Answered step by step
Verified Expert Solution
Question
1 Approved Answer
package console_apps; import java.util.Scanner; import model.Utilities; public class GetIntermediateStatsApp { public static void main(String[] args) { Scanner input = new Scanner(System.in); /* Prompt the user
package console_apps;
import java.util.Scanner;
import model.Utilities;
public class GetIntermediateStatsApp {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
/* Prompt the user for input bounds. */
System.out.println("Enter the first integer term of an arithmetic sequence:");
int ft = input.nextInt();
System.out.println("Enter the common difference of the sequence:");
int d = input.nextInt();
System.out.println("Enter the size of the sequence:");
int n = input.nextInt();
/* Invoke the utility method for calculation. */
String result = Utilities.getIntermediateStats(ft, d, n);
/* Output the result. */
System.out.println(result);
input.close();
}
}
/*
* Input parameters:
* - `ft`, `d`, `n` are the first term, common difference, and size of an arithmetic sequence.
*
* Use of arrays or any Java library class (e.g., ArrayList) is strictly forbidden.
* Violation of this will result in a 50% penalty on your marks.
* Try to solve this problem using loops only.
*
* Refer to you lab instructions for what the method should return.
*/
public static String getIntermediateStats(int ft, int d, int n) {
String result = "";
/* Your implementation of this method starts here.
* Recall from Week 1's tutorial videos:
* 1. No System.out.println statements should appear here.
* Instead, an explicit, final `return` statement is placed for you.
* 2. No Scanner operations should appear here (e.g., input.nextInt()).
* Instead, refer to the input parameters of this method.
*/
/* Your implementation ends here. */
return result;
}
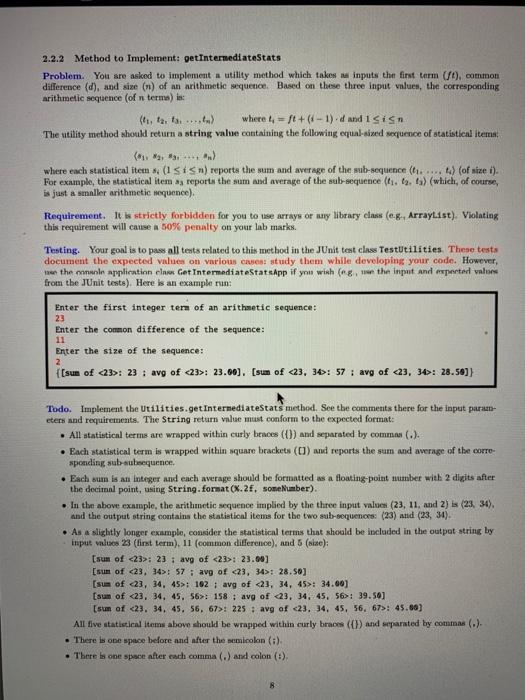
/*
* Tests related to getIntermediateStats
*/
@Test
public void test_getIntermediateStats_01() {
String result = Utilities.getIntermediateStats(7, 3, 0);
assertEquals("{}", result);
}
@Test
public void test_getIntermediateStats_02() {
String result = Utilities.getIntermediateStats(7, 3, 1);
String expected = "{[sum of : 7 ; avg of : 7.00]}";
assertEquals(expected, result);
}
@Test
public void test_getIntermediateStats_03() {
String result = Utilities.getIntermediateStats(7, 3, 2);
String expected = "{";
expected += "[sum of : 7 ; avg of : 7.00]" + ", ";
expected += "[sum of : 17 ; avg of : 8.50]";
expected += "}";
assertEquals(expected , result);
}
@Test
public void test_getIntermediateStats_04() {
String result = Utilities.getIntermediateStats(7, 3, 3);
String expected = "{";
expected += "[sum of : 7 ; avg of : 7.00]" + ", ";
expected += "[sum of : 17 ; avg of : 8.50]" + ", ";;
expected += "[sum of : 30 ; avg of : 10.00]";
expected += "}";
assertEquals(expected, result);
}
@Test
public void test_getIntermediateStats_05() {
String result = Utilities.getIntermediateStats(7, 3, 4);
String expected = "{";
expected += "[sum of : 7 ; avg of : 7.00]" + ", ";
expected += "[sum of : 17 ; avg of : 8.50]" + ", ";
expected += "[sum of : 30 ; avg of : 10.00]" + ", ";
expected += "[sum of : 46 ; avg of : 11.50]";
expected += "}";
assertEquals(expected, result);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
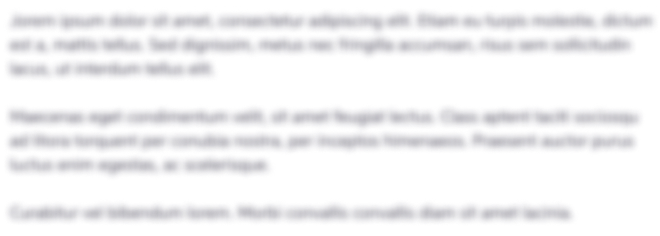
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started