends=0, money-$25, happiness-25, gpa-2.50, location=13] Turn research ent eneurial. Chris: (numberOfClubs=, numberOfFriendsze, money-$2025, happiness=25, gpa=3.30, location=15] Dean discovers your illegal party. Chris: (numberOfClubs-, numberOfFriends=0, money-$2025, happiness=13, gpa-3.30, location=17] Senioritis! Chris: [numberOfClubs-e, numberOfFriends-, money-$1625, happiness-13, gpa-2.64, location-23] Get graduation gift from Aunt June. Chris: [numberOfClubs-e, numberOfFriends-, money-$2375, happiness-28, gpa-2.64, location-25] GRADUATED! FINAL RESULTS Money improved! Happiness improved! Number of Friends worsened! GPA worsened! The Game class has a HashMap of all the text for each square indexed by square number. You will need to complete the game. 1. Create, in the Game class a similar HashMap, but one that contains Consumers to represent the action of each square. 2. Finish the run method in the Driver so that the player actions stored in the HashMap above are executed. The Game of College We will be building a board game, where a player rolls a dice and progresses through the board from Orientation to Graduation. Orientation Fall asleep in class. Lose 10% of GPA Roommate comforts you. Happiness +5 Roommate neads a loan. Lose $200 Skip class to travel to New York. Happiness +10. Lose 0.2 GPA. Pull an all nighter. Happiness - 3. Increase GPA by 0.1 Move ahead two spaces Lose a friend Roommate pays you back - with interest! Gain $220 Get caught buying paper from web. Lose $100 and 0.5 GPA Throw major party. Gain 2 friends. Lose $100. Kick ass on midterms. Improve GPA by 20%. Help classmate study Gain a friend Join a Club Join a Club Turn research entrepreneurial Gain 0.8 GPA and $2000 Fail DSA Go back 3 spaces; Lose 0.5 GPA Dean discovers your illegal party. Happiness -12 Win eSports tournament. Happiness +10; Gain $1000 Join Honors Club Gain 1.0 GPA Gain 2 friends Senioritis! Lose 20% GPA and $400 Study hard for Calculus III. Happiness -7; Gain 0.3 GPA Borrow from friend; forget to repay. Gain $150; Lose a friend Robbed! Lose $300 Graduation Get graduation gift from Aunt June Gain $750; Happiness +15 Playing the game might look like this: Roommate comforts you. Chris: [numberOfClubs-e, numberOfFriends-1, money-$225, happiness-25, gpa-3.00, location-2] Roommate needs a loan. Chris: [numberOfClubs-o, numberOfFriends-1, money-$25, happiness-25, gpa-3.00, location=3] Move ahead two spaces. Help classmate study. Chris: (numberOfClubs=e, numberOfFriends=2, money-$25, happiness=25, gpa=3.00, location=8] Lose a friend. Chris: [numberOfClubs-o, number of Friends-1, money-$25, happiness-25, gpa-3.00, location-13] Fail DSA Chris: [numberOfClubs-e, numberOfFriends-1, money-$25, happiness-25, gpa-2.50, location=16] Lose a friend. Chris: [numberOfClubs=e, numberOfFriends=0, money-$25, happiness-25, gpa-2.50, location=13] Turn research ent eneurial. Chris: (numberOfClubs=, numberOfFriendsze, money-$2025, happiness=25, gpa=3.30, location=15] Dean discovers your illegal party. Chris: (numberOfClubs-, numberOfFriends=0, money-$2025, happiness=13, gpa-3.30, location=17] Senioritis! Chris: [numberOfClubs-e, numberOfFriends-, money-$1625, happiness-13, gpa-2.64, location-23] Get graduation gift from Aunt June. Chris: [numberOfClubs-e, numberOfFriends-, money-$2375, happiness-28, gpa-2.64, location-25] GRADUATED! FINAL RESULTS Money improved! Happiness improved! Number of Friends worsened! GPA worsened! The Game class has a HashMap of all the text for each square indexed by square number. You will need to complete the game. 1. Create, in the Game class a similar HashMap, but one that contains Consumers to represent the action of each square. 2. Finish the run method in the Driver so that the player actions stored in the HashMap above are executed
Step by Step Solution
There are 3 Steps involved in it
Step: 1
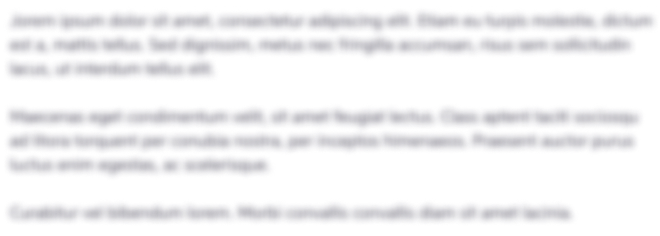
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started