Question
Part 1 Build an Account class. The Account class should have 3 Properties: balance, owner and acctNo. The Account class should have 2 constructors, one
Part 1
Build an Account class. The Account class should have 3 Properties: balance, owner and acctNo. The Account class should have 2 constructors, one that takes all 3 properties and one that takes no data (the default constructor). The Account class should have set and get methods as well as deposit and withdraw methods. Note that the Account class has no main() method.
Part 2
Create tester class called AccountTester to test out this class. The main() method will create an instance of the Account class and call its methods to test it. In particular the following tests should be done:
a) Create an Account with an initial balance of 200 using the multi-arg constructor. Use the widthdraw() method to withdraw 100 then display the balance.
b) Using the same Account object, call the deposit() method and deposit 50 and then display the balance.
c) Using the same Account object, use the withdraw() method to withdraw 500 and display the balance.
d) Using the setBalance() method on the Account object and set the balance to -100, then display the balance. What happens in your withdraw test if the balance goes below 0? Or if the setBalance() method is passed a negative number? In both cases the balance goes negative. Well, that is really an error, we don't want that. So we will need these methods to throw exceptions instead of creating a negative balance.
Part 3 Build an InsufficientFundsException class. Have this class extend from the Exception class. Also modify your Account class. Make it so that when the withdraw() method or the setBalance() method attempts to set the balance below zero, the InsufficientFundsException will be thrown. The AccountTester will, of course, also have to be changed to use a try/catch block anytime it calls the withdraw() or setBalance() methods catching the InsufficientFundsException and print out an appropriate message. Now rerun the AccountTester. What happens now?
Test Run
Here is what the output from running the AccountTester should look like after completing part 2.
Account object created with balance of 200
Withdrawing 100.
Balance after withdrawal of 100 is: 100
Depositing 50 Balance after deposit of 50 is: 150
Withdrawing 500
Error: Overdrawn
Balance is: 150
Setting Balance to -100
Error: Overdrawn
Balance is: 150
Step by Step Solution
There are 3 Steps involved in it
Step: 1
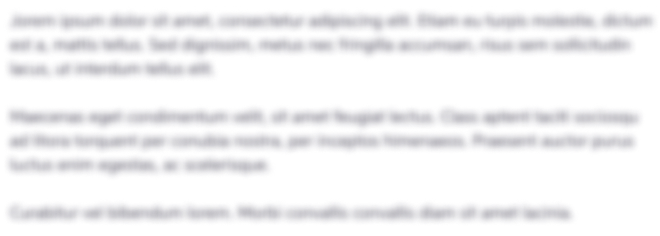
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started