Question
Part 1: Finishing the Card class The Card class has public static int data fields for all possible card ranks and suits. DO NOT CHANGE
Part 1: Finishing the Card class
The Card class has public static int data fields for all possible card ranks and suits. DO NOT CHANGE THESE.
In the Card class do the following:
1. Add private data fields for the cards rank, suit, and a boolean that represent if the card is face up or not (true = face up, false = face down).
2. Finish writing the constructor. Initialize the data fields to their corresponding parameters. 1 st parameter: the cards rank. 2 nd parameter: the cards suit. 3 rd parameter: a boolean indicating if the card is initially face up or not (true = face up, false = face down). If the constructors rank parameter is invalid (i.e., less than ACE or greater than KING), then set the cards rank to ACE. If the constructors suit parameter is invalid (i.e., less than SPADES or greater than CLUBS), then set the cards suit to SPADES. Use the constants ACE, KING, SPADES and CLUBS instead of writing their numeric values directly in code (e.g., write ACE instead of 1).
3. Finish writing the getRank() method to return the cards rank.
4. Finish writing the getSuit() method to return the cards suit.
5. Finish writing the isFaceUp() method. This method returns the value that indicates if the card is face up or not (true = face up, false = face down).
6. Finish writing the setFaceUp(boolean newValue) method. Passing true to this method sets the card face up. Passing false to this method sets the card face down. Do not add any more members to the public interface of class Card.
public class Card { // the different kinds of ranks public static final int ACE = 1; public static final int TWO = 2; public static final int THREE = 3; public static final int FOUR = 4; public static final int FIVE = 5; public static final int SIX = 6; public static final int SEVEN = 7; public static final int EIGHT = 8; public static final int NINE = 9; public static final int TEN = 10; public static final int JACK = 11; public static final int QUEEN = 12; public static final int KING = 13; // the different kinds of suits public static final int SPADES = 0; public static final int HEARTS = 1; public static final int DIAMONDS = 2; public static final int CLUBS = 3; // TODO: add any needed private members here. public Card(int theRank, int theSuit, boolean isTheCardFaceUp) { // TODO: write constructor here. } public int getRank() { // TODO: write method body here. return ACE; // Temporary return value. Replace this line. } public int getSuit() { // TODO: write method body here. return SPADES; // Temporary return value. Replace this line. } public boolean isFaceUp() { // TODO: write method body here. return false; // Temporary return value. Replace this line. } public void setFaceUp(boolean newValue) { // TODO: write method body here. } }
Part 2: Shuffling the deck of cards
In the TwentyOneGame class the deck of cards are stored in an array called deck. Locate the shuffleDeck() method in class TwentyOne. In here write code to randomly shuffle the array deck using the algorithm described below. Do not use any of the Java library utilities for shuffling the deck. To shuffle deck, loop over deck backwards starting at the last element and going down to the second element. In each loop iteration swap the current element (i.e., deck[i] where i is the loop counter) with a random element to the left of or at the current position. For example, if the current position is 5, then we would randomly select an index j from 0 to 5 (inclusive) and then swap the card at position 5 with the one at position j. To generate a random integer between 0 (inclusive) and n (exclusive), call generator.nextInt(n)
