Answered step by step
Verified Expert Solution
Question
1 Approved Answer
package sll; import java.util.NoSuchElementException; /** * This class provides a basic implementation of a singly linked list. It's * motivated by the implementation in Big
package sll; import java.util.NoSuchElementException; /** * This class provides a basic implementation of a singly linked list. It's * motivated by the implementation in Big Java, 5e, ch. 16.1. * * @author Xusheng "Fred" Liu on . * @param * The type of elements in the list */ public class LinkedList implements List, Iterable { private ListNode first; // Note that in addition to a head (first) pointer // this list contains a last pointer. Be sure to // BOTH first and last up to date in all your code. private ListNode last; /** * Constructs a new, empty linked list. */ public LinkedList() { this.first = null; this.last = null; } @Override public String toString() { if (this.first == null) { return "[]"; } String result = "["; ListNode current = this.first; while (current != this.last) { result += (current.getElement() + ", "); current = current.getNext(); } return result + current.getElement() + "]"; } @Override public boolean add(T x) { ListNode newNode = new ListNode(x); if (newNode == null) { } else { newNode.setNext(null); if (this.first == null) { this.first = newNode; this.last = newNode; } else { this.last.setNext(newNode); this.last = newNode; } } return true; } @Override public int size() { // TODO 02 Implement the size() method. int size = 0; ListNode temp = this.first; for (temp = this.first; temp != null; temp = temp.getNext()) { size++; } return size; } @Override public void add(int i, T x) throws IndexOutOfBoundsException { // TODO 03 Implement the add(int i, T x) method. // Note that this adds at the specific index ListNode newNode = new ListNode(x); if (newNode == null) { } else { newNode.setNext(null); if (this.first == null) { this.first = newNode; this.last = newNode; } else { this.last.setNext(newNode); this.last = newNode; } } return true; } @Override public boolean contains(T x) { // TODO 04 Implement the contains(T x) method. return false; } @Override public boolean remove(T x) { // TODO 05 Implement the remove(T x) method. return true; } @Override public T get(int index) throws IndexOutOfBoundsException { // TODO 06 Implement the get(int index) method. return null; } @Override public int indexOf(T x) { // TODO 07 Implement the indexOf(T x) method. return -1; } @Override public T set(int index, T element) throws IndexOutOfBoundsException { // TODO 08 Implement the set(int index, T element) method. return null; } @Override public Iterator iterator() { return new LinkedListIterator(); } /** * This is an iterator over this list. * * @author TODO on . */ private class LinkedListIterator implements Iterator { private ListNode current, previous; private LinkedListIterator() { this.current = null; this.previous = null; } @Override public boolean hasNext() { // TODO 09 Implement the hasNext() method. return false; } @Override public T next() throws NoSuchElementException { // TODO 10 Implement the next() method. return null; } @Override public void remove() { // TODO 11 Implement the remove() method. } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
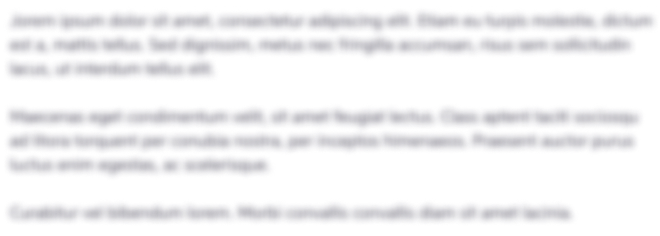
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started