Question
package Lab6; public abstract class Container { // do not change the value of the following constant. protected final int ORIGINAL_SIZE = 10; protected Object[]
package Lab6;
public abstract class Container { // do not change the value of the following constant. protected final int ORIGINAL_SIZE = 10; protected Object[] list; // is a container that stores the element of MyList protected Object[] set; // is a container that stores the element of MySet protected int size; // this variable holds the actual number of elements that are stored in either of the containers (i.e. MyList or MySet). /** * This method adds the obj
to the end of the container. * @param obj is the object that is added to the container. */ void add(Object obj) { System.out.println("The object was added to the contianer"); } /** * This method removes the object at the given index * @param index is the index of the object that is removed. * @return returns the removed object. */ Object remove(int index) { // Change the return value. return index; }
/** * This method returns true if the container is empty. * @return It returns true if the container is empty, otherwise false. */ boolean isEmpty() { return size == 0 ? true: false; } /** * This method returns the number of elements stored in the container. * @return It returns the number of elements in the container. */ int getSize() { return size; }
}
/** * * This class simulates an ArrayList, where you can add unlimited number of * elements to the list. * */ class MyList extends Container{ /** * This is the default constructor that sets all the instance variables to their defualt value. */ public MyList () { list= new Object[ORIGINAL_SIZE]; size = 0; } /** * This method returns the element that is stored at index index
. * @param index is the index
at which the element is accessed and returned. * @return it returns the element stored at the given index
. */ public Object get(int index) { Object obj = list[index]; return obj; } /** * This method overrides the add
method defined in class container
, by * adding the obj
to the back of list
array. * The original size of the array
, is defined by ORIGINAL_SIZE
, however, it is possible that * more elements is added to this array. In case the array does not have enough capacity to add one more element, it grows itself * by doubling the size of list
array. */ @Override void add(Object obj) { if (size index is in a valid range. */ @Override Object remove(int index) { // implement this code. You many need to change the return value return index; }
/** * This method returns the elements of the MyList in a form of * [obj1 obj2 obj3 ...] */ @Override public String toString() { String result= "["; for(int i = 0; i class MySet extends Container{ public MySet() { set = new Object[ORIGINAL_SIZE]; size = 0; } /** * This method overrides the /** * This method returns the elements of the MySet in a form of * [obj1 obj2 obj3 ...] */ @Override public String toString() { String result= "["; for(int i = 0; i /** * This class implements a Stack. * */ class Stack{ private Container stack; // no other instance variable should be defined here. /** * This is the constructor that initializes } /** * This method removes an object from the top of the stack. * @return It returns the object that is returned from the top of the stack. */ public Object pop() { // insert your code here. only one line should be added here. You may need to change the return value. return new Object(); } /** * This method returns the object, which is at the top of the stack without removing it from the stack. * @return It returns the element, which is found on top of the stack. */ public Object top() { // insert your code here. only one line should be added here. You may want to change the return value. return new Object(); } /** * This method shows how many elements are in the stack. * @return It returns the number of elements in the stack. */ public int getSize() { // change the return value. No any other code should be added. return this.stack.size; } } add
method defined in class container
, by * adding the obj
to the back of set
array. * The original size of the set
, is defined by ORIGINAL_SIZE
, however, it is possible that * more elements is added to this set. In case the set does not have enough capacity to add one more element, it grows itself * by doubling the size of set
array. */ @Override void add(Object obj) { // if the array is full, double its size if (size == set.length ) { Object[] setCopy = new Object[2*set.length]; for(int i = 0; i stack
. */ public Stack() { // insert your code here. only one line should be added here. } /** * This method adds the object to the top of the stack. * @param object is the object that is added to the top of the stack. */ public void push(Object object) { // insert your code here. only one line should be added here.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
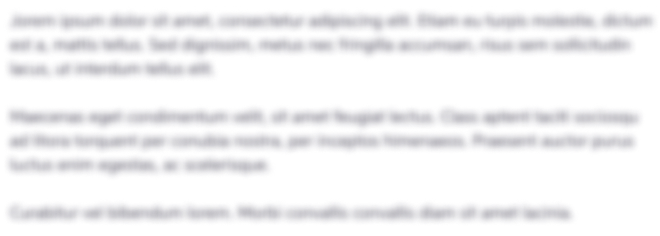
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started