Question
package javafxapplication2; import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.control.PasswordField; import javafx.scene.control.TextField; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import
package javafxapplication2; import javafx.application.Application; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.control.PasswordField; import javafx.scene.control.TextField; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.layout.GridPane; import javafx.scene.layout.HBox; import javafx.stage.Stage; import javafx.scene.text.Text; public class JavaFXApplication2 extends Application { public static void main(String[] args) { launch(args); } private ImageView imageview; @Override public void start(Stage primaryStage) { GridPane grid = new GridPane(); grid.setAlignment(Pos.CENTER); grid.setHgap(20); grid.setVgap(20); imageview=new ImageView(); imageview.setFitHeight(50); imageview.setFitWidth(100); Image img=new Image("file:src/images/icon1.png"); imageview.setImage(img); grid.add(imageview, 1, 1); Label userNameLbl = new Label("User Name"); grid.add(userNameLbl, 0, 2); TextField userNameTextField = new TextField(); grid.add(userNameTextField, 1, 2); Label passLbl = new Label("Password"); grid.add(passLbl, 0, 3); PasswordField password = new PasswordField(); grid.add(password, 1, 3); Button btnLogin = new Button("LogIn Button"); HBox loginBox = new HBox(10); loginBox.getChildren().add(btnLogin); grid.add(loginBox, 1, 5); final Text messageTxt = new Text(); grid.add(messageTxt, 1, 6); btnLogin.setOnAction(new EventHandler() { @Override public void handle(ActionEvent e) { messageTxt.setText("Logged In Successfully!!!"); } }); Scene scene = new Scene(grid, 500, 300); primaryStage.setScene(scene); primaryStage.show(); } }
Must design and implement the EventHandlers corresponding to their own GUI interface design.
1. Please test your handlers to call/invoke your GUI implementations. Also please enhance your implementation so that your handlers can run multiple processing steps instead of a simple String return.
2. One of the handlers should be associated with a button to retrieve/save customer info. When the button is clicked multiple times, multiple users/customers' information can be saved. And they can be retrieved later accordingly. 2a. (optional) You may implement a mouse/key handler as the 2nd handler.
3. You can implement the 2nd handler to response to other GUI components (if you choose NOT to implement the optional requirement #2.)
4. Try a variety of handler styles/structure. One handler must use the shorthand lamda notation format. The other(s) should use a different format of your choice (e.g., in a full handler class, as an anonymous inner class, and so on).
5. Your codes will be judged on functionality and variety of implementations as well as the integration with your previous GUI in the above code. If you implement more than the required 2 handlers, you choose the best TWO and comment out the rest of handlers. Please add detail comments/documentation in your codes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
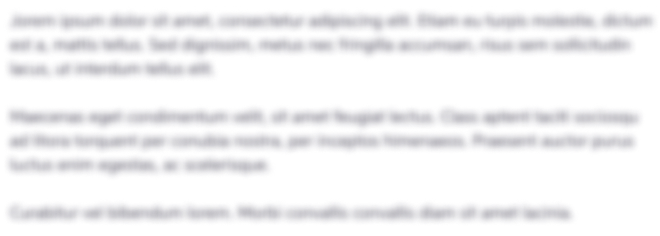
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started