package twentyone; import java.util.ArrayList; import java.util.Random; public class TwentyOneGame { public static final int TARGET_SCORE = 21; private final Card[] deck; // The deck of cards. private int topOfDeck; // Index to top of deck. // The bottom of the deck has index 0. private boolean isGameOver; // Indicates if the game is over. private final ArrayList houseCards; // List of house's cards. private final ArrayList playerCards; // List of player's cards. private final Random generator; // Used for generating random numbers. public TwentyOneGame() { deck = new Card[Card.KING * (Card.CLUBS + 1)]; generator = new Random(); houseCards = new ArrayList<>(); playerCards = new ArrayList<>(); newGame(); } // seed the internal random number generator before creating a new game public void newGame(long seed) { generator.setSeed(seed); newGame(); } public void newGame() { topOfDeck = deck.length - 1; int i = 0; for (int suit = Card.SPADES; suit <= Card.CLUBS; ++suit) { for (int rank = Card.ACE; rank <= Card.KING; ++rank) { deck[i++] = new Card(rank, suit, false); } } shuffleDeck(); // Remove all cards from the house and the player. houseCards.clear(); playerCards.clear(); // deal the cards out to the player and the house. hit(); houseHit(false); hit(); houseHit(true); isGameOver = false; } public boolean isOver() { return isGameOver; } public final ArrayList getHouseCards() { return houseCards; } public final ArrayList getPlayerCards() { return playerCards; } private void shuffleDeck() { // TODO: Shuffle the array deck using the algorithm // described in the instructions. Do NOT use any of // the Java library utilities to do the shuffling. } // Player takes a card from the deck. // Returns the new card from the deck or null if either // the deck is empty or the game is over. public Card hit() { if (isGameOver || topOfDeck < 0) return null; Card newCard = deck[topOfDeck--]; newCard.setFaceUp(true); playerCards.add(newCard); if (getPlayerScore() > TARGET_SCORE) { isGameOver = true; houseCards.get(0).setFaceUp(true); } return newCard; } // House takes a card from the deck. // If parameter faceCardUp is true, then the card will be placed face up, // otherwise it will be placed face down. // Returns the new card from the deck or null if the deck is empty. private Card houseHit(boolean faceCardUp) { if (topOfDeck < 0) return null; Card newCard = deck[topOfDeck--]; newCard.setFaceUp(faceCardUp); houseCards.add(newCard); return newCard; } private void housePlay() { // Turn house's face down card up. houseCards.get(0).setFaceUp(true); // The house takes a card until his score is 17 or greater. while (getHouseScore() < 17) { houseHit(true); } isGameOver = true; // The game is now over. } public void stand() { if (!isGameOver) housePlay(); } private static int getScore(ArrayList hand) { // TODO: Compute the total score of the cards in the given hand. // A card that is face down does not add to the score. // A face card (Jack, Queen, or King) is worth 10 points. // A numeric card (2 through 10) is worth the value on the card. // An Ace is worth either 11 points or 1 point. It is worth 11 points // if this does not make the total score go over 21, otherwise it is // worth 1 point. // The returned score is the sum of all the values of the cards in // the ArrayList hand. // Do NOT use the magic number 21 in your code. Instead, // use the class constant TARGET_SCORE for 21. return TARGET_SCORE; // Temporary return value. Replace this line. } public int getHouseScore() { return getScore(houseCards); } public int getPlayerScore() { return getScore(playerCards); } // Returns false if the player loses or the game is not over // otherwise return true. public boolean playerWins() { int houseScore = getHouseScore(); int playerScore = getPlayerScore(); return isGameOver && playerScore <= TARGET_SCORE && (playerScore > houseScore || houseScore > TARGET_SCORE); } }
Part 3: Computing the score
Your task in this part is to compute the score of a given players hand. Locate the getScore(ArrayList hand) method in class TwentyOneGame. Its parameter hand is an ArrayList of all the cards in someones hand. Write the body of this method to compute and return the total score of all the cards in the ArrayList hand. Value of cards: A card that is face down does not add to the total score. A face card (Jack, Queen, or King) is worth 10 points. A numeric card (2 through 10) is worth the value on the card. An Ace is worth either 11 points or 1 point. It is worth 11 points if it does not make the total score go over 21. Otherwise it is worth 1 point. Example: If the hand is {A, 5, 8}, then A is worth 1 point since 11 + 5 + 8 = 24 > 21. But if the hand is {A, A}, then one of the Aces is worth 11 points and the other is worth 1 point. Do not write the magic number 21 directly in your code. Instead, use the class constant TARGET SCORE for 21.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
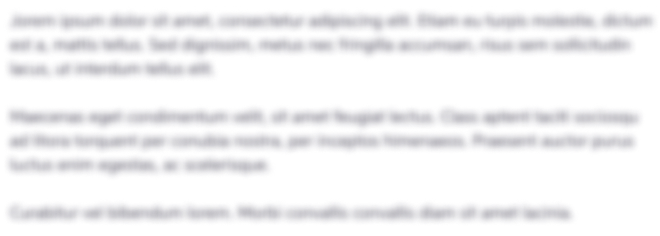
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started