Question
Part 1 Create and use a stack ADT (Abstract Data Type) to be used with a simple calculator. The calculator will be capable of evaluating
Part 1
Create and use a stack ADT (Abstract Data Type) to be used with a simple calculator.
The calculator will be capable of evaluating the following simple expression:
2.3 * 1.2 + 14.0
The operators for the simple calculator are as followed:
Addition +
Subtraction -
Multiplication *
Division /
This task involves three classes but just focus on the stack class please since I have to submit this question in two separate parts: Stack, Calculator, Driver. The driver class is complete and should not be modified. The other two classes are provided in incomplete format so they should be modified. You must fill in the details to make the class complete and for the driver to process correctly.
For the Stack class, you will need to complete:
top()
pop()
push()
isEmpty()
size()
Only the bodies of these 5 methods need to be completed.
Only include Stack.java work & down low is a driver class for reference if needed
Driver.java file
import java.io.BufferedReader;
import java.io.IOException; import java.io.InputStreamReader; public class Driver { public static void main(String[] args) throws IOException { // For example, entering: 2.3 * 1.2 + 14.0 - 100.5 // Displays: The result of 2.3 * 1.2 + 14.0 - 100.5 is -83.74000000000001 Calculator calc = new Calculator(); BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); System.out.print("Enter an expression: "); String str = br.readLine(); System.out.print("The result of " + str + " is " + calc.evalExp(str)); } }
Stack.java file
public class Stack
// Instance variables E stack[]; int top; int capacity; private final static int DEFAULT_SIZE = 30; // Constructors public Stack () { this(DEFAULT_SIZE); } public Stack (int capacity) { stack = (E[]) new Object[capacity]; this.top = -1; this.capacity = capacity; } // Other methods // Complete these methods for the assignment /** * Pushes an object on to the stack ** @param obj the object to be pushed */ public void push (E obj) { // Begin: Insert your code here to complete the method // End: Of your code } /** * Removes the top element on the stack and returns it to the caller *
* @return The top element of the stack, null if the stack is empty */ public E pop() { // Begin: Insert your code here to complete the method // End: Of your code } /** * Returns the top element on the stack to the caller *
* @return The top element of the stack, null if the stack is empty */ public E top() { // Begin: Insert your code here to complete the method // End: Of your code } /** * Returns true if the stack is empty *
* @return true if the stack is empty, otherwise false */ public boolean isEmpty() { // Begin: Insert your code here to complete the method // End: Of your code } /** * Returns the number of elements currently on the stack *
* @return number of elements */ public int size() { // Begin: Insert your code here to complete the method // End: Of your code } }
If this is still too much please explain why in a comment because this is my 5th time reposting this question and I need help on this problem before my final grade is submitted.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
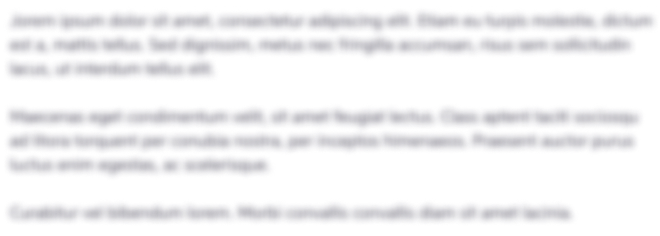
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started