Question
package hw2; /** * * A class that mimics how Java's String class behaves with a few minor changes. * See the documentation for each
package hw2;
/**
*
* A class that mimics how Java's String class behaves with a few minor changes.
* See the documentation for each of the methods.
*
*/
public class String {
private char[] data;
@Override
public boolean equals(Object obj) {
String other = (String) obj;
return this.equals(other);
}
/************** DO NOT MODIFY ANYTHING ABOVE THIS LINE **************/
/**
* Allocates a new {@code String} so that it represents the sequence of
* characters currently contained in the character array argument. The
* contents of the character array are copied; subsequent modification of
* the character array does not affect the newly created string.
*
* @param value
* The initial value of the string
*/
public String(char[] value) {
//TODO
throw new RuntimeException("Not Implelmented");
}
/**
* Returns the {@code char} value at the
* specified index. An index ranges from {@code 0} to
* {@code length() - 1}. The first {@code char} value of the sequence
* is at index {@code 0}, the next at index {@code 1},
* and so on, as for array indexing.
*
* @param index the index of the {@code char} value.
* @return the {@code char} value at the specified index of this string.
* The first {@code char} value is at index {@code 0}.
* @exception IndexOutOfBoundsException if the {@code index}
* argument is negative or not less than the length of this
* string.
*/
public char charAt(int index) {
//TODO
throw new RuntimeException("Not Implelmented");
}
/**
* Returns the length of this string.
* The length is equal to the number of characters in the string.
*
* @return the length of the sequence of characters represented by this
* object.
*/
public int length() {
//TODO
throw new RuntimeException("Not Implelmented");
}
/**
* Returns the index within this string of the first occurrence of
* the specified character. If a character with value
* {@code ch} occurs in the character sequence represented by
* this {@code String} object, then the index of the first such
* occurrence is returned. This is the smallest value k such that:
*
* this.charAt(k) == ch
*
* is true. If no such character occurs in this
* string, then {@code -1} is returned.
*
* @param ch a character.
* @return the index of the first occurrence of the character in the
* character sequence represented by this object, or
* {@code -1} if the character does not occur.
*/
public int indexOf(char ch) {
//TODO
throw new RuntimeException("Not Implelmented");
}
/**
* Compares this string to the specified string. The result is {@code
* true} if and only if the argument is not {@code null} and
* represents the same sequence of characters as this string. This
* equality check is case sensitive.
*
* @param other
* The {@code String} to compare this {@code String} against
*
* @return {@code true} if the given {@code String} is
* equivalent to this {@code String}, {@code false} otherwise
*/
public boolean equals(String other) {
//TODO
throw new RuntimeException("Not Implelmented");
}
/**
* Compares two strings lexicographically.
* The comparison is based on the Unicode value of each character in
* the strings. The character sequence represented by this
* String
object is compared lexicographically to the
* character sequence represented by the argument string. The result is
* a negative integer if this String
object
* lexicographically precedes the argument string. The result is a
* positive integer if this String
object lexicographically
* follows the argument string. The result is zero if the strings
* are equal; compareTo
returns 0
exactly when
* the {@link #equals(String)} method would return true
.
*
* This is the definition of lexicographic ordering. If two strings are
* different, then either they have different characters at some index
* that is a valid index for both strings, or their lengths are different,
* or both. If they have different characters at one or more index
* positions, let k be the smallest such index; then the string
* whose character at position k has the smaller value, as
* determined by using the < operator, lexicographically precedes the
* other string.
* If there is no index position at which they differ, then the shorter
* string lexicographically precedes the longer string.
*
* @param anotherString the String
to be compared.
* @return the value 0
if the argument string is equal to
* this string; a value less than 0
if this string
* is lexicographically less than the string argument; and a
* value greater than 0
if this string is
* lexicographically greater than the string argument.
*/
public int compareTo(String anotherString) {
//TODO
throw new RuntimeException("Not Implelmented");
}
/**
* Returns a string resulting from replacing all occurrences of
* {@code oldChar} in this string with {@code newChar}.
*
* If the character {@code oldChar} does not occur in the
* character sequence represented by this {@code String} object,
* then a reference to this {@code String} object is returned.
* Otherwise, a newly created {@code String} object is returned that
* represents a character sequence identical to the character sequence
* represented by this {@code String} object, except that every
* occurrence of {@code oldChar} is replaced by an occurrence
* of {@code newChar}.
*
* Examples:
*
* "mesquite in your cellar".replace('e', 'o')
* returns "mosquito in your collar"
* "the war of baronets".replace('r', 'y')
* returns "the way of bayonets"
* "sparring with a purple porpoise".replace('p', 't')
* returns "starring with a turtle tortoise"
* "JonL".replace('q', 'x') returns "JonL" (no change)
*
*
* @param oldChar the old character.
* @param newChar the new character.
* @return a string derived from this string by replacing every
* occurrence of {@code oldChar} with {@code newChar}.
*/
public String replace(char oldChar, char newChar) {
//TODO
throw new RuntimeException("Not Implelmented");
}
/**
* Returns a string that is a substring of this string. The
* substring begins at the specified {@code beginIndex} and
* extends to the character at index {@code endIndex - 1}.
* Thus the length of the substring is {@code endIndex-beginIndex}.
*
* Examples:
*
* "hamburger".substring(4, 8) returns "urge"
* "smiles".substring(1, 5) returns "mile"
*
*
* @param beginIndex the beginning index, inclusive.
* @param endIndex the ending index, exclusive.
* @return the specified substring.
* @exception IndexOutOfBoundsException if the
* {@code beginIndex} is negative, or
* {@code endIndex} is larger than the length of
* this {@code String} object.
*/
public String substring(int beginIndex, int endIndex) {
//TODO
throw new RuntimeException("Not Implelmented");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
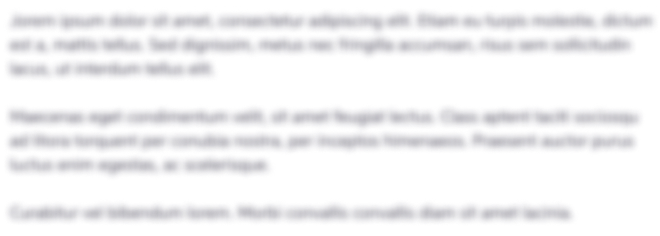
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started