Question
Package Write a fully-documented class named Package which represents the mail packages that are being delivered and picked up from from the mailroom. The Package
Package
Write a fully-documented class named Package which represents the mail packages that are being delivered and picked up from from the mailroom. The Package class will have the following member variables (each with Getters and Setters):
private String recipient
private int arrivalDate
The arrival date will start at day 0 (see sample IO).
private double weight
public Package(String recipient, int arrivalDate, double weight)
Default constructor of the Package class
Postconditions:
This object has been initialized to a package object with specified recipient, arrival date and weight.
PackageStack
Write a fully-documented class named PackageStack. For this homework assignment, you are allowed to use built-in Java libraries for your stack. You may choose to extend a stack (ArrayList
private final int CAPACITY = 7
public void push(Package x)
Pushes x onto the top of the backing data structure.
Throws:
FullStackException if the stack is at capacity.
If you are extending LinkedList, you may rename this pushPackage(Package x) if your IDE is complaining about throwing a FullStackException
public Package pop()
Removes the topmost package from the stack and returns it.
Throws:
EmptyStackException if the stack was empty.
If you are extending LinkedList, you may rename this popPackage() if your IDE is complaining about throwing a EmptyStackException
public Package peek()
Returns the topmost Package from the stack without removing it. The stack should be unchanged as a result of this method.
Throws:
EmptyStackException if the stack was empty
public boolean isFull()
Returns true if the stack is full, false otherwise.
Public boolean isEmpty()
Returns true if the stack is empty, false otherwise.
Documentation for Javas implementation of LinkedList and ArrayList can be found here: https://docs.oracle.com/javase/7/docs/api/java/util/LinkedList.html
https://docs.oracle.com/javase/7/docs/api/java/util/ArrayList.html
MailroomManager
Write a fully-documented driver class named MailroomManager which contains 6 package stacks for storing mail. Packages can be added to stacks, removed from stacks, and moved from one stack to another. Mail will be stored according to the following criteria: Packages whose recipients name begins with A-G will be stored in the first stack, H-J in second, K-M in the third, N-R in the fourth, and S-Z in the fifth. The sixth stack will serve as the Floor stack, which is used when moving packages of a specific recipient.
public static void main(String[] args)
The main method runs a menu driven application which allows the user to create six instances of the PackageStack class and then prompts the user for a menu command selecting the operation. The required information is then requested from the user based on the selected operation.
FullStackException
An Exception class that is thrown when a stack has reached its Capacity (the FloorStack has no capacity)
EmptyStackException
An Exception class that is thrown when trying to pop from a stack with no elements.
Note on Exceptions: all exceptions should be handled gracefully - they should be caught in the main, and the user should be notified by a nice printout. Your messages will not be graded for creativity, but they should clearly indicate what the problem is (bad index, full list, negative number, etc.). The program should continue to run normally after an exception is encountered. We will not be checking Input Mismatch cases.
UI Required Functions
Note: please make sure that the menu is NOT case sensitive (so selecting A should be the same as selecting a).
D - Deliver Package
G - Get Packages for a user - gets the topmost package for a user
T - Make it tomorrow
P - print the stacks (format for a package is [Name arrivalDate]
M - move a package from one stack to another
F - Find packages in the wrong stack and move to Floor (note: just like in a teenager's room, the Floor never becomes full)
L - List all the packages awaiting a user
E- Empty the floor, moving all packages to the trash.
Q - Quit
Program Sample
Welcome to the Irving Mailroom Manager. You can try to make it better, but the odds are stacked against you. It is day 0.
Menu:
D) Deliver a package
G) Get someone's package
T) Make it tomorrow
P) Print the stacks
M) Move a package from one stack to another
F) Find packages in the wrong stack and move to floor
L) List all packages awaiting a user
E) Empty the floor.
Q) Quit
Please select an option: D
Please enter the recipient name: Jones
Please enter the weight (lbs): 2
A 2 lb package is awaiting pickup by Jones.
Please select an option: D
Please enter the recipient name: Johnson
Please enter the weight (lbs): 5
A 5 lb package is awaiting pickup by Johnson.
Please select an option: D
Please enter the recipient name: Johnson
Please enter the weight (lbs): 6
A 6 lb package is awaiting pickup by Johnson.
Please select an option: D
Please enter the recipient name: Ivanov
Please enter the weight (lbs): 3
A 3 lb package is awaiting pickup by Ivanov.
Please select an option: P
Current Packages:
--------------------------------
Stack 1 (A-G):|empty.
Stack 2 (H-J):|[Jones 0][Johnson 0][Johnson 0][Ivanov 0]
Stack 3 (K-M):|empty.
Stack 4 (N-R):|empty.
Stack 5 (S-Z):|empty.
Floor: |empty.
Please select an option: T
It is now day 1.
Please select an option: D
Please enter the recipient name: Jones
Please enter the weight (lbs): 3
A 3 lb package is awaiting pickup by Jones.
Please select an option: D
Please enter the recipient name: Ivanov
Please enter the weight (lbs): 1
A 1 lb package is awaiting pickup by Ivanov.
Please select an option: P
Current Packages:
--------------------------------
Stack 1 (A-G):|empty.
