Question
Part 1: Creating a JavaFX program (Lab2 1) In IntelliJ create a new project called Lab21. Under src create a package called lab21. In package
Part 1: Creating a JavaFX program (Lab2
1)
In IntelliJ create a new project called Lab21. Under src create a package called lab21. In package lab21 create a class called Lab21. In Lab21.java write a JavaFX program that displays a text field, a button, and a label. It is your choice where you would like to place them in the window, just not one on top the other. For example, you may create a FlowPane and add a TextField, Button and Label object to it. Set the caption on the button to say Submit. Initially, the text on the label should be blank. When the button is pressed set the labels text to the text in the text field. You can get and set text with methods getText() and setText(String value) of class TextField and Label respectively. TextField, Button and Label are in package javafx.scene.control.
Panes are in package javafx.scene.layout.
EventHandler and ActionEvent are in package javafx.event.
Padding is the area around a regions content. If you would like to add padding to a pane you will need to use class Inset. Inset is in package javafx.geometry. For example, if we have a pane called p, we can set its padding on all four sides to 10 pixels by doing p.setPadding(new Insets(10)); To create a Scene for pane p we can do: Scene scene = new Scene(p);
The title bar of stage (the main window) can be set by doing: stage.setTitle("Lab21");
To display scene on object stage we can do: stage.setScene(scene);
Finally, to show stage we need to do: stage.show();
Part 2: Tail Recursion for Fibonacci Numbers (Lab22)
Recall, a recursive method is tail recursive if the recursive call is the last operation performed in the method.The nth Fibonacci number fibo(n) is defined as follows:
fibo(0) = 0
fibo(1) = 1
fibo(n) = fibo(n-2) + fibo(n-1) for all integers n>1.
For negative values of n we will set fibo(n) = 0.
In this part we will write a tail recursive method for computing the nth
Fibonacci number.
Lets first look at a non-tail recursive solution to this problem:
public class Lab22
{
public static long fibo(int n)
{
if (n <= 0) return 0;
if (n == 1) return 1;
return fibo(n-2) + fibo(n-1);
}
}
This solution is inefficient in terms of both memory and time. We will convert this to a tail recursive version, which
will be efficient in both memory and time.
To convert this to tail recursion, create auxiliary parameters a and b for storing the old values of fibo(n-2) and
fibo(n-1) respectively. Think about what data type a and b should be. Hint: what is the return type of the method.
Rename the parameter n to i (this is done for clarity). The parameter i will count down from n by 1 until it reaches 0 (or less). When this happens, the final result will be stored in a. So our base case is when n0, in which case we return a.
Next, modify the method so that it makes only one recursive call. This recursive call will be the last action
performed in the method. Return its computed value.
The new values of i, a and b will be passed to the recursive call.
The new value of i is one less than the old value of i.
The new value of a is the old value of b.
The new value of b is the old value of a plus the old value of b.
Do not change the value of i, a or b. Instead, compute and pass their new values to the recursive call.
Notice how addition (which was done after the recursive call in the non-tail recursive solution) is now incorporated into the new value of b. This is performed before making the recursive call. The class user should not know about the auxiliary parameters a and b. To encapsulate this, make our method fibo(which now takes 3 arguments) private. Then overload fibo by creating the following public helper method for the class user to call:
public static long fibo(int n)
{
return fibo(n, 0, 1);
}
Here the parameter i is initialized to n, a is initialized to 0, and b is initialized to 1.
Finally, create a main method. In main prompt the user for the number n, then call fibo(n) and display its result to the screen.
Write a Java program that computes the nth Catalan number using a tail recursive method similar to part 2.
Name your project Lab23
Step by Step Solution
There are 3 Steps involved in it
Step: 1
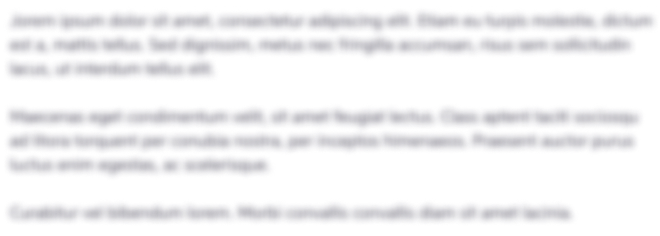
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started