Question
Part 1: Create Spinner.java to simulate a board game spinner. Each Spinner has a color and a number of sections. The spinner has an equal
Part 1:
Create Spinner.java to simulate a board game spinner. Each Spinner has a color and a number of sections. The spinner has an equal likelihood of landing in any of the sections.
The object should contain the following:
~ Data fields:
*Color (String)
*Number of Sections (int)
~Constructors:
*A default constructor. Have it set the color to red and the number of sections to 4.
*A custom constructor. Have it take 2 arguments, a String for the color and an int for the number of sections
~Methods:
*Getters:
public String getColor()
public int getNum()
*Setters:
public void setColor(String s)
public void setNum(int n)
*Method that simulates spinning the spinner. It returns a random number of one of the sections
public int spin()
Part 2:
import java.util.*; class SpinnerGame { public static void main(String[] args) { Scanner input = new Scanner(System.in); int board, posBlue =0, posPink=0; Spinner blue = new Spinner("blue",5); Spinner pink = new Spinner("pink", 3); System.out.println("How many spaces is the board?"); board = input.nextInt(); while(posBlue < board && posPink= board) System.out.println("Blue is the winner!"); else if(posPink >= board) System.out.println("Pink is the winner!"); } } }
Currently there are two Spinner objects in the game, a blue one and a pink one. Add another Spinner to the game. The new Spinner should be Green and have 4 spaces. All three Spinners then compete to see which one will get to the end of the board first.
For the first part, I have the following code:
public class Spinner{
//instance variable
private String color;
private int sections;
//default constructor
public Spinner(){
color = "red";
sections = 4;
}
//custom constructor
public Spinner (String c, int s){
color = c;
sections = s;
}
//setter
public void setColor (String c)
{
public void setSections (int s);}
//getter
public String getColor(){
return color;
}
public int getSections(){
return sections;
}
public String toString(){
return color + "," + sections + "sections"
}
//start spinning
public void set();
public int spin();
}
I then created a new file:
public class Spin{ public static void main(String[]){ Spinner c1 = new color(); System.out.println(c1); Spinner c2) = new color ("Green", 4); System.out.println(c2); s.1num(1); System.out.println(s1.getSections()); } }
I am certain there are errors in my code, and I would appreciate some fixes and explanations.
As for part 2, can someone guide me as to how to do this problem.
**I am coding in JAVA using repl.it.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
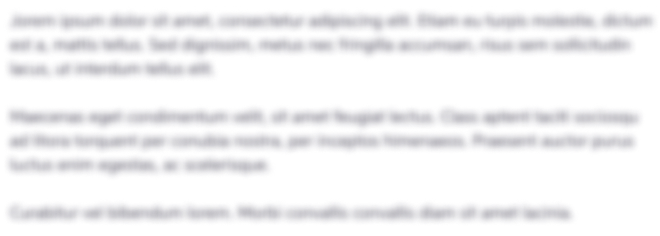
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started