Stack 2 (H-J):|[Jones 0][Johnson 0][Johnson 0][Ivanov 0][Jones 1][Ivanov 1]
Stack 3 (K-M):|empty.
Stack 4 (N-R):|empty.
Stack 5 (S-Z):|empty.
Floor: |empty.
Please select an option: L
Please enter the recipient name: Jones
Jones has 2 packages total.
Package 1 is in Stack 2, it was delivered on day 0, and weighs 2 lbs.
Package 2 is in Stack 2, it was delivered on day 1, and weighs 3 lbs.
Please select an option: G
Please enter the recipient name: Johnson
Move 3 packages from Stack 2 to floor.
Current Packages:
--------------------------------
Stack 1 (A-G):|empty.
Stack 2 (H-J):|[Jones 0][Johnson 0][Johnson 0]
Stack 3 (K-M):|empty.
Stack 4 (N-R):|empty.
Stack 5 (S-Z):|empty.
Floor: |[Ivanov 1][Jones 1][Ivanov 0]
Give Johnson 6 lb package delivered on day 0.
Return 3 packages to stack 2 from floor.
Current Packages:
--------------------------------
Stack 1 (A-G):|empty.
Stack 2 (H-J):|[Jones 0][Johnson 0][Ivanov 0][Jones 1][Ivanov 1]
Stack 3 (K-M):|empty.
Stack 4 (N-R):|empty.
Stack 5 (S-Z):|empty.
Floor: |empty.
Please select an option: D
Please enter the recipient name: Jordan
Please enter the weight (lbs): 1.5
A 1.5 lb package is awaiting pickup by Jordan.
Please select an option: D
Please enter the recipient name: Jacobs
Please enter the weight (lbs): 1
A 1 lb package is awaiting pickup by Jacobs.
Please select an option: P
Current Packages:
--------------------------------
Stack 1 (A-G):|empty.
Stack 2 (H-J):|[Jones 0][Johnson 0][Ivanov 0][Jones 1][Ivanov 1][Jordan 1][Jacobs 1]
Stack 3 (K-M):|empty.
Stack 4 (N-R):|empty.
Stack 5 (S-Z):|empty.
Floor: |empty.
Please select an option: D
Please enter the recipient name: Harken
Please enter the weight (lbs): 4
A 4 lb package is awaiting pickup by Harken. As stack 2 was full, it was placed in stack 1.
//Stack 3 would have been acceptable too. Try all stacks 1 away, then all 2 away
Please select an option: M
Please enter the source stack (enter 0 for floor): 2
Please enter the destination stack: 3
Please select an option: P
Current Packages:
--------------------------------
Stack 1 (A-G):|[Harken 1]
Stack 2 (H-J):|[Jones 0][Johnson 0][Ivanov 0][Jones 1][Ivanov 1][Jordan 1]
Stack 3 (K-M):|[Jacobs 1]
Stack 4 (N-R):|empty.
Stack 5 (S-Z):|empty.
Floor: |empty.
Please select an option: T
It is now day 2.
Please select an option: T
It is now day 3.
Please select an option: T
It is now day 4.
Please select an option: F
Misplaced packages moved to floor.
Please select an option: P
Current Packages:
--------------------------------
Stack 1 (A-G):|empty.
Stack 2 (H-J):|[Jones 0][Johnson 0][Ivanov 0][Jones 1][Ivanov 1][Jordan 1]
Stack 3 (K-M):|empty.
Stack 4 (N-R):|empty.
Stack 5 (S-Z):|empty.
Floor: |[Harken 1][Jacobs 1]
//packages from stack 1 should be before stack 2, followed by stack 3 ...
Please select an option: T
It is now day 5. 3 packages have been returned to sender.
Please select an option: P
Current Packages:
--------------------------------
Stack 1 (A-G):|empty.
Stack 2 (H-J):|[Jones 1][Ivanov 1][Jordan 1]
Stack 3 (K-M):|empty.
Stack 4 (N-R):|empty.
Stack 5 (S-Z):|empty.
Floor: |[Harken 1][Jacobs 1]
Please select an option: E
The floor has been emptied. Mr. Trash Can is no longer hungry.
Please select an option: P
Current Packages:
--------------------------------
Stack 1 (A-G):|empty.
Stack 2 (H-J):|[Jones 1][Ivanov 1][Jordan 1]
Stack 3 (K-M):|empty.
Stack 4 (N-R):|empty.
Stack 5 (S-Z):|empty.
Floor: |empty.
Please select an option: Q
Use Amazon Locker next time.
(A-G, H-J, K-M, N-R, S-Z)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
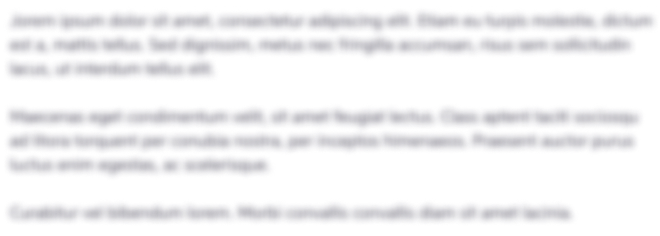
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started