Question
package option2; import java.util.ArrayList; public class Matrix { private ArrayList < ArrayList > data; /** * DO NOT MODIFY * construct an empty matrix */
package option2;
import java.util.ArrayList;
public class Matrix {
private ArrayList< ArrayList
/**
* DO NOT MODIFY
* construct an empty matrix
*/
public Matrix() {
data = new ArrayList< ArrayList
}
/**
* construct an n by n matrix filled with zeroes
* @param n
*/
public Matrix(int n) {
}//TO BE COMPLETED
/**
* create a matrix of nRows rows and nCols columns
* @param nRows
* @param nCols
*/
public Matrix(int nRows, int nCols) {
//TO BE COMPLETED
}
/**
*
* assumption values is a valid string
* (contains double values separated by space)
* For example, "1.5 2.4 5.6 7.1 4 7.8"
*
* If the number of items in values is square
* of an integer, it creates a square matrix.
* For example, "1 2 3 4" would create matrix
* 1 2
* 3 4
*
* If the number of items in values is not square
* of an integer, it tries to create a matrix of size
* 2 by (values.length/2). If not possible then,
* 3 by (values.length/3). If not possible then,
* ...
* and in the worst case scenario, it creates a matrix
* of size values.length by 1
*
* For example, "1 2 3 4 5 6" would create matrix
* 1 2 3
* 4 5 6
*
* while "1 2 3 4 5 6 7" would create the matrix
* 1
* 2
* 3
* 4
* 5
* 6
* 7
*
* @param values
*/
public Matrix(String values) {
data = new ArrayList< ArrayList
//TO BE COMPLETED
String[] numbers = values.split(" ");
int size = numbers.length;
int rows;
int cols;
if(Math.sqrt(size) - (int)Math.sqrt(size) == 0)
{
rows = (int)Math.sqrt(size);
cols = rows;
}
else
{
int n = 2;
do
{
cols = size / n;
rows = n;
n++;
}
while(rows * cols != size);
}
int k = 0;
for(int i = 0; i < rows; i++)
{
ArrayList
for(int j = 0; j < cols; j++)
{
temp.add(Double.parseDouble(numbers[k]));
k++;
}
data.add(temp);
}
}
/**
* construct the matrix as a clone of the source matrix
* @param source
*/
public Matrix(Matrix source) {
this.data = source.data;
}
/**
* DO NOT MODIFY
* @param row
* @param column
* @return the value at given row and column if any.
* return null if the location is invalid
*/
public Double get(int row, int column) {
if(!isValid(row, column))
return null;
return data.get(row).get(column);
}
/**
* DO NOT MODIFY
* @return number of rows
*/
public int rowCount() {
// TODO Auto-generated method stub
if(data == null)
return 0;
return data.size();
}
/**
* DO NOT MODIFY
* @return number of columns
*/
public int columnCount() {
// TODO Auto-generated method stub
if(data == null)
return 0;
if(data.size() == 0)
return 0;
return data.get(0).size();
}
/**
* DO NOT MODIFY
* @return true if matrix is a square matrix, false otherwise
*/
public boolean isSquare() {
return rowCount() == columnCount();
}
/**
* DO NOT MODIFY
* return String representation of the matrix
*/
public String toString() {
String result = "";
for(ArrayList
result+="| ";
for(Double item: list) {
result += item+" ";
}
result+="| ";
}
result+=" ";
return result;
}
/**
*
* @return true if calling object is a zero matrix,
* false otherwise
*/
public boolean isZero() {
return false; //TO BE COMPLETED
}
/**
*
* @return if calling object is a diagonal matrix,
* false otherwise
*/
public boolean isDiagonal() {
return false; //TO BE COMPLETED
}
/**
*
* @return true if calling object is an identity matrix,
* false otherwise
*/
public boolean isIdentity() {
return false; //TO BE COMPLETED
}
/**
*
* @param incValue
* @return a matrix that represents calling matrix shifted by incValue.
*/
public Matrix scalarShift(double incValue) {
return null; //TO BE COMPLETED
}
/**
*
* @param factor
* @return a matrix that represents calling matrix that
* undergoes scalar multiplication with factor
*/
public Matrix scalarMultiplication(double factor) {
return null; //TO BE COMPLETED
}
/**
*
* @param other
* @return matrix representing vector addition of the calling object and parameter object
*/
public Matrix vectorAddition(Matrix other) {
return null; //TO BE COMPLETED
}
/**
*
* @param other
* @return matrix representing vector subtraction of the parameter object from calling object
* that is, (calling matrix) - (parameter matrix)
*/
public Matrix vectorSubtraction(Matrix other) {
return null; //TO BE COMPLETED
}
/**
*
* @param row
* @param column
* @return true if the passed address is valid, false otherwise
*/
public boolean isValid(int row, int column) {
return false; //TO BE COMPLETED
}
/**
*
* @param row
* @param column
* @param value
* @return if the row and column passed are valid, set the value at that location
* to the passed value and return true, and if they are not valid, return false
*/
public boolean set(int row, int column, double value) {
return false; //TO BE COMPLETED
}
/**
*
* @param other
* @return matrix representing calling matrix multiplied by parameter matrix
*/
public Matrix vectorMultiplication(Matrix other) {
return null; //TO BE COMPLETED
}
/**
*
* @return determinant of the matrix.
* if the matrix is not a square matrix, return 0.
* if row count is zero, return 0.
*/
public double determinant() {
return 0; //TO BE COMPLETED
}
/**
*
* @return true if matrix is invertible, false otherwise
*/
public boolean isInvertible() {
return false; //TO BE COMPLETED
}
/**
*
* @param row
* @param column
* @return return minor of the matrix for the given address
*/
public Matrix minor(int row, int column) {
return null; //TO BE COMPLETED
}
/**
*
* @return cofactor of the matrix
*/
public Matrix cofactor() {
return null; //TO BE COMPLETED
}
/**
*
* @return transpose of the matrix
*/
public Matrix transpose() {
return null; //TO BE COMPLETED
}
/**
*
* @return inverse of the matrix
*/
public Matrix inverse() {
return null; //TO BE COMPLETED
}
/**
*
* @param other
* @return true if calling matrix and parameter matrix are identical, false otherwise
*/
public boolean equals(Matrix other) {
return false; //TO BE COMPLETED
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
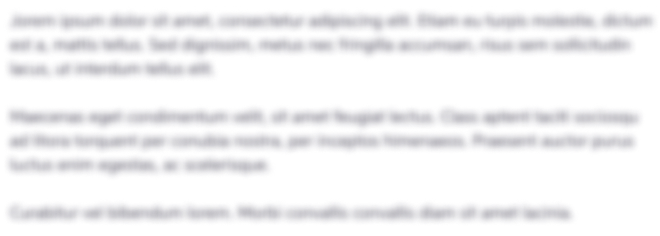
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started