Question
Part 1: Adding a Manufacturer and AutoModel Class Provide a Manufacturer class that stores the manufacturer's name and country. This class (along with all other
Part 1: Adding a Manufacturer and AutoModel Class
Provide a Manufacturer class that stores the manufacturer's name and country. This class (along with all other classes specified in this assignment) should provide a suitable constructor, appropriate access modifiers, and getter methods. Additionally, override the toString() such that instances of the Manufacturer object are rendered as follows:
(recall that anything in <..> is replaced by its corresponding field).
Now add a class to represent an automobile's model (AutoModel). This class should be able to store the name of the model, whether or not it is still in production, and a list of Year objects denoting the years it was in production. In the constructor, include a defensive check that ensures the passed-in year list is nonempty (if it is, throw an IllegalArgumentException).
Now override the toString() method to render AutoModel objects as follows:
Note: that you do not need to store trims anymore (or be able to add trims anymore).
Part 2: Stubbing out a Vehicle Class Hierarchy
Now create empty classes for a Sedan, Vehicle, and Truck. Note that you do NOT have to create the Comparable interface yourself -- it already exists in Java's libraries (you'll just be implementing a method the interface exports in Part 3).
Here are some general notes to follow when writing these classes:
the Sedan and Truck classes should extend the Vehicle class,
the Vehicle class must include an abstract method, numberOfWheels, which takes nothing as a formal parameter and returns an int,
a constructor in each subclass must invoke a super(..) constructor -- no empty/default constructors are needed in this assignment,
The Sedan should be able to store:
the manufacturer (use your Manufacturer class),
the model (use your AutoModel class),
the sedan's mpg.
Implement the numOfWheels method -- it should always return 4. Provide a toString() that resembles the following:
(
The Truck should be able to store:
the manufacturer,
whether or not the truck is dually,
the model,
mpg,
Implement the numberOfWheels method such that it returns 6 if the truck is a dually and 4 if it isn't. Now provide an overloaded constructor that invokes the full constructor using the this(..) command. The shorter, overloaded constructor should assume that the Truck being constructed is not a dually.
Override toString() to print the additional information as follows:
(
More on The Vehicle Class:
You will need to determine what should be stored in this class based on what you identify as the fields and methods common between both types of vehicles. Once you determine what to store in the vehicle base class, create a suitable constructor (and the subclasses should "super" up the required information).
The vehicle class should provide getter methods for:
each field that you determine should be stored in vehicle (based on observed duplication in the subclasses)
a getter method to retrieve the release year of the vehicle (use Java's Year class as the return type)
hint: the constructor for Vehicle should NOT take a parameter of type Year directly, rather, it should be obtained via the AutoModel class (this suggests at least one field that the Vehicle class should store)
The class should contain the abstract method, numberOfWheels (specified above), and another method, howFarWith(int numOfGallons) that returns how many miles the car will go on the given number of gallons.
Part 3: Making Vehicles Comparable
Now you will make your Vehicle class implement the Comparable interface. Here's a snippet of the relevant change to the header for your Vehicle class:
public abstract class Vehicle implements Comparable{
Once you add this, you'll now need to override/implement the interface's compareTo(..) method. We'll define the natural ordering for Vehicles by their release year. Consult class notes for a summary of the compareTo(..) method contract. Essentially, the method has formal parameter o of type Vehicle and should return an int x, where:
x < 0 if this vehicle's release year "comes before" vehicle o's release year
x == 0 if this vehicle's release year is the same as the other vehicle o's release year
x > 0 if this vehicle's release year "comes after" vehicle o's release year
Hint: for any two Year reference variables a and b, one can test if year a comes "before" or "after" year b by saying a.isBefore(b) and a.isAfter(b), respectively (and you can test for exact equality by saying a.equals(b)).
Part 4: Garage Class and a Tester
Now write a class called Garage. The garage class should store a single private field, vehicles, of declared-type ArrayList
an addVehicle method that takes a Vehicle as a parameter, and adds it to the vehicles array list (the method returns nothing)
an emptyGarage() method that takes nothing and returns nothing, but clears out the internal vehicles array list
don't set vehicles = null here, call an array list method instead
a sortByReleaseYear() method that sorts all vehicle objects in the garage (you can call Collections.sort(vehicles) to accomplish this)
note: we can do this because we made Vehicle implement Comparable
override toString() such that it returns each vehicle stored in the vehicles list on a separate line
After this, create a Tester class that contains a main() method. Inside the main, create an instances of the following vehicles:
an F150 Truck whose model production years range from 2020-2022
a Honda Civic LX with production years from 1996-1998
a BMW M3 Limited (production years: 2015-2018)
a Toyota Tundra truck that is a dually (production years: 1987-1988).
Now instantiate a Garage object named g and add the created vehicles one at a time to g (using the garage's addVehicle method).
After the vehicles are all added to the garage, print g using system.out and then call g.sortByReleaseYear(); then print g again to see the sorted vehicles list.
For example, given this code in my main():
System.out.println("Before sorting: "); System.out.println(g); g.sortGarage(); // g is a garage object containing all 4 vehicles System.out.println("After sorting: "); System.out.println(g);
Here is what was output:
Before sorting: (Ford, USA) F150 in production = true, release year: 2020, mpg: 20.00 is dually truck: false (Honda, Japan) Civic in production = false, release year: 1996, mpg: 28.00 (BMW, Germany) M3 Limited in production = false, release year: 2015, mpg: 30.00 (Toyota, Japan) Tundra in production = false, release year: 1987, mpg: 30.00 is dually truck: true After sorting: (Toyota, Japan) Tundra in production = false, release year: 1987, mpg: 30.00 is dually truck: true (Honda, Japan) Civic in production = false, release year: 1996, mpg: 28.00 (BMW, Germany) M3 Limited in production = false, release year: 2015, mpg: 30.00 (Ford, USA) F150 in production = true, release year: 2020, mpg: 20.00 is dually truck: false
Step by Step Solution
There are 3 Steps involved in it
Step: 1
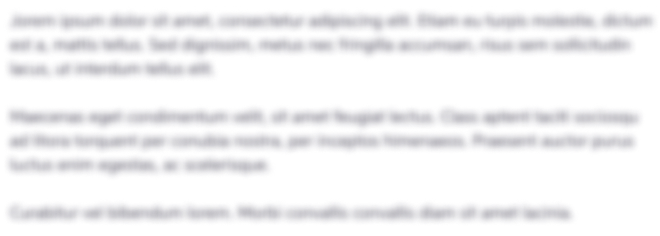
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started