Answered step by step
Verified Expert Solution
Question
1 Approved Answer
package Lab 0 4 ; import javax.swing.JFrame; import java.awt.BorderLayout; import java.awt.Color; import java.awt.Font; import java.awt.GridLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel;
package Lab;
import javax.swing.JFrame;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Font;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class TicTacToeFrame extends JFrame implements ActionListener
Define the game components
private JLabel lblStatus; The label to show the game status
private JPanel gamePanel; The panel that displays the button grid
private JButton btnGrid; A D array of buttons on the grid
private boolean xTurn; Stores the player turntrue X false
private boolean bFinished; Stores whether the game is finished or not
public static void mainString args
TicTacToeFrame frame new TicTacToeFrame;
frame.setVisibletrue;
Constructor method
public TicTacToeFrame
Add code for this constructor method
this.setDefaultCloseOperationEXITONCLOSE;
this.setSize; Set form size width and height
this.setLocationRelativeTonull; Make the frame appearing in the center of the screen
this.setTitleTic Tac Toe";
this.setLayoutnew BorderLayout;
xTurn true; Player X starts first
bFinished false; The game is not over at first
lblStatus new JLabelPlayer X turn";
lblStatus.setFontnew FontArial Font.BOLD, ;
this.addlblStatus BorderLayout.NORTH;
gamePanel new JPanelnew GridLayout; Game panel is a grid size x
this.addgamePanel BorderLayout.CENTER;
btnGrid new JButton; Initialize the button grid
forint i ; i ; i
forint j ; j ; j
btnGridij new JButton;
btnGridijsetFontnew FontArial Font.BOLD, ;
btnGridijsetBackgroundnew Color;
btnGridijsetOpaquetrue;
btnGridijaddActionListenerthis; register this as the event handler
gamePanel.addbtnGridij;
This method handles the button click event
@Override
public void actionPerformedActionEvent e
ifbFinished
return;
forint i ; i ; i
forint j ; j ; j
ifegetSource btnGridijEvent source is button at i j
ifbtnGridijgetTextisEmptyButton i j is not clicked before
btnGridijsetForegroundxTurn Color.red : Color.yellow;
btnGridijsetTextxTurn X : O;
xTurn xTurn; Invert xTurn to change the player turn
lblStatus.setTextStringformatPlayer s turn", xTurn X : O;
checkWin; check if the game is finished or not
This method checks if the game is finished or not
public void checkWin
Check rows
forint i ; i ; i
ifbtnGridigetTextisEmptyThe player has put symbol here already
&& btnGridigetTextequalsbtnGridigetText
&& btnGridigetTextequalsbtnGridigetText
lblStatus.setTextStringformatPlayer s wins", btnGridigetText;
bFinished true;
Check columns
forint i ; i ; i
ifbtnGridigetTextisEmptyThe player has put symbol here already
&& btnGridigetTextequalsbtnGridigetText
&& btnGridigetTextequalsbtnGridigetText
lblStatus.setTextStringformatPlayer s wins", btnGridigetText;
bFinished true;
check diagonal
forint i ; i ; i
ifbtnGridgetTextisEmptyThe player has put symbol here already
&& btnGridgetTextequalsbtnGridgetText
&& btnGridgetTextequalsbtnGridgetText
lblStatus.setTextStringformatPlayer s wins", btnGridgetText;
bFinished true;
check diagonal
forint i ; i ; i
ifbtnGridgetTextisEmptyThe player has put symbol here already
&& btnGridgetTextequalsbtnGridgetText
&& btnGridgetTextequalsbtnGridgetText
lblStatus.setTextStringformatPlayer s wins", btnGridgetText;
bFinished true;
Lab assignment
Check if the game is a tie
Step by Step Solution
There are 3 Steps involved in it
Step: 1
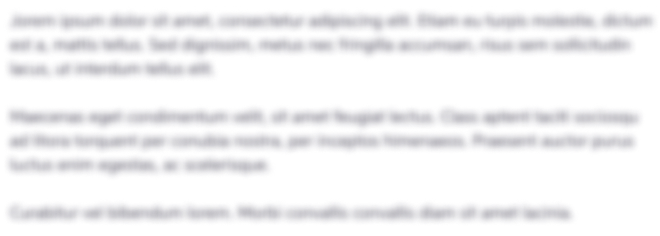
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started