Question
package option1.stage1; import java.util.ArrayList; import java.util.Arrays; public class Doctor { private String name; private String speciality; /* * note specialities is static means that only
package option1.stage1;
import java.util.ArrayList;
import java.util.Arrays;
public class Doctor {
private String name;
private String speciality;
/*
* note specialities is static means that only one instance is created
* irrespective of number of Doctor instances and that one instance of
* specialities is shared by all instances. it can be accessed by Doctor.specialities
*/
public static ArrayList
"Pediatrician", "Podiatrician", "Dermatologist",
"Radiologist", "Pathologist", "Physician",
"Obstetrician", "Gynaecologist", "Oncologist"));
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSpeciality() {
return speciality;
}
/**
* set speciality of the calling object to the passed value IF the value
* exists (case insensitive) in the ArrayList specialities.
* If the passed value doesn't exist in the ArrayList specialities, set
* the speciality of the calling object to "General Practitioner".
* For example, if spec = "oncologist" or if spec = "Oncologist" or spec = "ONCOLOGIST",
* instance variable speciality should become "Oncologist"
* @param spec
*/
public void setSpeciality(String spec) {
//TO BE COMPLETED
}
public Doctor(String name, String spec) {
setName(name);
setSpeciality(spec);
}
public String toString() {
return "Dr. "+name+" ("+speciality+")";
}
/**
*
* @param other
* @return true if the names and specialities of the calling object and the parameter object
* are the same (case insensitive)
*/
public boolean equals(Object other) {
if(other instanceof Doctor)
return name.equalsIgnoreCase(((Doctor)other).name) && speciality.equalsIgnoreCase(((Doctor)other).speciality);
else
return false;
}
}
DOCTORTEST.JAVA:
package option1.stage1;
import common.Graded;
import static org.junit.Assert.*;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Date;
import java.text.SimpleDateFormat;
import org.junit.AfterClass;
import org.junit.Test;
import option1.stage1.*;
public class DoctorTest {
private static int score = 0;
private static String result = "";
@Test @Graded(marks=5, description="Doctor:setSpeciality(String) (5 marks)")
public void testSetSpeciality() {
Doctor d = new Doctor("G", "Pediatrician");
assertEquals("Pediatrician", d.getSpeciality());
d = new Doctor("G", "oncologist");
assertEquals("Oncologist", d.getSpeciality()); //Uppercase 'O'
d = new Doctor("G", "supernintendo chalmers");
assertEquals("General Practitioner", d.getSpeciality());
score+=5;
result+="Doctor:setSpeciality(String) passed (5 marks) ";
}
//log reporting
@AfterClass
public static void wrapUp() throws IOException {
System.out.println(result.substring(0,result.length()-1));
System.out.println("--------------------------------------------");
System.out.println("Total: "+score+" out of 5");
System.out.println("-------------------------------------------- ");
String timeStamp = new SimpleDateFormat("yyyy-MM-dd-HH-mm-ss").format(new Date());
File file = new File("log/Doctor"+timeStamp+".txt");
FileWriter writer = new FileWriter(file);
writer.write(result.substring(0,result.length()-1)+" ");
writer.write("-------------------------------------------- ");
writer.write(score+" ");
writer.flush();
writer.close();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
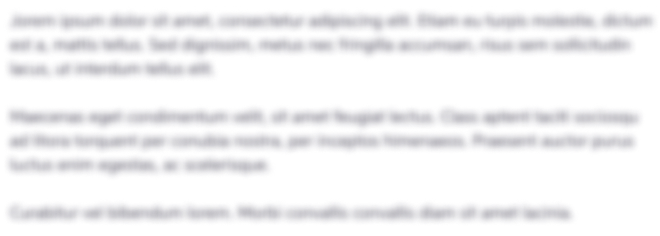
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started