Question
package scheduler; import java.util.List; public class Scheduler { /** * Instantiates a new, empty scheduler. */ public Scheduler() { } /** * Adds a course
package scheduler;
import java.util.List;
public class Scheduler {
/**
* Instantiates a new, empty scheduler.
*/
public Scheduler() {
}
/**
* Adds a course to the scheduler.
*
* @param course the course to be added
*/
public void addCourse(Course course) {
}
/**
* Returns the list of courses that this scheduler knows about.
*
* This returned object does not share state with the internal state of the Scheduler.
*
* @return the list of courses
*/
public List
return null;
}
/**
* Adds a student to the scheduler.
*
* @param student the student to add
*/
public void addStudent(Student student) {
}
/**
* Returns a list of the students this scheduler knows about.
*
* This returned object does not share state with the internal state of the Scheduler.
* @return
*/
public List
return null;
}
/**
* Assigns all students to courses in the following manner:
*
* For a given student, check their list of preferred courses. Add them to the course that:
* - exists in the scheduler's list of courses
* - the student most prefers (that is, comes first in their preference list)
* - the student is not not already enrolled in
* - and is not full (in other words, at capacity)
* Adds courses to the *end* of the student's current list of classes. Adds students to
* the *end* of the course's roster.
*
* Repeat this process for each student, one-by-one; each student will now have one course,
* usually (but not always) their most preferred course.
*
* Then repeat this whole process (adding one course per student, when possible, proceeding
* round-robin among students), until there is nothing left to do: Students might
* all be at their maximum number of courses, or there may be no available seats in courses
* that students want.
*/
public void assignAll() {
}
/**
* Drops a student from a course.
*
* @param student
* @param course
* @throws IllegalArgumentException if either the student or the course are not known to this scheduler
*/
public void drop(Student student, Course course) throws IllegalArgumentException {
}
/**
* Drops a student from all of their courses.
*
* @param student
* @throws IllegalArgumentException if the student is not known to this scheduler
*/
public void unenroll(Student student) throws IllegalArgumentException{
}
}
---------------------------------------------------------------------
package scheduler;
import java.util.List;
/**
* A class representing a student.
*
* @author liberato
*
*/
public class Student {
/**
*
* Instantiates a new Student object. The student's maximum course load must be greater
* than zero, and the preferences list must contain at least one course.
*
* The preference list is copied into this Student object.
*
* @param name the student's name
* @param maxCourses the maximum number of courses that can be on this student's schedule
* @param preferences the student's ordered list of preferred courses
* @throws IllegalArgumentException thrown if the maxCourses or preferences are invalid
*/
public Student(String name, int maxCourses, List
}
/**
*
* @return the student's name
*/
public String getName() {
return null;
}
/**
*
* @return the student's max course load
*/
public int getMaxCourses() {
return -1;
}
/**
* Returns the student's list of course preferences, ordered from most- to least-desired.
*
* This returned object does not share state with the internal state of the Student.
*
* @return the student's preference list
*/
public List
return null;
}
/**
* Returns the student's current schedule.
*
* This returned object does not share state with the internal state of the Student.
*
* @return the student's schedule
*/
public List
return null;
}
}
---------------------------------------------------------------------------
package scheduler;
import java.util.List;
/**
* A class representing a Course.
*
* @author liberato
*
*/
public class Course {
/**
* Instantiates a new Course object. The course number must be non-empty, and the
* capacity must be greater than zero.
* @param courseNumber a course number, like "COMPSCI190D"
* @param capacity the maximum number of students that can be in the class
* @throws IllegalArgumentException thrown if the courseNumber or capacity are invalid
*/
public Course(String courseNumber, int capacity) throws IllegalArgumentException {
}
/**
*
* @return the capacity of the course
*/
public int getCapacity() {
return -1;
}
/**
*
* @return the course number
*/
public String getCourseNumber() {
return null;
}
/**
* Returns the list of students enrolled in the course.
*
* This returned object does not share state with the internal state of the Course.
*
* @return the list of students currently in the course
*/
public List
return null;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
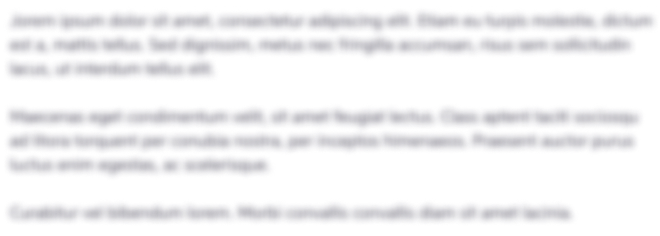
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started