Question
PART 1: GETTING STARTED Create a new Java Project for this lab assignment. Create a new class. Name it Lab9. Add this code to the
PART 1: GETTING STARTED
Create a new Java Project for this lab assignment.
Create a new class. Name it Lab9.
Add this code to the Lab9 class.
/*
* Adapted from Kjell, slide 110_10
*/
public class Lab9
{
// Sort the Array
//
public static void main ( String[] args )
{
int[] values = { 17, 5, 21, 8, 19, 2, 23, 15, 4, 13 };
int numValues = 10;
// print out the array
System.out.println("initial values: ");
for ( int i=0; i < numValues; i++ )
System.out.println( values[i]);
// sort the array
SelectionSortFilm.sortIt( values, numValues );
// print out the array
System.out.println(" sorted values: ");
for ( int i=0; i < numValues; i++ )
System.out.println( values[i]);
System.out.println( );
}
}
Create a new class. Name it SelectionSortFilm.
Add this code to the SelectionSortFilm class.
public class SelectionSortFilm {
public static void sortIt( int[] array,
int numberOfThingsToSort )
{
/* Find the integer that should go in
* each cell j of the array, from cell 0 to the end
*/
for ( int j=0; j { /* Find min: the index of the integer * that should go into cell j. * Look through the unsorted integers * (those at j or higher) */ int min = j; for ( int k=j+1; k< numberOfThingsToSort; k++ ) if ( array[k] < array[min] ) min = k; // Swap the int at j with the int at min int temp = array[j]; array[j] = array[min]; array[min] = temp; } } } Run the program to make sure it correctly sorts the array of 10 integers before continuing. List the numbers printed after the array of 10 integers is sorted: Create a third class. Name it FilmTake2. Page Break Add this code to the FilmTake2 class. /* * The Film class contains data about a film. * * Methods: * Film() - no arguments constructor * Film(String, int) - constructor with two arguments * String toString() - convert data members to String * boolean equals(Film) - compare two Film objects for equality */ public class FilmTake2 { private String title;// Title of film private int year;// Year film was released // No arguments constructor initializes data members to // null or zero public FilmTake2() { title = ""; year = 0; } // Constructor initializes data members public FilmTake2(String filmTitle, int filmYear ) { title = new String(filmTitle); year = filmYear; } // toString() converts data members of object to String public String toString() { return title + " " + year; } // equals() compares two Films for equality public boolean equals (FilmTake2 f2){ if (this.title.equals(f2.title)) return true; else return false; } } PART 2: COMPARING TWO OBJECTS Add this compareTo() method to the FilmTake2 class. /* compareTo(FilmTake2) * * Sample call: film1.compareTo (film2) * * compareTo() compares the titles of two films and returns a * value based on their alphabetical order * * compareTo() returns * -1 if film1 < film2 * 0 if film1 == film2 * +1 if film2 > film2 * */ public int compareTo(FilmTake2 f2) { return (this.title.compareToIgnoreCase(f2.title)); } Thought experiment: What value would compareTo() return from these sample calls? FilmTake2 f1 = new FilmTake2("Venom", 2018); FilmTake2 f2 = new FilmTake2("Halloween", 2018); f1.compareTo(f2); f2.compareTo(f1); f1.compareTo(f1); PART 3: SORTING OBJECTS Make these highlighted changes to the SelectionSortFilm class. public class SelectionSortFilm { public static void sortIt( FilmTake2[] array, int numberOfThingsToSort ) { /* Find the integer that should go in * each cell j of the array, from cell 0 to the end */ for ( int j=0; j { /* Find min: the index of the integer * that should go into cell j. * Look through the unsorted integers * (those at j or higher) */ int min = j; for ( int k=j+1; k< numberOfThingsToSort; k++ ) if ( array[k].compareTo(array[min]) < 0) min = k; // Swap the int at j with the int at min FilmTake2 temp = array[j]; array[j] = array[min]; array[min] = temp; } } } Make these changes to the Lab9 class. Change the type of the values array so it holds items of type FilmTake2. Instantiate the array. It should hold up to 20 items. Add at least 10 films to the array starting with this one: values[numValues++] = new FilmTake2("Venom", 2018); Now run the program and see if your program sorts the films into alphabetical order. What is the difference between a static method and a method that is not static?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
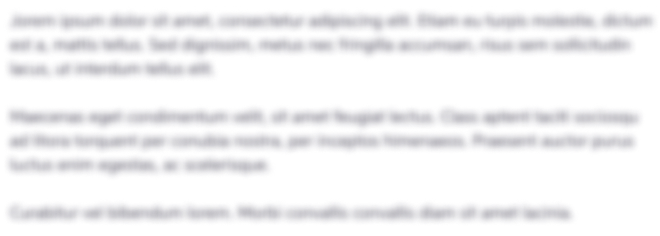
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started