Question
package Shopping; import java.util.*; /** * This is the file where you will do most of your work. You are responsible for * implementing remove(),
package Shopping;
import java.util.*;
/** * This is the file where you will do most of your work. You are responsible for * implementing remove(), find(), and contains(). Feel free to use the Array * based implementation as a base to figure out what you need to do * * @version Spring 2021 * @author ITCS 2214 */ public class ShoppingListArrayList implements ShoppingListADT {
private ArrayList
/** * Default constructor for creating an empty shopping list */ public ShoppingListArrayList() { this.shoppingList = new ArrayList(); }
/** * Method to add a new entry to the shopping list. If a matching item already * exists, the quantities are combined * * @param entry the new item to add */ @Override public void add(Grocery entry) { if (entry == null) { return; }
// Check if this item already exists if (this.contains(entry)) { // Merge the quantity of new entry into existing entry this.combineQuantity(entry); return; }
this.shoppingList.add(entry); }
/** * Method to remove a specific entry from the shopping list * * @param entry the item to remove * @return true if the operation was completed successfully */ @Override public boolean remove(Grocery entry) {
// the boolean found describes whether or not we find the // entry to remove boolean found = false;
// TODO Search the parameter variable ent in the shoppingList; // if it is found, please remove it and update the value of `found`. // It is okay to directly use methods from the Java Build-in ArrayList class.
// Return false if not found
}
/** * Method to find a specific item in the shopping list * * @param index the index of the item in the shopping list * @return the entry at the specified index * @throws IndexOutOfBoundsException if the index is out of bounds * @throws EmptyCollectionException if the shopping list is empty */ @Override public Grocery find(int index) throws IndexOutOfBoundsException, EmptyCollectionException { return null; // TODO throw EmptyCollectionException if shopping list is empty
// TODO check whether or not the given index is legal // for example, the given index is less than 0 or falls outside of the size. // If it is not legal, throw an IndexOutOfBoundsException
// return the corresponding entry in the shoppingList
}
/** * Method to find the index of a Grocery item that is equivalent to the one * provided * * @param entry the item to find * @return the index of the specified item in the shopping list * @throws ElementNotFoundException if the specified item is not in the shopping * list */ @Override public int indexOf(Grocery entry) throws ElementNotFoundException { if (entry != null) { for (int i = 0; i
/** * Method to determine the existence of a specific item in the shopping list * * @param entry the item to find * @return true if the specified item exists in the shopping list, otherwise * false */ @Override public boolean contains(Grocery entry) { boolean hasItem = false;
if (entry != null) { // TODO go through the shoppingList and try to find the // given item, named entry, in the list. If found, return true. Either using // methods from Java build-in ArrayList method or writing your own loop is fine.
} return hasItem; }
/** * Method to get the size of the shopping list * * @return the size of the shopping list */ @Override public int size() { return shoppingList.size(); }
/** * Method to tell if the shopping list is empty * * @return true if the shopping list is empty, otherwise false */ @Override public boolean isEmpty() { return shoppingList.isEmpty(); }
/** * Method to return a string representation of the shopping list * * @return the string representation of the shopping list */ @Override public String toString() { StringBuilder s = new StringBuilder(); s.append(String.format("%-25s", "NAME")); s.append(String.format("%-18s", "CATEGORY")); s.append(String.format("%-10s", "AISLE")); s.append(String.format("%-10s", "QUANTITY")); s.append(String.format("%-10s", "PRICE")); s.append(' '); s.append("------------------------------------------------------------" + "-------------"); s.append(' '); for (int i = 0; i
return s.toString(); }
/** * Method to add the quantity of duplicate entries in the shopping list * * @param entry duplicate entry */ private void combineQuantity(Grocery entry) { try { int index = this.indexOf(entry); this.shoppingList.get(index).setQuantity(this.shoppingList.get(index).getQuantity() + entry.getQuantity()); } catch (ElementNotFoundException e) { System.out.println("combineQuantity - ECE"); }
}
}
Objectives At the completion of this assignment, you will have completed an ArrayList implementation of a ShoppingList interface, and completed unit testing of the implementation. By completing this project, you will have gained experience in understanding the difference between interface and implementation, a critical part of encapsulation. You will get experience writing JUnit test cases for methods, to make sure the methods work as expected. You will also become accustomed to the Web-Cat system for auto-grading of code projects and tests. This system may seem frustrating at first, but in industry you will have to check in code that you write to similar systems that will run your code against a bunch of test cases. You can also observe the use of exceptions, how they are passed and handled and how you work with them when writing test cases. Instructions During this assignment, you will be working with an interface for shopping lists. We provide a completed array implementation of this interface, and an incomplete ArrayList implementation. Your job is to complete the ArrayList implementation. Both implementations adhere to the ShoppingListADT interface. The shopping lists hold Grocery items, and there is a Grocery class that defines what a Grocery item is. While duplicate grocery items are not allowed in the list, the quantities of a grocery item are combined when an attempt is made to add a duplicate Grocery. Through the Grocery class's compareTo method, entries are determined as duplicates if their name and category match, regardless of letter case (ie. "bread" is the same as "Bread). Requirements You must fulfill the following requirements in your implementation. 1. Complete the three methods (remove(), find(), and contains() in the ShoppingListArrayList.java file. These methods are already implemented in the ShoppingListArray class. Fill in missing statements in these three methods. The comments starting with "TODO" indicates the missing statements. 2. Ensure all methods in both implementations have appropriate JavaDoc comments. 3. Generate the JavaDoc html files. 4. Complete the ShoppingListArrayListTest.java (Please complete the testAdd() method, testRemove() method, and testFind(). When you first import the project, it will run using the array implementation of ShoppingList. After completing the methods described in the first requirement, the project can be run using the ArrayList implementation. Commented code is provided for switching to this version of the simulation in Shopping Simulation.java. The UML class diagram is as follows: Grocery - String nane -String category Sintaisie - Hout price in quantityStep by Step Solution
There are 3 Steps involved in it
Step: 1
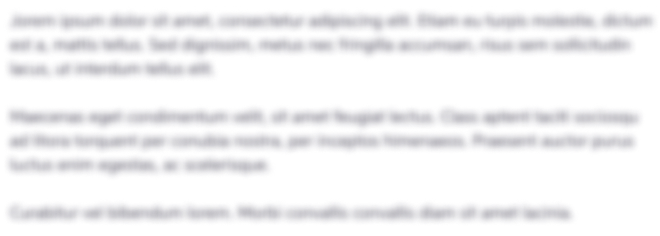
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started